Routes are a flexible scheme that we have to link a URI to a functional process; and this functional process can be:
- A callback, which is a local function defined on the same routes.
- A controller, which is a separate class.
- A component, which is like a controller, but more flexible.
If we check in the routes folder; we will see that there are 4 files:
- api: To define routes of our Apis Rest.
- channels: For fullduplex communication with channels.
- console: To create commands with artisan.
- web: The routes for the web application.
The one we are interested in in this chapter is the web.php; which allows us to define the routes of our web application (those that our client consumes from the browser).
The routes in Laravel are a central element that allow link controllers, and to be able to programmer our own processes; that is, the routes do not need the controllers to be able to present a content; and therefore, it is the first approach that we are going to present.
If you notice, we have a route already defined:
Route::get('/', function () {
return view('welcome');
});
Which, as you can imagine, is what we see on the screen just when we start the application like figure 2-1.
Note that a class called Route is used, which is imported from:
use Illuminate\Support\Facades\Route;
Which is internal to Laravel and are known as Facades.
The Facades are nothing more than classes that allow us to access the framework’s own services through static classes.
Finally, with this class, we use a method called get(); for routes we have different methods, as many methods as type of requests we have:
- POST create a resource with the post() method.
- GET read a resource or collection with the get() method.
- PUT update a resource with the put() method.
- PATCH update a resource with the patch() method.
- DELETE delete a resource with the delete() method.
In this case, we use a route of type get(), which leads to using a request of type GET.
The get() method, like the rest of the methods noted above, takes two parameters:
Route::<ResourceFunction>(URI, callback)
- Application URI.
- The callback is the controller function, which in this case is a function, but it can be the reference to the function of a controller or a component.
And where "ResourceFunction" is the get(), post(), put(), patch() o delete() method.
In the example above, the ”/” indicates that you are the root of the application, which is:
http://larafirststeps.test/
Or localhost if you use MacOS or Linux using Docker.
In this case, the functional part is an anonymous function; this function can do anything, return a JSON, an HTML, a document, send an email and much more.
In this example, it returns a view; to return views, the helper function called view() is used, which references the views that exist in the folder:
resources/views/<View and Foldes>
By default, there is only a single file; the one called welcome.blade.php, and yes, it’s the one we’re revering in the path above with:
return view('welcome');
Note that it is not necessary to indicate the path, nor the blade or php extension.
Blade refers to Laravel’s template engine which we’ll talk about a bit later.
If you check the view of welcome.blade.php:
You’ll see all the HTML of it:
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laravel</title>
***
In which we see something like figure 2-1 in the browser.
So, if we create a few more routes:
Route::get('/writeme', function () {
return "Contact";
});
Route::get('/contact', function () {
return "Enjoy my web";
});
And we go to each of these pages, respectively:
And
Route::get('/custom', function () {
$msj2 = "Msj from server *-*";
$data = ['msj2' => $msj2, "age" => 15];
return view('custom', $data);
});
views/cursom.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<p>{{ $msj2 }}</p>
<p>{{ $age }}</p>
</body>
</html>
Named routes
Another configuration that cannot be missing in your application is the use of named routes; as its name indicates, it allows you to define a name for a route.
***
Route::get('/contact', function () {
return "Enjoy my web";
})->name('welcome');
For that, a function called name() is used, to which the name is indicated; to use it in the view:
<a href="{{ name('welcome') }}">Welcome</a>
This is particularly useful, since you can change the URI of the route, group it or apply any other configuration to it, but as long as you have the name defined in the route and use this name to reference it, Laravel will update it automatically.
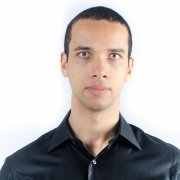
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter