In this post, we are going to continue working on creating our applications in SwiftUI; previously we talked a little about SwiftUI, which is Apple's new framework to create our applications in a declarative way; In other words, SwiftUI is Google's Flutter but in the best Apple style:
Get started with SwiftUI to build your declarative interface.
In addition to this, we saw what is the environment that we must use to develop our first apps, which has been xCode 11 from iOS 13, some examples of lists, foreach, etc. to get used to its syntax, its form of organization and some resources to get started with SwiftUI.
In summary Swiftui is a simple declarative interface with which we can build our interfaces in the Apple ecosystem.
Creating a basic app with SwiftUI
Today we are going to start creating a fairly simple app which consists of some texts, a central image and a container element to place all this and we are going to create a text, for that we use the Text struct:
import SwiftUI
struct texto_imagen: View {
var body: some View {
Text("Hello World")
}
}
And we get:
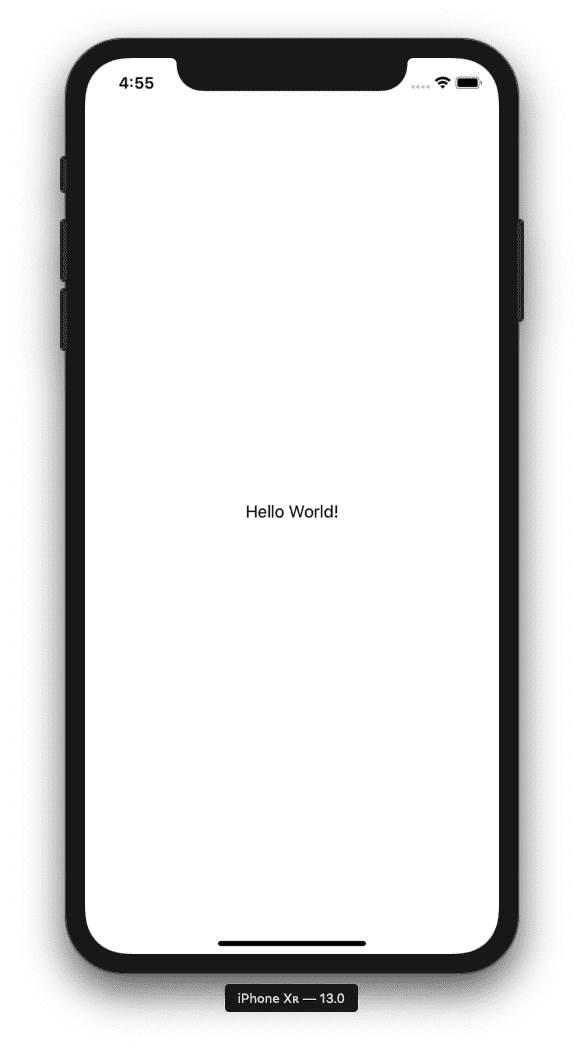
Everything starts here, as you can see, this is why SwiftUI is a declarative interface framework, since in the same file in which we are declaring the functionality (like our controller), this same view element also displays what It would be an interface, that is, that Text to which we can apply some logic also renders a text in our interface without the need to apply a separate view file as it would be in Android with the layouts or in classic Swift with the Main Storyboard.
How do you see we see our text on our screen; now we want to add a simple image; for that we use the Image specifying the image that must be loaded in the Assets.xcassets folder in the traditional way, then we reference it by name and that's it; Would you think we can do the following:

import SwiftUI
struct texto_imagen: View {
var body: some View {
Image("final_udemy")
Text("Hello World")
}
}
And we get an error:
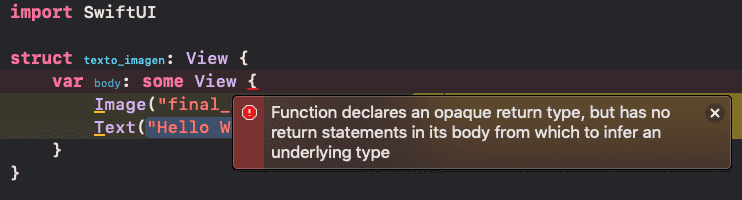
The VStack in Swiftui for the structure of our application
But this will give us an error since we cannot use multiple elements in the root, for that we need a root element, an element that allows us to add other structs as well as in Flutter, we use -for example- the Column widget to add a list of widgets, which in other words means one or more widgets; here we can also do the same and use a container element; in this case, since we want to place everything as if it were a stack, that is, one on top of the other, we are going to use what would be a VStack-type container and within it, the code of our image and the text:
import SwiftUI
struct texto_imagen: View {
var body: some View {
VStack {
Image("final_udemy")
Text("Hello World")
}
}
}
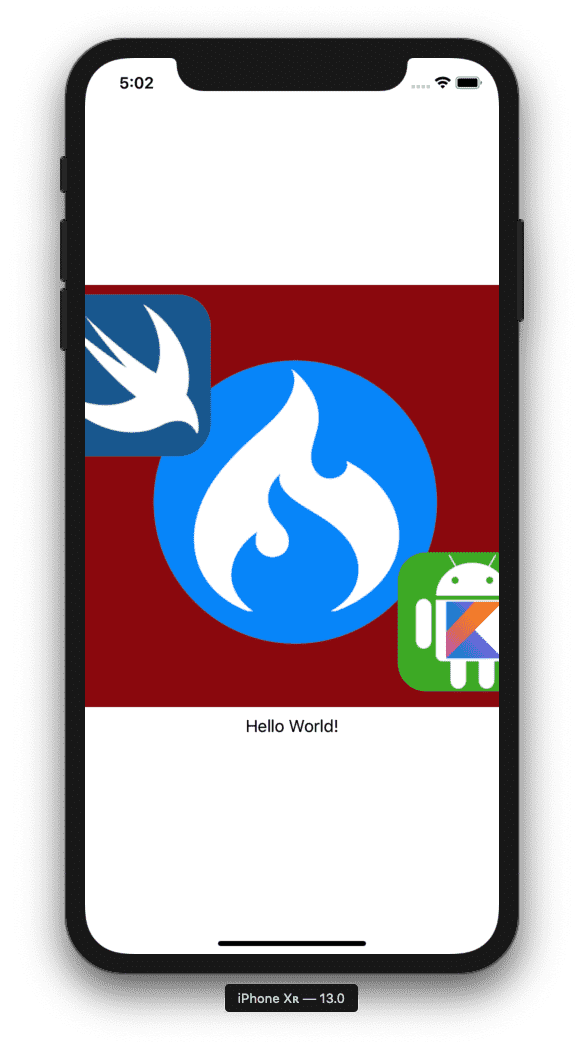
In this case, place an image of my course on Udemy in which we teach how to create our first mobile apps with Android and iOS and connect them to a Rest API.
Modifiers on our view elements
Here's the kicker, from our file, we can call functions with parameters which allow us to alter the style, position, and behavior of our elements; these same ones are known as modifiers since they allow us to "modify" or vary the style, position and/or behavior of our view elements that today we have seen three:
Text
Image
VStak
Now, before we get into some modifiers we're going to want to add a title to our image and a more original description, and with our VStack this works perfectly for us:
import SwiftUI
struct texto_imagen: View {
var body: some View {
VStack {
Text("Aprende a crear apps en Android e iOS con una RestAPI en PHP")
Image("final_udemy")
Text("Crear tus apps nativas para Android e iOS y conectalas a una Rest Api en CodeIgniter 3: m贸dulo de gesti贸n con jQuery, Bootstrap 4, roles de usuarios, Retrofit, Alamofire, peticiones Post, Get, creaci贸n de Rest Api, primeros pasos con Kotlin y Swift, listados (RecyclerView, TableView), SwiftUI, uploads de avatares desde las apps m贸viles y mucho m谩s")
}
}
}
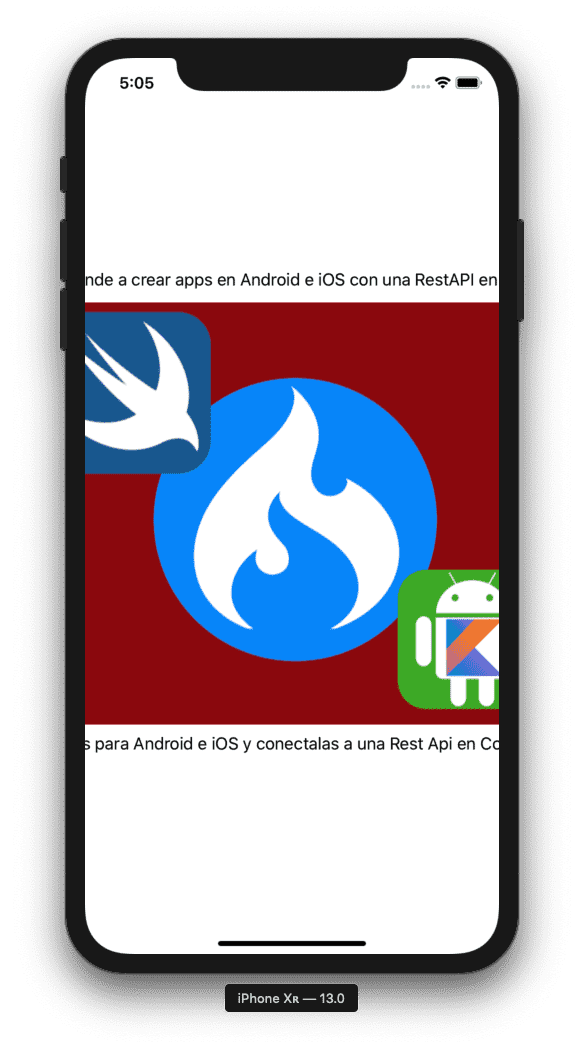
As you can see, everything is stacking up perfectly; but as you can see we have several problems, the first one is that the text is cut and only one line appears, the next one would be that the upper and lower text have the same presentation; We are going to vary it a bit with the font modifier/function to vary the text and another modifier to indicate that it presents the content in several lines if necessary:
import SwiftUI
struct texto_imagen: View {
var body: some View {
VStack {
Text("Aprende a crear apps en Android e iOS con una RestAPI en PHP")
.lineLimit(3)
.font(.title)
Image("final_udemy")
Text("Crear tus apps nativas para Android e iOS y conectalas a una Rest Api en CodeIgniter 3: m贸dulo de gesti贸n con jQuery, Bootstrap 4, roles de usuarios, Retrofit, Alamofire, peticiones Post, Get, creaci贸n de Rest Api, primeros pasos con Kotlin y Swift, listados (RecyclerView, TableView), SwiftUI, uploads de avatares desde las apps m贸viles y mucho m谩s")
.lineLimit(nil)
.font(.body)
}
}
}
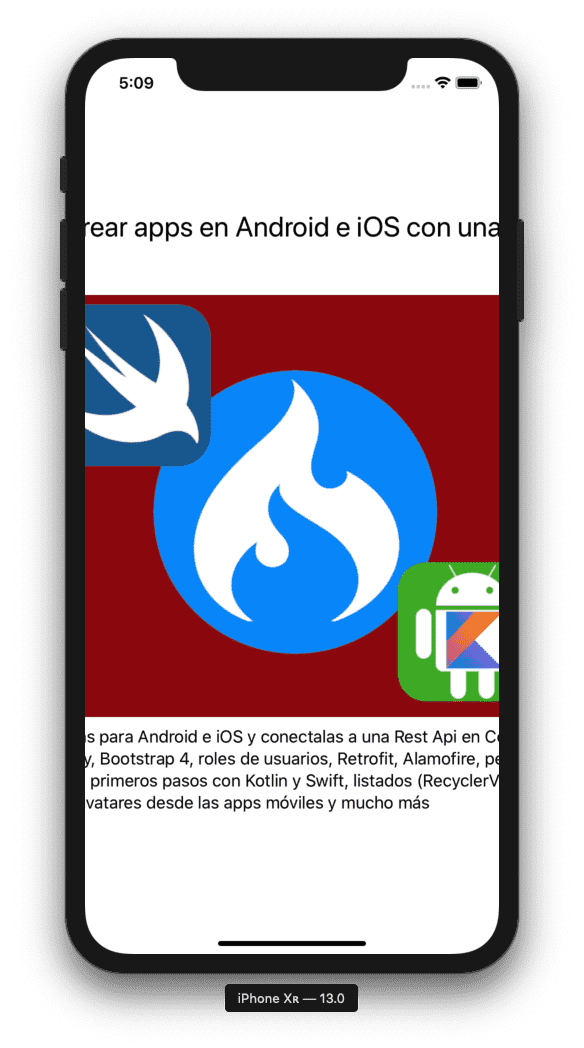
As you can see it comes out a bit better but we still have some issues but before that as you can see we added a couple of modifiers one to indicate the font but you are free to explore and try different combinations but as you can see , it receives different parameters and some values such as body, title, largeTitlee, etc. Which automatically vary the presentation of our text.
Regarding the text lines, now you can see that for the description it is now presented in several lines, and we achieve this with the lineLimit modifier that receives a number that indicates the maximum number of lines that the text will have, or nil so that iOS apply the number of lines on our elements.
We are going to solve the problem with the texts and images, as you can see, the image is very large and it stretches our container, which also causes it not to be completely visible on the screen, we can fix this by indicating a size to the image; For that we use the frame modifier:
struct texto_imagen: View {
var body: some View {
VStack {
Text("Aprende a crear apps en Android e iOS con una RestAPI en PHP")
.lineLimit(3)
.font(.title)
Image("final_udemy")
.resizable()
.frame(width: 375, height: 211)
Text("Crear tus apps nativas para Android e iOS y conectalas a una Rest Api en CodeIgniter 3: m贸dulo de gesti贸n con jQuery, Bootstrap 4, roles de usuarios, Retrofit, Alamofire, peticiones Post, Get, creaci贸n de Rest Api, primeros pasos con Kotlin y Swift, listados (RecyclerView, TableView), SwiftUI, uploads de avatares desde las apps m贸viles y mucho m谩s")
.lineLimit(nil)
.font(.body)
}
}
}
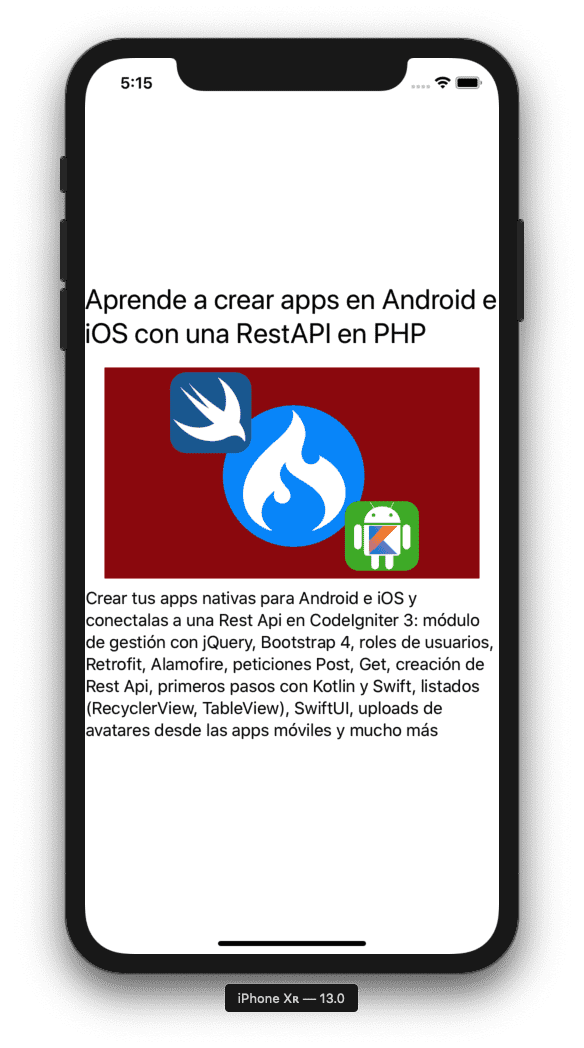
We also apply the resizable modifier that has to be accompanied by the frame modifier to indicate that we want to resize the image; as a task, comment out or temporarily remove the resizable modifier and you'll see the behavior.
Now we see everything much better than before, at least our application looks better, however now everything is very stuck together, we are going to apply another modification but this time on our image since it is our central element and in this way we do not have to apply a padding for each of the texts; We'll also apply padding to our container:
struct texto_imagen: View {
var body: some View {
VStack {
Text("Aprende a crear apps en Android e iOS con una RestAPI en PHP")
.lineLimit(3)
.font(.title)
Image("final_udemy")
.resizable()
.frame(width: 375, height: 211)
.padding()
Text("Crear tus apps nativas para Android e iOS y conectalas a una Rest Api en CodeIgniter 3: m贸dulo de gesti贸n con jQuery, Bootstrap 4, roles de usuarios, Retrofit, Alamofire, peticiones Post, Get, creaci贸n de Rest Api, primeros pasos con Kotlin y Swift, listados (RecyclerView, TableView), SwiftUI, uploads de avatares desde las apps m贸viles y mucho m谩s")
.lineLimit(nil)
.font(.body)
}.padding()
}
}
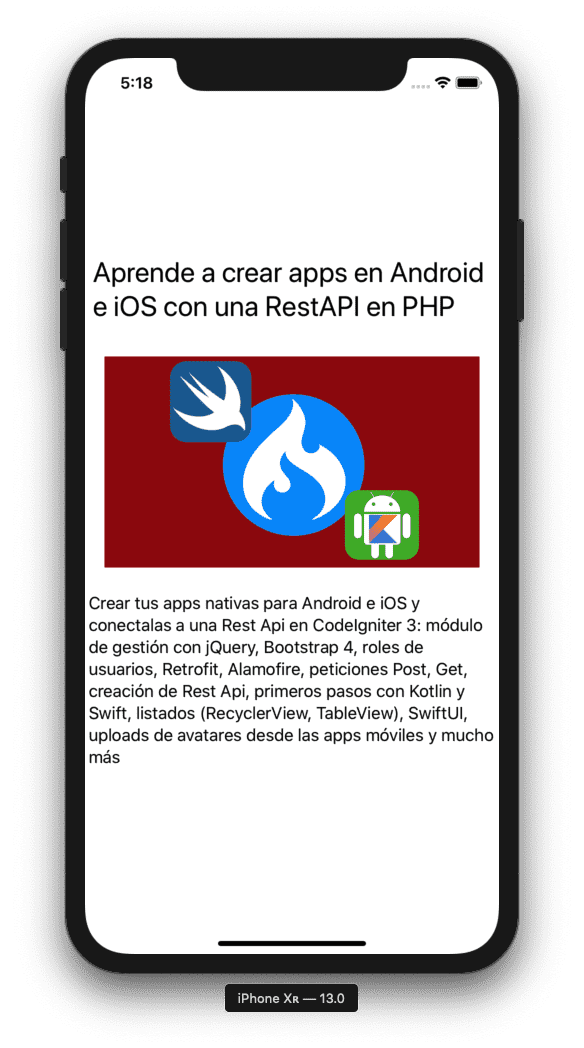
Just by placing padding that, as you can see, corresponds to another modifier, a margin is applied automatically, but it can receive different values that indicate the number of points that the padding will have; For example, 30 points to apply on each of its sides, that is, on the top, left, right and bottom:
struct texto_imagen: View {
var body: some View {
VStack {
Text("Aprende a crear apps en Android e iOS con una RestAPI en PHP")
.lineLimit(3)
.font(.title)
Image("final_udemy")
.resizable()
.frame(width: 375, height: 211)
.padding(30)
Text("Crear tus apps nativas para Android e iOS y conectalas a una Rest Api en CodeIgniter 3: m贸dulo de gesti贸n con jQuery, Bootstrap 4, roles de usuarios, Retrofit, Alamofire, peticiones Post, Get, creaci贸n de Rest Api, primeros pasos con Kotlin y Swift, listados (RecyclerView, TableView), SwiftUI, uploads de avatares desde las apps m贸viles y mucho m谩s")
.lineLimit(nil)
.font(.body)
}.padding()
}
}
It as well as all modifiers receive different builders which you are free to explore.
Always remember to help you with the autocompletion and hints for parameters and view elements that xCode offers to develop your apps in SwiftUI and in general.
Conclusion
With this simple example, we have been able to create a simple application that, although it is not the most functional in the world, we could see that with a few lines of code and with a single file we can build complete interfaces without much effort, we learned to use some view elements like this as its most basic modifiers, but in future posts we will see how all this can be further enhanced and also new structures and view elements; In the next entry we will see how to create lists and foreach and other aspects of interest.
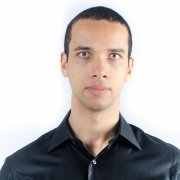
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter