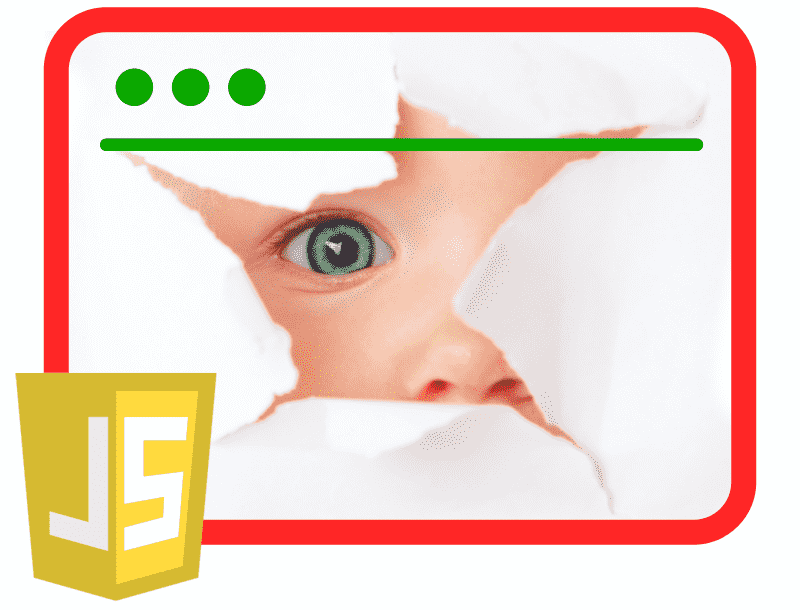
The Page Visibility API allows us to know when a web page is being viewed by the user and therefore we can make configurations to avoid the use of unnecessary resources once the user is not visiting the page in question; the following image indicates which pages are being displayed and which are not:
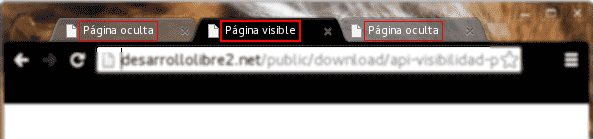
That is, when the user moves to another tab or minimizes the window; an event called visibilitychange is activated to indicate that the page is not being displayed (hidden); this can be quite useful for customizing sections of the web page; for example the one presented by the MDN.
In this post we will explain how to use the Page Visibility API, which is one of the other JavaScript APIs that we have covered in DesarrolloLibre:
- Geolocalización con JavaScript
- La API Vibration (Vibración) en HTML5
- La API Battery (Batería) en HTML5
- Introducción a las notificaciones en JavaScript
The Page Visibility API in practice
In this part we will introduce ourselves to the use of the API by explaining its main components.
Access to the Page Visibility API in JavaScript
The document.hidden property allows you to access the Page Visibility API; However, due to the large number of browsers and their versions, some may not support this API or it may be necessary to use prefixes.
The following function allows you to verify if the browser supports the Page Visibility API and obtain its prefix if it warrants it; It can be easily adapted for any of the JavaScript APIs:
// Obtenemos el prefijo para cada navegador
function getPrefix() {
// verificamos si el navegador soporta el prefijo por defecto
if ('hidden' in document)
return NULL;
// otros prefijos posibles
var prefixes = [ 'moz', 'ms', 'o', 'webkit' ];
for (var i = 0; i < prefixes.length; i++) {
var testPrefix = prefixes[i] + 'Hidden';
if (testPrefix in document)
return prefixes[i]; // retornamos el prefijo
}
// el navegador no soporta la API
return NULL;
}
Once we have the browser prefix to access the API, we can obtain access to the property in question using the following function that receives the previously obtained prefix as a parameter:
// Obtenemos la propiedad
function getHiddenProperty(prefix) {
if (prefix) {
return prefix + 'Hidden';
} else {
return 'hidden';
}
}
Page Visibility API Events
Using again the prefix obtained in the function defined above, we obtain the visibilitychange event in its variation depending on the browser used:
// Obtenemos el prefijo para acceder al evento visibilitychange
function getVisibilityEvent(prefix) {
if (prefix) {
return prefix + 'visibilitychange';
} else {
return 'visibilitychange';
}
}
Finally the definition of the visibilitychange event:
// Obtenemos el prefijo para acceder al evento visibilitychange
function getVisibilityEvent(prefix) {
if (prefix) {
return prefix + 'visibilitychange';
} else {
return 'visibilitychange';
}
}
You can see a final example that puts into practice everything seen so far:
I agree to receive announcements of interest about this Blog.
The Page Visibility API allows us to know when a web page is being viewed by the user and therefore we can make configurations to avoid the use of unnecessary resources.
- Andrés Cruz