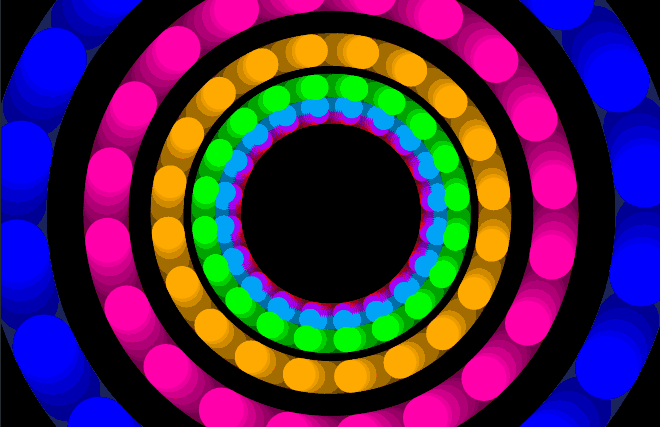
In this post we will see how to create a simple system of circle rings like the following:
Again we will make use of several mathematical operations (among them the sine and cosine functions to determine the X and Y position of the circles given the angle) and again the requestAnimationFrame() function introduced in a previous installment called: THE SECRET OF JAVASCRIPT ANIMATIONS , the Canvas element, and a bit of JavaScript keeping the HTML to a minimum just like in the previous posts:
- ¿CÓMO CREAR UN EFECTO DE ONDA CON CANVAS Y JAVASCRIPT?
- ¿CÓMO CREAR UN PUNTO LUMINOSO CON JAVASCRIPT Y CANVAS?
- CREANDO PARTÍCULAS CON JAVASCRIPT Y CANVAS
Building our circle system with Canvas and JavaScript
As in other posts, we first show the entirety of the code used:
var c = document.getElementById('canv');
var w = c.width = window.innerWidth;
var h = c.height = window.innerHeight;
var $ = c.getContext('2d');
var v = 0; // velocidad
var dst = 100; // cercania
var rings = 10; // numero de anillos de circulos
var circs = 20; // numero de circulos
var dosPi = Math.PI * 2; // dos veces PI
window.addEventListener('resize', function () {
c.width = window.innerWidth;
c.height = window.innerHeight;
}, false);
function draw(ang) {
var col = -80;
for (var u = 0; u < rings; u++) {
sd = Math.pow(u, 3) + dst; // distancia entre los anillos de circulos
x = w / 2 + Math.cos(ang) * sd;
y = h / 2 + Math.sin(ang) * sd;
s = sd / 10;
$.fillStyle = 'hsla(' + col * (u) + ',100%, 50%, 1)';
$.beginPath();
$.arc(x, y, s, 0, dosPi);
$.fill();
}
}
function go() {
$.fillStyle = 'hsla(0,0%,0%,.08)';
$.fillRect(0, 0, w, h);
v += 0.02;
if (v >= 5)
v = 0;
for (var i = 0; i < circs; i++) {
ang = (v + (i / circs * dosPi));
draw(ang);
}
window.requestAnimationFrame(go);
}
go()
Parsing the above JavaScript code
Here again is a brief explanation of the most important sections of the above JavaScript.
Global variables
We declare the global variables for our experiment, those that allow access to the Canvas and its context:
var c = document.getElementById('canv');
var c = document.getElementById('canv');
var w = c.width = window.innerWidth;
var h = c.height = window.innerHeight;
var $ = c.getContext('2d');
var v = 0; // velocidad
var dst = 100; // cercania
var rings = 10; // numero de anillos de circulos
var circs = 20; // numero de circulos
var dosPi = Math.PI * 2; // dos veces PI
The variable dosPi is used to calculate the angles of the circle in radians; For now, do not worry too much about this, later I will give a link in which you can detail the previous information.
Calculating the angle and setting the animation
The first function to execute after loading the entire web page is the function called go(); this function is responsible for calculating the angles of the circles and thus being able to locate them within the ring:
function go() {
$.fillStyle = 'hsla(0,0%,0%,.08)';
$.fillRect(0, 0, w, h);
v += 0.02;
if (v >= 5)
v = 0;
for (var i = 0; i < circs; i++) {
ang = (v + (i / circs * dosPi));
draw(ang);
}
window.requestAnimationFrame(go);
}
In addition to this, we also animate the Canvas using the requestAnimationFrame function which recursively calls this go() function.
The go function also paints the characteristic transparent black box to create the halos of the circles.
The calculation:
ang = (v + (i / circs * dosPi));
It allows calculating the angle in radians of the unit Circumference, although this is a bit beyond the theme of this post, which is to show how to create rings of circles in JavaScript and Canvas; If you want to know more, check the previous link.
As you may have noticed, we are simply going around the circle -anillo- where the value of the variable dosPi (which is twice the value of PI, something like 6.282) represents a complete turn around the circumference.
In summary, we are calculating the angle to place the different circles in the shape of a ring by going through the angle from 0 to twice the value of PI.
It's like drawing circles within a circle (ring).
Drawing the circles
Once we have the angle we must apply the cosine and sine functions to obtain the position where we are going to place the circles that we use to draw the position of the small circles that form the ring in the draw() function:
x = w / 2 + Math.cos(ang) * sd;
y = h / 2 + Math.sin(ang) * sd;
The variable v is used to animate the rings of circles, the larger the incremental value of the variable, the greater the angle and therefore the greater the displacement of the circle and the faster the animation will be.
Once we have the angle at which to draw the circles we proceed to calculate the exact position and draw a circle in a ring at a time:
function draw(ang) {
var col = -80;
for (var u = 0; u < rings; u++) {
sd = Math.pow(u, 3) + dst; // distancia entre los anillos de circulos
x = w / 2 + Math.cos(ang) * sd;
y = h / 2 + Math.sin(ang) * sd;
s = sd / 10;
$.fillStyle = 'hsla(' + col * (u) + ',100%, 50%, 1)';
$.beginPath();
$.arc(x, y, s, 0, dosPi);
$.fill();
}
}
I agree to receive announcements of interest about this Blog.
In this post we will see how to create a simple circle ring system with Canvas and JavaScript; for this, the requestAnimationFrame() function will be used to animate the Canvas.
- Andrés Cruz