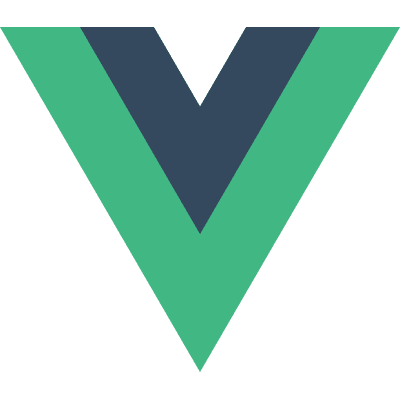
In this post we will see the first steps with this framework called Vue, which is the boom of today, the JavaScript framework that is more fashionable; we will take a quick look at Vue's features, where to install it, how to download Vue, we will do a simple comparison of Vue with jQuery and then we will give some small examples to start working with Vue.
What is Vue and what is it for?
Vue is a Progressive Framework, which means it's for consuming the user interface; It was created by Evan You who was working at Google at the time; Vue emerged in 2014, as you can see it is a recent framework with "little" time on the market but it is very polished, it is well received by users, a growing community, good documentation and ease of use that do not require not even use Node to use it, which is a great advantage it has over the competition.
Vue Features
Vue is a progressive framework, which means that we can use it for something very basic, just like jQuery or larger and more complex systems, and in general it has very good performance for how little it weighs:
- Vue is an accessible framework that is available to everyone through open source.
- It is a very small framework that greatly facilitates interaction with the interface or HTML.
- Optimized; its kernel is very small and occupies about 70Kb, which is quite light.
- As we indicated before, it has a growing community and good documentation with a low learning curve since it is easy to use and very concise.
How to install Vue?
As we have indicated in its characteristics, we have several ways to install Vue, if we want to install it to do simple things, without using components, we just need to use the script available on the CDN:
<script src="https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.17/vue.min.js"></script>
Now if we want to use it to do more complex things, use components, etc., we can use the Vue cli that allows us to add components, pages, and total control over our project from the command line that we will see in other posts.
To install Vue we go to the official site at:
From the web we will see that we can install it through an npm module, but we can also download it with any JavaScript file and copy it into our HTML file.
Vue has a tool called Vue Cli that allows us to install plugins and thus extend its functionality, we can easily create projects based on this technology, however the easiest way to start is by including the previous URL as we will do in this entrance; It will be left for more advanced entries to use the Vue Cli tool to integrate it with Laravel or Codeigniter, etc.
Vue vs jQuery
The first thing you have to keep in mind is that they are two worlds apart, two different paradigms that seek to do the same thing, web applications in a simple way, jQuery has a huge number of plugins and gives us that extra that we don't have with native JavaScript and Vue also gives us a significant number of plugins and allows us to gain organization, optimizing at the code level down to the minimum to do things that are general as we will see below.
There are many reasons why we can use frameworks like Vue that are progressive instead of jQuery or similar frameworks; Vue offers us a greater organization (although in practice jQuery does not offer us any organization or development pattern) than that offered by jQuery, they are codes that are easier to understand and maintain since it has components and the Vue template pattern that we saw in the previous section; A very strong point is, as we will see in other Vue entries, it is the powerful interaction based on events, we can establish models or variables in Vue and tie them to an HTML element and modify it wherever we want, that is, if we modify the variable from JavaScript, this change will be reflected in the variable; the same happens if we modify it in an HTML element.
We can reference variables in the HTML as if it were a template and it will be printed and updated in real time if the variable undergoes changes without us doing something.
Now it is up to you to imagine how to do this with native JavaScript or jQuery, which although it is not a complicated task, as you will see in the examples section in Vue, it is something native and works very well.
We can print arrays easily and from the HTML itself using some special clauses that vue itself processes internally, in the case of jQuery we would have to iterate everything through a for or each within the script block
In the end, one technology does not have to overlap the other, it all depends on the project you are carrying out and intelligently coupling one or the other technology, including both without any problem.
Some jQuery Pros
- Being able to make Ajax requests easily.
- A set of animations extensible through plugins.
- Thousands of plugins at your disposal.
Some cons of jQuery
- You must handle each HTML element independently.
- The rendering system (showing content in HTML) is typical and somewhat outdated compared to other technologies.
- It's a pain when the app grows as it lacks organization.
Some advantages of Vue
- Great system to render JavaScript to HTML as if it were a template.
- Low learning curve.
- Organization through components.
- Extensible through components.
- Vue Cli to create SPA applications.
Some disadvantages of Vue
- It does not make Ajax requests natively.
- It is an evolving technology, always changing.
Of course, jQuery has important plugins and methods that make life much easier for us, so depending on the focus of your project you can select one or the other or even both and use the strongest elements of each of them.
Why start using Vue?
As we mentioned before, we should not cling to one technology or another, each of them has its advantages and disadvantages, the same things can be done in different ways, so depending on what you want to do, select one technology or another; Vue allows us to save time when creating applications with which we are going to manipulate the HTML DOM a lot due to how it is conceived, but there are many other more specific tools that you can also use depending on what you want to do.
Vue Examples: Building Our First Applications
In this section we will see how to use the basics of Vue, we remind you that Vue as a framework that is, you can expand its functionality through plugins and it has its own command line system through Vue Clic, but we can do really interesting things with Vue using a minimal JavaScript compared to jQuery or even native JavaScript:
Declarative rendering
As a very powerful feature, Vue has its own rendering system in which in the HTML that we define the scope of Vue, we can render values of variables declared in JavaScript and referenced in HTML as we see below:
<div id="app">
<h1>{{message}}</h1>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
new Vue({
el: '#app',
data: {
message: "Hola Vue"
}
})
</script>
This gives us the following output:
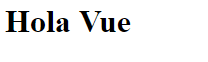
As you can see it is a very powerful feature of Vue, nowadays that we receive data from everywhere, we can easily reference variables, gaining readability and organization in JavaScript like never before.
You might think something like "Ok ok we can print variables in the HTML with some braces what else can it do".
Conditionals in Vue
Another very common characteristic is that we want to show a certain HTML block if something happens, or hide it, for this there are conditionals; for example for the following code:
<div id="app">
<h1 v-if="isActive">{{name}}</h1>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
let vm = new Vue({
el: '#app',
data: {
isActive:true,
name: 'Andres'
}
})
</script>
In this way, if we change the value of the isActive property to false then the h1 defined in the HTML would not be displayed; for the above example the output would be like the following:
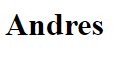
But we can put an else or better v-else clause like the following to show another message, code block or whatever you prefer:
<div id="app">
<h1 v-if="isActive">{{name}}</h1>
<h1 v-else-if="name === 'Andres'">Hola Andres como estas</h1>
<h1 v-else="isActive">No User</h1>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
let vm = new Vue({
el: '#app',
data: {
isActive:false,
name: 'Andres'
}
})
</script>
And we can even place the typical v-else-if to place more conditions as in the following case:
<div id="app">
<h1 v-if="isActive">{{name}}</h1>
<h1 v-else-if="name === 'Andres'">Hola Andres como estas</h1>
<h1 v-else="isActive">No User</h1>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
let vm = new Vue({
el: '#app',
data: {
isActive:false,
name: 'Andres'
}
})
</script>
Loops in Vue
The next thing we'll see is the use of loops in Vue; for it is the directive v-for to which a collection of elements must reference; for example:
<div id="app">
<ul>
<li v-for="post in posts" :key="post.id">{{post.title}}</li>
</ul>
</div>
<script src="vue.js"></script>
<script>
let vm = new Vue({
el: '#app',
data: {
posts: [
{id: 1, title:"Entrada 1"},
{id: 2, title:"Entrada 2"},
{id: 3, title:"Entrada 3"}
]
}
})
</script>
We see several interesting features, the first thing we see is that we define an unordered list to show the array elements that we have defined as a property that simulates the content of 3 entries; in the v-for directive that is inside the li element we define a key that is optional to indicate the identifier of the element; it is something internal to Vue, the next thing we see is the basic rendering of the posts property that we treat as if it were an array; the output is the following:
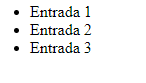
User events
With Vue we can control the user events, click, focus and others using the v-on directive followed by the event that we are going to register; for example for the click event:
<script src="vue.js"></script>
<div id="app">
<h1 :style="{color:'red', fontSize: fontSize+'px'}">{{message}}</h1>
<button @click="increateFont">Increase</button>
<button @click="decreateFont">Decrease</button>
</div>
</div>
<script>
let vm = new Vue({
el: '#app',
data: {
message: "hola",
fontSize:12
},
methods:{
increateFont(){
this.fontSize++
},
decreateFont(){
this.fontSize--
}
}
})
</script>
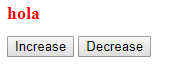
I agree to receive announcements of interest about this Blog.
We will talk about what Vue is, what it is for, features, how to install and use Vue, we will compare Vue with jQuery and some examples to start with Vue
- Andrés Cruz