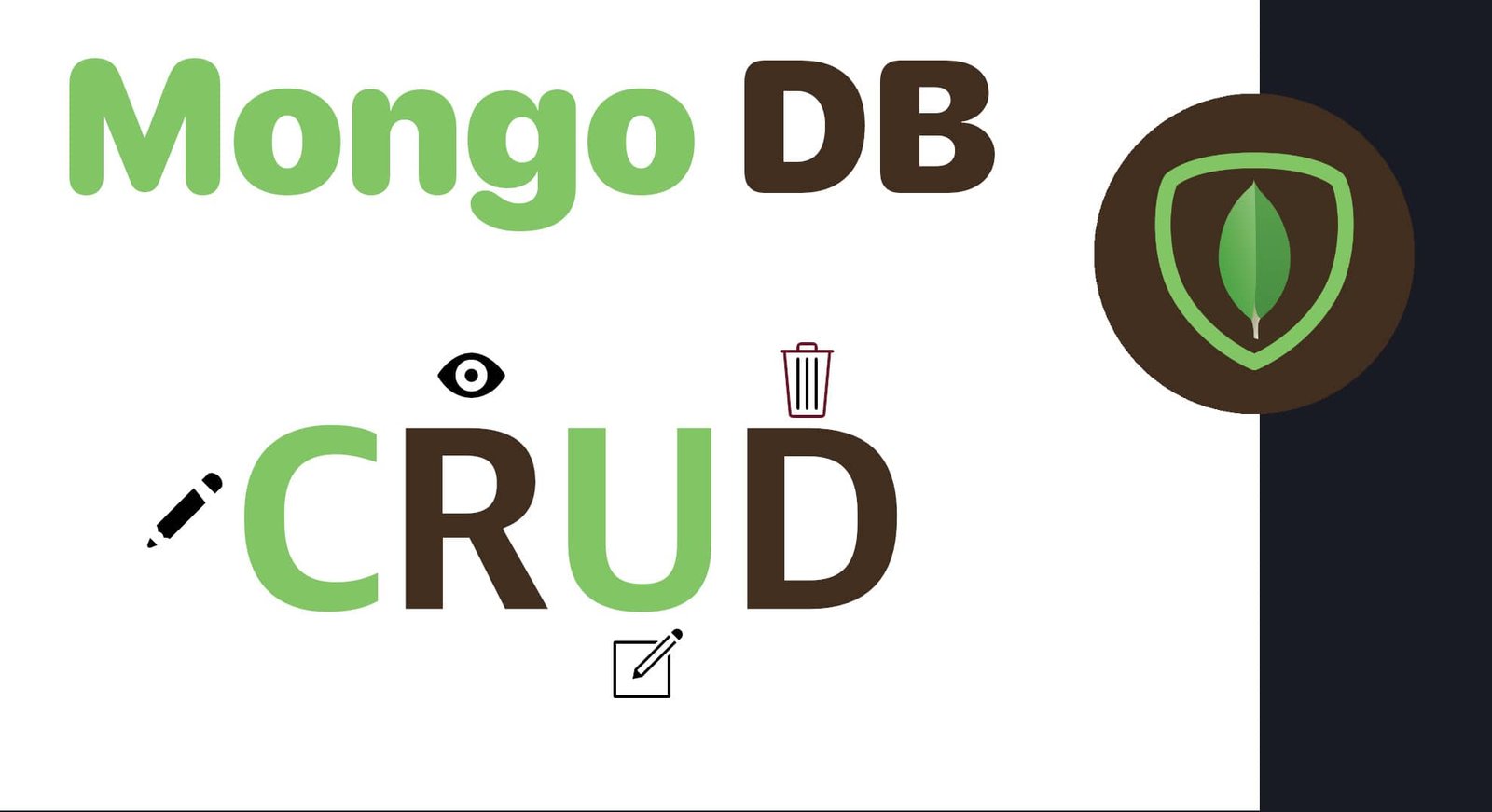
We are going to know how we can perform CRUD type operations using MongoDB, remember that CRUD type operations are those that allow us to Create, Read, Update and Delete (CRUD) respectively.
Operations to create
Create or insert operations add new documents to a collection. If the collection does not currently exist, insert operations will create the collection.
MongoDB provides the following methods to insert documents into a collection:
db.collection.insertOne()
db.collection.insertMany()
Example operations:
var user2 = {
name: 'Andres',
last_name: 'Cruz',
age: 29,
email: 'andres@gmail.com'
}
var user3 = {
name: 'Pablo',
last_name: 'Lama',
age: 30,
email: 'pablo@gmail.com'
}
var user4 = {
name: 'Luis',
last_name: 'Yello',
age: 99,
email: 'pepe@gmail.com'
}
db.users.insertOne(user2)
db.users.insertMany(
[user3, user4, user2]
)
Which allow inserting a single record or more than one respectively.
Read operations
Read operations retrieve documents from a collection; that is, consult a collection of documents. MongoDB provides the following operation to read documents from a collection:
db.collection.find()
Operaciones de ejemplo:
db.users.find(
{ age: 25 },
{ name: true, email: true, _id: false }
).pretty()
db.users.find(
{ age: 25 },
{ email: false, _id: false }
).pretty()
Operations to Update
Update operations modify existing documents in a collection. MongoDB provides the following methods to update documents in a collection:
db.collection.updateOne()
db.collection.updateMany()
db.collection.replaceOne()
Operaciones de ejemplo:
db.users.updateMany(
{
name: {
$exists: true
}
},
{
$set: {
name2: "Luisito"
}
}
)
db.users.updateOne(
{
name: {
$exists: true
}
},
{
$unset: {
name2: "Luisito"
}
}
)
Delete Operations
Delete operations remove documents from a collection. MongoDB provides the following methods to remove documents from a collection:
db.collection.deleteOne()
db.collection.deleteMany()
Example operations:
db.users.deleteOne({"_id": new mongo.ObjectID(id) })
db.users.deleteMany({"_id": new mongo.ObjectID(id) })
I agree to receive announcements of interest about this Blog.
Let's learn how we can perform CRUD type operations using MongoDB from its command line.
- Andrés Cruz