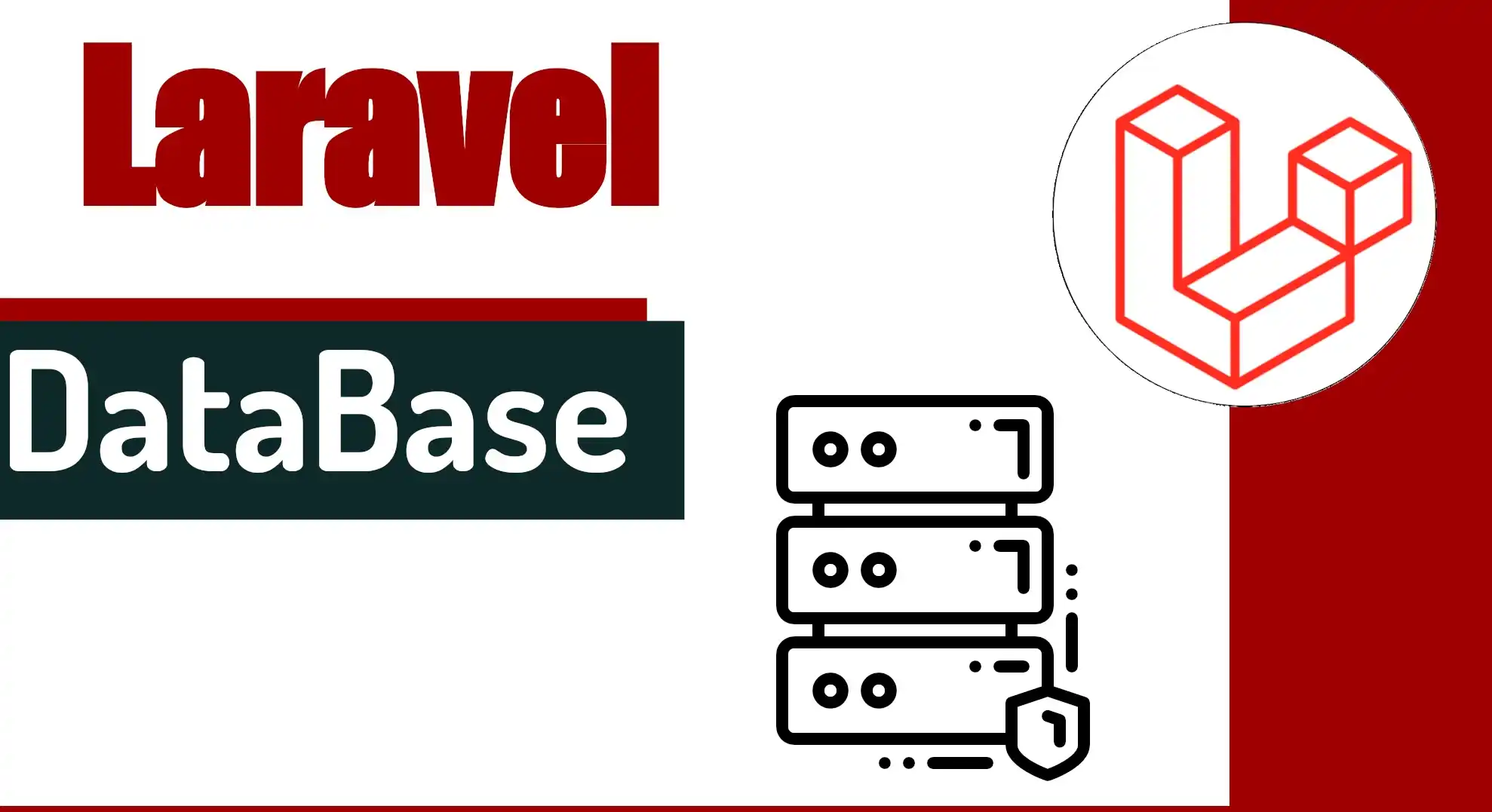
Depending on the type of application we want to build, it is often necessary to perform multiple operations on the database safely, for example, a purchase of an article from a book that we must do steps such as the following:
- Register the payment identifier in the database.
- Discount inventory quantities.
- Register the product in the customer's shopping list.
These are some possible steps, but there may be more and they can be in any order. There are many other cases in which it is necessary to do several operations on the database and if one of these fails, then all the previous operations should be done. reverted to avoid leaving the process in an intermediate state; in the previous example, we could say that if I reach step two and for some reason the product is no longer in inventory, the previous operation would give an error and I would not be able to record step three as it could not discount the product from inventory, leaving the order in limbo since step one would have been completed.
And this is where the use of transactional operations comes in, allowing a set of queries to be grouped into a single unit of work, ensuring that all of them are executed correctly or none of them are. In other words, if a query fails, all modifications previously made to the database are reverted. In our previous example, if there is a problem in any of the steps, Laravel reverts all operations, with this, we guarantee the integrity of the data, preventing the order from being left in limbo and atomicity of the operations, since all operations they are carried out in the same work unit and if any of them fails, all the operations are reversed and with this we fulfill the main objective of making the application safe from possible problems as we mentioned before.
Aquí tenemos un ejemplo de su uso:
use Illuminate\Support\Facades\DB;
try {
// start the transaction
DB::beginTransaction();
// Realiza tus consultas aquí
DB::table('users')->insert(['name' => 'John Doe']);
DB::table('orders')->insert(['user_id' => 1, 'total_amount' => 100]);
DB::commit(); // Commit changes to the database
} catch (\Exception $e) {
DB::rollback(); // Revert changes in case of error
return $e->getMessage();
}
In this example:
DB::beginTransaction() initializes the transaction. Within the try block, we perform all operations on the database using our models or similar.
- If all the operations we wanted to perform were resolved successfully, we called the DB::commit() method to commit the changes.
- If an error occurs, the catch block calls DB::rollback() to rollback any changes that may have been made.
It can also be implemented using a callback:
DB::transaction(function () {
// Make your queries here
DB::table('users')->insert(['name' => 'John Doe']);
DB::table('orders')->insert(['user_id' => 1, 'total_amount' => 100]);
DB::commit();
});
I agree to receive announcements of interest about this Blog.
We will learn how to use Transactional Operations in the database with Laravel so that we can perform several operations on the same task unit in Laravel.
- Andrés Cruz
This material is part of my complete course and book; You can purchase them from the books and/or courses section, Curso y Libro Laravel 12 con Tailwind Vue 3, introducción a Jetstream Livewire e Inerta desde cero - 2025.