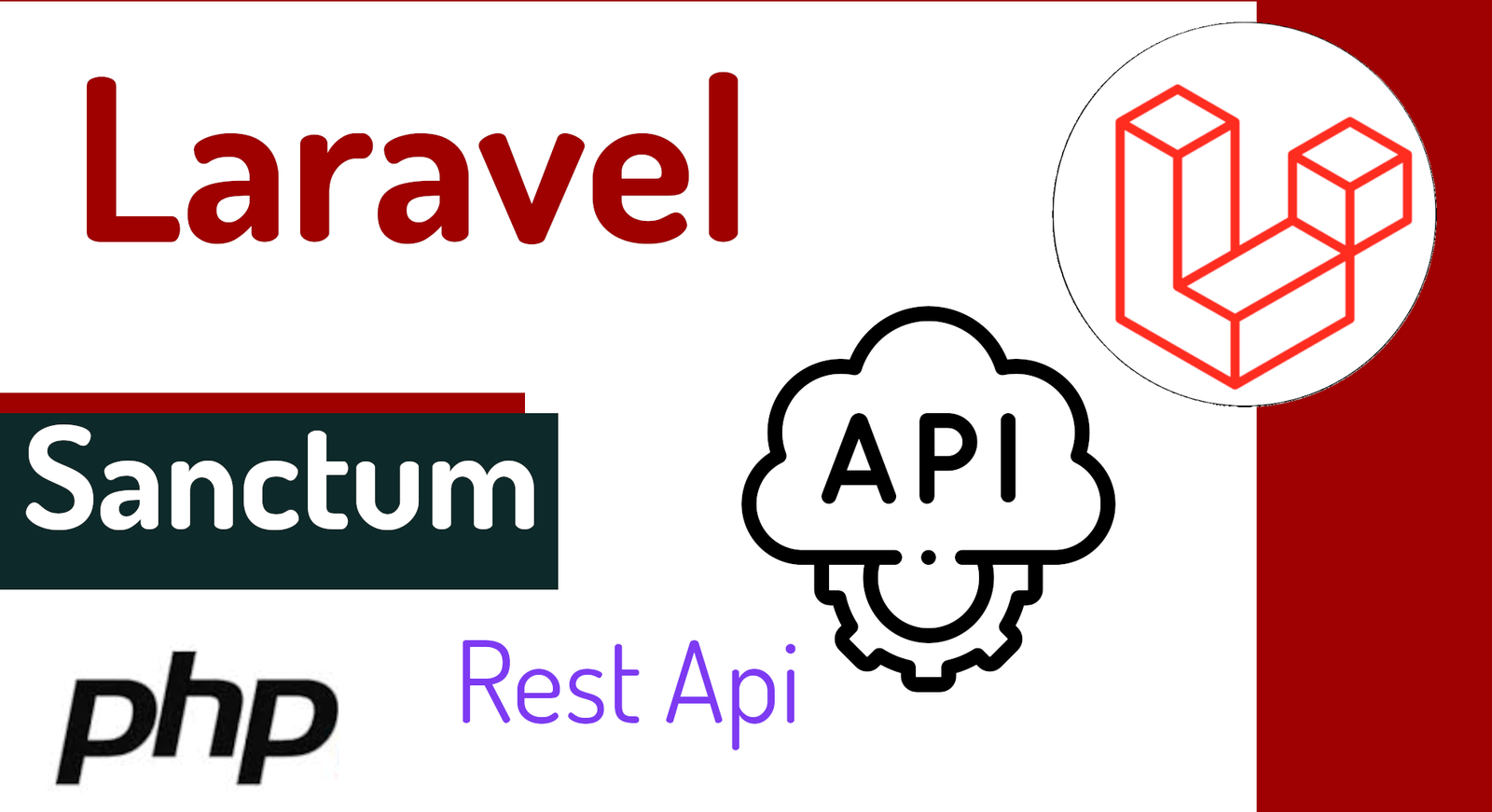
Laravel Sanctum is a package for the authentication of single page applications (SPAs) and mobile applications that we can use to protect the Rest API through required authentication; Laravel Sanctum and simple token-based APIs; remember that it is not as direct as adding a session, and this is because a Rest API is recommended to be stateless and this is where Laravel Sanctum comes into play. Allows each user of your application to generate multiple API tokens for their account. Laravel Sanctum provides a lightweight authentication system for SPAs and simple APIs
Using Laravel Santum we ensure that only authenticated users can access the routes that require it.
The idea behind Sanctum is to protect the APIs of Laravel applications through authentication and access tokens. Doing so ensures that only authenticated and authorized requests are allowed, which in turn ensures the integrity of the application and the data involved. I hope this has helped you understand a bit more about the theory behind Laravel Sanctum.
Laravel Sanctum nos ofrece dos esquemas para trabajar.
1 Authentication via SPA
This is useful when we have a SPA page and want to add authentication to protect a Rest API and access the user; this option is based on a scheme between session authentication and cookies that we have to configure; that is, it is the simpler of the two:
At the kernel level, we have to enable the cookie and session service for Sanctum:
//App/Http/Kernel
//***
class Kernel extends HttpKernel
{
//***
'api' => [ \Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
];
Specify the domain you are going to use for cookie-based authentication in the config/sanctum.php file:
'stateful' => explode(',', env('SANCTUM_STATEFUL_DOMAINS', sprintf(
'%s%s',
'localhost,localhost:3000,127.0.0.1,127.0.0.1:8000,::1,laraprimerospasos.test',
env('APP_URL') ? ','.parse_url(env('APP_URL'), PHP_URL_HOST) : ''
))),
If you're using Mac or Linux with Sail, you don't need to modify this file since localhost is already present; also, you can put your domain URL in production.
In the config/cors.php file you have to enable the supports_credentials to true.
In order to use axios (which we will do later), you must find the file resources/js/bootstrap.js and place:
axios.defaults.withCredentials = true;
This is to enable the use of cookies with the user's credentials, and to be able to use it transparently with axios.
Finally, with this, we can use the middleware for the authentication of the routes; in an exemplary way, we will protect a set of routes:
Route::group(['middleware' => 'auth:sanctum'], function () {
Route::resource('category', CategoryController::class)->except(["create", "edit"]);
Route::resource('post', PostController::class)->except(["create", "edit"]);
});
For the rest, all you have to do is log in and we are ready; you can use this scheme with axios.
2 Based on API Tokens
The following scheme is more manual and in the end it is an authentication based on tokens; for this, now when we authenticate ourselves, we generate the authentication tokens:
Before we start, let's disable the old Sanctum scheme for authentication via SPA:
'api' => [ //\Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
***
],
Create tokens
To create the tokens for our users, we are going to create a login function that allows us to generate these tokens (you could also use this function for authentication via SPA but without generating the token):
php artisan make:controller Api/UserController
In which, we will define the following content:
<?php
namespace App\Http\Controllers\Api;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
class UserController extends Controller
{
public function login(Request $request)
{
$credentials = [
'email' => $request->email,
'password' => $request->password
];
if(Auth::attempt($credentials)){
$token = Auth::user()->createToken('myapptoken')->plainTextToken;
return response()->json($token);
}
return response()->json("Usuario y/o contraseña inválido");
}
}
It is important to note that Sanctum is already installed in modern Laravel projects; all of this material is part of my complete Laravel course and my book on Laravel.
In the entry in which we create an authentication token with Laravel Sanctum we see how to continue working with santum.
I agree to receive announcements of interest about this Blog.
Configure Laravel Sanctum for SPA authentication and API Tokens
- Andrés Cruz