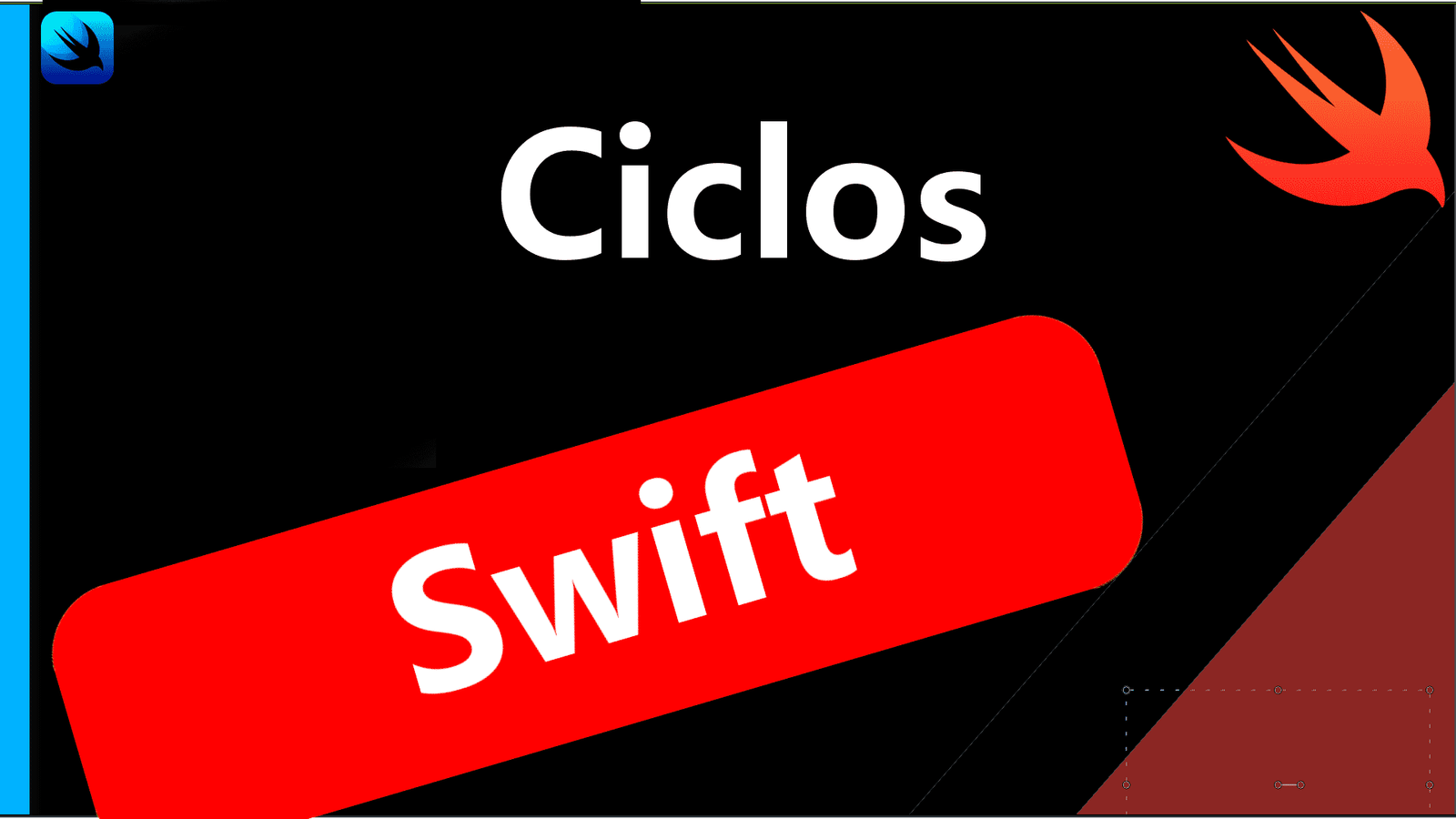
Today we are going to talk about another of the basic elements in any programming language, such as cycles or also called loops that, as you should know, allow a group of iterations to be executed a certain number of times over a block of code, and this number of repetitions is established or marked by a condition in the style of the conditionals we discussed in the previous entry.
In this post we are going to talk about the for and while loops in Swift.
Although as we are going to see, each of them has its variation that we are going to discuss below:
The for in Swift
There are many variations that you can apply to a for type loop, generally all of them are followed by the in which immediately allows us to specify the type of structure that we can iterate, which can be from ranges to arrays, all with their variations and with the possibility of combining all of them. the schemes that we bring you here; remember that we present the most common schemes but there are more.
for
There are many variations that you can apply to a for type loop, generally all of them are followed by the in which immediately allows us to specify the type of structure that we can iterate, which can be from ranges to arrays, all with their variations and with the possibility of combining all of them. the schemes that we bring you here; Remember that we present the most common schemes but there are more.
for i in 1...5 {
print(i)
}
Here we get as output:
12345
In the previous example we show a variable called i that takes the first value of one, since that is the first value of the range 1..5, and as this value is executed it increases by one until it reaches the end of the range. which for this example would be 5.
In it we can place any type of logic we want, for example, if we want to make a distinction between even and odd values:
for i in 1...10 {
if i%2 == 0{
print("Es par \(i)")
} else {
print("Es impar \(i)")
}
}
The result would be:
Es impar 1
Es par 2
Es impar 3
Es par 4
Es impar 5
Es par 6
Es impar 7
Es par 8
Es impar 9
Es par 10
This is the default structure and is usually used to iterate collections such as lists or arrays.
For in and where
Now, suppose we have a very large list or range, and we only want to process some of them and not the entire collection to save computing resources, according to a conditional as we explained in the previous post, then; How do we do so that the for is only executed based on a condition?; For that we can use the where structure as we see below where only the even values will be displayed:
for i in 1...10 where i % 2 == 0{
print("Es par \(i)")
}
The result would be:
Es par 2
Es par 4
Es par 6
Es par 8
Es par 10
As you can see, we simply added a conditional structure, as we explained in the previous video.
For in in reverse (reversed)
Now, suppose we are interested in processing a list backwards, although this is a function of ranges, it is important to know that we can also do this kind of definition when we are creating a for in Swift:
for i in (1...5).reversed(){
print("Val \(i)")
}
The result would be:
Val 5
Val 4
Val 3
Val 2
Val 1
For in decreasing values
If we want to go from greater to lesser, we can implement a structure like the following in which we decrease values from 5 to 1:
for i in stride(from: 5, to: 0, by: -1) {
print("Val \(i)")
}
The result would be:
Val 5
Val 4
Val 3
Val 2
Val 1
For in increment on custom values
We can also customize how much our variable will increment or decrement by using the stride function as follows:
for i in stride(from: 0, to: 10, by: 2) {
print("Val \(i)")
}
The result would be:
Val 0
Val 2
Val 4
Val 6
Val 8
Loop through arrays with for in
As we indicated at the beginning of the entry, the most common use that we can give to the for is that they iterate a list or array type object as we present in the following case:
let numeros = [5, 10, 15, 20, 25, 30, 100]
for i in numeros {
print("Val \(i)")
}
We can also vary its implementation as follows:
numeros.forEach { i in
print("Val \(i)")
}
The result would be:
Val 5
Val 10
Val 15
Val 20
Val 25
Val 30
Val 100
Get index and value
We can customize our for so that it also indicates the index of the iteration, in addition to its value:
for (index, i) in numeros.enumerated() {
print("Val \(i) - Indice \(index)")
}
The result would be:
Val 5 - Indice 0
Val 10 - Indice 1
Val 15 - Indice 2
Val 20 - Indice 3
Val 25 - Indice 4
Val 30 - Indice 5
Val 100 - Indice 6
The while loop in Swift
For the while loop in Swift it's pretty much the same as in any programming language; We have a couple of variations, the traditional while and the so-called do-while or here in Swift it would be repeat-while:
while
The basic structure of the while is that as long as a condition is not executed, then it will continue to be executed, unlike for in which we can see a number of well-specified interactions, not here:
while val > 10 {
print(val)
val += 1
}
And we get as result:
0
1
2
3
4
5
6
7
8
9
Now, if we set the following configuration:
var val = 10
while val > 10 {
print(val)
val += 1
}
The loop is not executed, since the first thing the while does is check if the condition applies, but as we saw in the previous case, this is false from the beginning since 10 is not less than 10 and therefore the loop ends before starting, but if we need it to be executed at least once regardless of the initial condition, we can use the following structure.
repeat while
Here we are going to be sure that at least once our code is going to be executed:
var val = 10
repeat {
print(val)
val += 1
} while val > 10
And we get as output:
10
As you can see, it's the same syntax, only we move the while check to the end and we place the repeat keyword at the beginning.
If we start from the initial example, where val had a value of zero, we will see that it is the same output, since when val reaches the value of 10, immediately the next line of code that is executed is the while check:
var val = 10
repeat {
print(val)
val += 1
} while val > 10
I agree to receive announcements of interest about this Blog.
We are going to explain the loops in Swift, we will see the for and some of its main variations, the while and the repeat-while.
- Andrés Cruz