Laravel sanctum is wonderful for when we want to protect a Rest API created with Laravel either through tokens or a hybrid between section and cookies; before showing you how you can do the more traditional implementation, let's get into the subject a bit and introduce what Sanctum is.
What is Laravel Sanctum?
Laravel Sanctum is an authentication package for Laravel that provides a simple and secure method to authenticate Single Page Applications (SPAs) by users and protect your API using API tokens. Sanctum allows you to generate and manage API tokens, which are issued through a rest resource that we implement and which is passed to the client so that when they want to access protected resources, they must use said access token and be able to connect to the resource and consume the API response.
These tokens are great since you can use them to authenticate API requests and also to restrict access to routes, since, with it, from Laravel you know which user it is that tries to consume a certain resource and perform some trace or limitation.
Generate access tokens
Laravel Sanctum provides us with a simple mechanism with which to add authentication to Rest Api, through authentication token or authentication tokens, in this first entry, we will see how to use the authentication token mechanism that would be the most traditional scheme.
It is worth mentioning that it is not necessary to install Sanctum since, for a long time, Sanctum has been part of a project in Laravel.
In our rest controller, to handle the user, in the case of our course in Laravel, it would be the following:
app\Http\Controllers\Api\UserController.php
We will create a login function, using the attempt function with which, we can check user credentials that are provided in the request:
$credentials = [
'email' => $request->email,
'password' => $request->password
];
Once the credentials are verified, we create the token and return the response:
Auth::user()->createToken('myapptoken')->plainTextToken;
Finally, the complete code:
public function login(Request $request)
{
$credentials = [
'email' => $request->email,
'password' => $request->password
];
if (Auth::attempt($credentials)) {
$token = Auth::user()->createToken('myapptoken')->plainTextToken;
session()->put('token', $token);
return response()->json($token);
}
return response()->json("Usuario y/o contraseña inválido", 422);
}
The most important thing is to note that, although sanctum provides all the mechanisms to generate the token, it is up to the user to implement the necessary logic to generate the token.
More examples with Sanctum
Here I leave you other examples with authentication in Laravel sanctum and protection of routes.
In case the project no longer has Sanctum, you must install Laravel Sanctum using Composer:
composer require laravel/sanctum
Then, you will publish the Sanctum configuration and migration files:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
php artisan migrate
Now we can protect our routes by using an authentication middleware in your routes file. For example:
routes/api.php
Route::middleware('auth:sanctum')->group(function () {
Route::get('/post', function () {
return view('dashboard');
});
});
In this example, the "/post" path will only be accessible if the user is authenticated using the Sanctum middleware.
After that, you can generate an API token for an authenticated user. For example:
$token = $user->createToken('token-name')->plainTextToken;
This code will generate an API token for the given user with the name "token-name". You can then use the token to authenticate API requests; here is another example of logging in with sanctum:
routes/api.php
use App\\Models\\User;
use Illuminate\\Http\\Request;
use Illuminate\\Support\\Facades\\Hash;
Route::post('/login', function (Request $request) {
$user = User::where('email', $request->email)->first();
if (! $user || ! Hash::check($request->password, $user->password)) {
throw ValidationException::withMessages([
'email' => ['The provided credentials are incorrect.'],
]);
}
$token = $user->createToken($request->device_name)->plainTextToken;
return ['token' => $token];
});
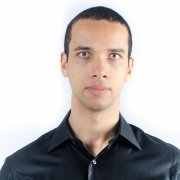
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter