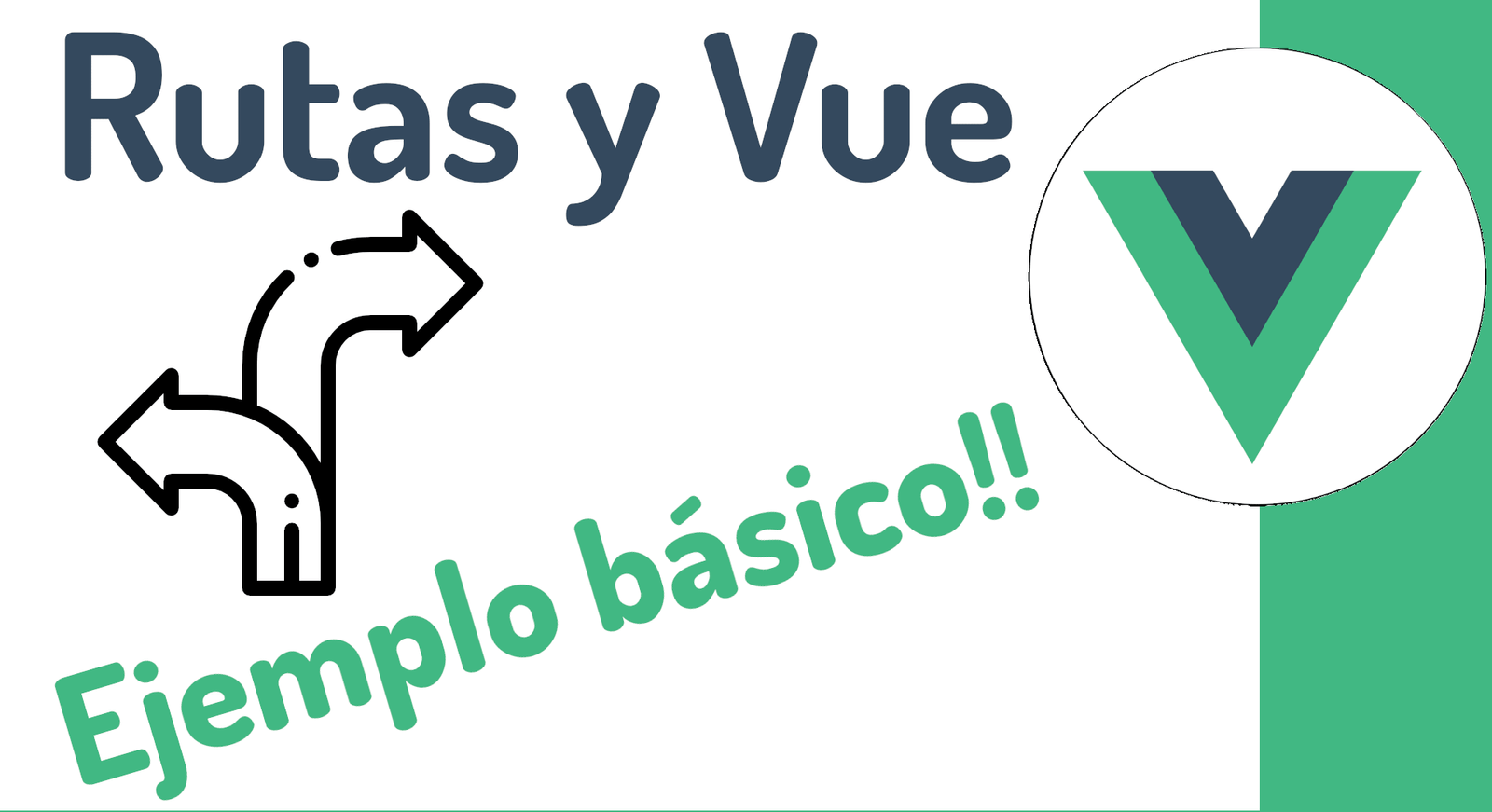
We are going to learn how to do a simple routing in Vue 3, for this we are going to use the Vue Router plugin which is excellent because it is developed and maintained by the same Vue people, therefore the integration is optimal.
About routing in Vue
Navigation is a fundamental issue for any type of application development that we are carrying out, and Vue or any other client-side JavaScript framework cannot be the exception.
Navigation through routes allows us to link different pages
Pages based on components, therefore we can easily scale the application with new pages and/or components.
You can see the components as if they were a micro app that performs a particular function, a list, a form, a functionality, etc., which, in turn, can be divided into components that are found in other components, but this is another matter..
Create an app in Vue CLI
As always, we are going to start by creating a new application in Vue, for that we are going to use the command line that Vue offers us, known as CLI, we are going to install it globally to our OS:
npm install -g @vue/cli
Or
yarn global add @vue/cli
Remember that you can use Windows, Linux or Mac since it is multiplatform.
Now, we happily create a new project in Vue 3:
vue create my-vue-router
Select Vue 3, the preview and with this we will have our basic and clean project in VUE 3 in no time:
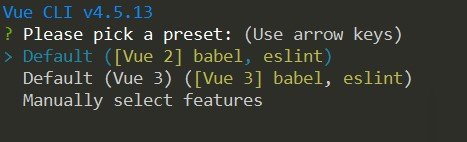
Vue Router Basic
Install Vue Router
On the folder of our project that we created previously, we execute:
npm i vue-router@next
Creating the file for the routes
As you can guess, just like the classic operation of any plugin, the linking of the plugin with Vue has to be done in the main instance, here (in the main instance file) you can place any amount of code that in the case of the routes would be the definition of the routes that look like this:
import { createRouter, createWebHistory } from 'vue-router'
import Login from '../components/Login';
import App from '../components/App';
import Group from '../components/Group';
import Chat from '../components/Chat';
const routes = [
{
path: '/',
name: 'App',
component: App
},
{
path: '/login',
name: 'Login',
component: Login
},
{
path: '/group',
name: 'Group',
component: Group
},
{
path: '/chat/:room',
name: 'Chat',
component: Chat
}
]
const router = createRouter({
// 4. createWebHashHistory
history: createWebHistory(),
routes, // short for `routes: routes`
})
export default router
But obviously defining all the routes that you are going to use; So, to keep this file simple, we're going to make this entire definition in a separate file that we'll then export and define directly within the main Vue instance.
Create and define the router file
Although you can name this file anything and place it in any location, I like to place them inside:
helpers/router.js
Here I am going to assume that we have some dummy components, although they are based on my Electron course in which we were building a Chat application with Firebase and you can get more information from this page in the course section.
Explanation of the above code
There is not much to say here, basically everything is self-explanatory, in the same way:
- The function called createRouter that is part of the Router Vue plugin allows us to define the routes, for this we have to pass a parameter that is the routes, an array with the routes, in which each element has to have the path, which It is the uri and the component, the name is optional and it is useful when we want to create a navigation link (router-link) or programmatically navigate through the router component.
- For the rest, we have to define the URL composition mode for the createRouter function:
- history: createWebHistory(),
Below this array, you'll notice that we created the router itself, using the routes, and also passed createWebHistory. This is used to switch from hashing to history mode within your browser, using the HTML5 History API. The way we set this up is a bit different than what we did in Vue 2 in the same course.
It is simply to indicate if we want a clean URL or a simulated one with a hash mark.
Defining the base template
In our src/App.vue, which is the component that is created by default and loaded by default, we place the skeleton of the app together with the router-view that allows us to create the SPA web; and where the components through which our user navigates and that correspond to the previously defined routes are rendered:
<template>
<div id="nav">
<router-link to="/">App</router-link> |
<router-link to="/login">Login</router-link> |
<router-link to="/group">Group</router-link>
</div>
<router-view />
</template>
<script>
export default {
name: "App",
components: {},
};
</script>
You can see each of the components that we use here in a demonstrative way in the github repository that I left you earlier.
Keywords:
- Route: The URL path where this route can be found.
- Name – An optional name to use when binding to this route.
- Component: Which component to load when this route is called.
- Using <router-view> and <router-link>
There are two directives that our Vue app gives us to use in our template:
<router-view />: when navigating to a route in the browser, this is where the component is rendered, ie where the changing content of our SPA type page is rendered. For example, in our code, going to / will render the Home component where we listed <router-view />.
<router-link> – This is the directive we use to create links between our different component pages, rather than using <a href> .
Why Vue Router?
There are two directives that our Vue app gives us to use in our template:
<router-view />: when navigating to a route in the browser, this is where the component is rendered, ie where the changing content of our SPA type page is rendered. For example, in our code, going to / will render the Home component where we listed <router-view />.
<router-link> – This is the directive we use to create links between our different component pages, rather than using <a href> .
I agree to receive announcements of interest about this Blog.
We are going to learn how to do a simple routing in Vue 3, for this we are going to use the Vue Router plugin which is excellent because it is developed and maintained by the same Vue people, therefore the integration is optimal.
- Andrés Cruz