Getting started with SQLite in JavaScript: the openDatabase, executeSql and executeSql methods
- Andrés Cruz
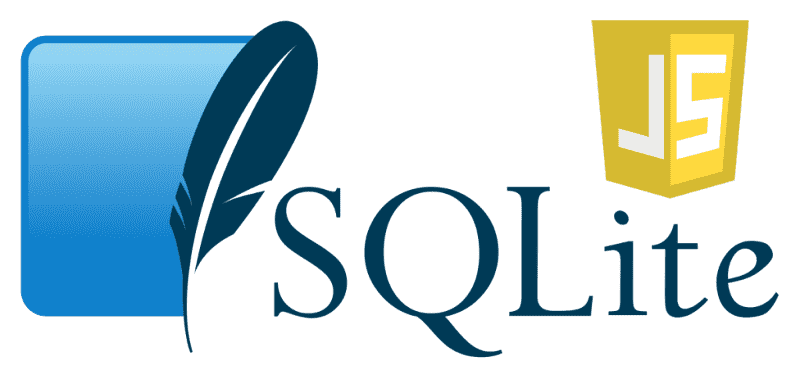
It is essential for web applications that handle high volumes of files at the time of loading by the user; be able to validate or verify the file data that makes up the file; before we had to load the file so that these validations could be carried out on the server side; It is now also possible to perform part of these validations from the client side with the File API in JavaScript.
To date, there have been talks about some JavaScript APIs that allow multiple functions to be performed and that without them would be very difficult to do; Now we will see how to work with part of the File API in JavaScript; We will see how you can obtain data from files that are loaded locally through the input type="file" and derivatives.
File API Properties in JavaScript
Once the user uploads the files, we can use the JavaScript File API to query data from the uploaded files; data such as extension, MIME, file size and in this way easily validate that it has the specified format:
- File.Name: Returns the name of the file (read only).
- File.Size: Returns the size of the file (read only).
- File.lastModifiedDate: Returns the last modification made to the file (read only).
In summary, the JavaScript file API allows you to perform a multitude of operations with any file, including images, plain text files, docs, etc.
Checking browser compatibility
As it is a technology provided from the HTML5 API, it may undergo changes from one version of the browser to another; therefore, it is necessary to validate that the browser really has the corresponding support for this API.
To verify compatibility we can use the following JavaScript code:
if (!(window.FileList)) {
console.log('La API FileList no está soportada');
return;
}
The Filelist object is referenced even if it is not used directly by this name, it allows returning a list of the selected files (for example) in an input type="file".
Obtaining information on the constitution of the files
With support guaranteed by the browser, we can now be sure that the browser has support for the properties listed above.
Getting access to user files
The most common method of uploading files to an application is by using the input type="file" tag; Once uploader with the help of file API we can get a list of files uploaded by the user and their information.
The file API 100% supports the multiple attribute present in the input type="file" tag.
Showing uploaded file data in a multiple input type="file"
The following example shows the different information obtainable through the API of files selected by a multiple input type="file":
if (!(window.FileList)) {
console.log('La API FileList no está soportada');
return;
}
var files = document.getElementById('files').files;
var output = [];
for (var i = 0, f; f = files[i]; i++) {
output.push('<li>', escape(f.name), '(', f.type || 'n/a', ') - ',
f.size, ' bytes, last modified: ',
f.lastModifiedDate.toLocaleDateString(), '</li>');
}
document.getElementById('list').innerHTML = '<ul>' + output.join('') + '</ul>';
Showing uploader file data using Drag and Drop
The following example is a variation of the previous one, and we can use Drag and Drop to load the file and later display the file information:
function drop(event) {
event.stopPropagation();
event.preventDefault();
if (!(window.FileList)) {
console.log('La API FileList no está soportada');
return;
}
var files = event.dataTransfer.files;
var output = [];
for (var i = 0, f; f = files[i]; i++) {
output.push('<li>', escape(f.name), '(', f.type || 'n/a', ') - ',
f.size, ' bytes, última modificación: ',
f.lastModifiedDate.toLocaleDateString(), '</li>');
}
document.getElementById('list').innerHTML = '<ul>' + output.join('') + '</ul>';
}
function dragenter(event) {
event.stopPropagation();
event.preventDefault();
}
function dragover(event) {
event.stopPropagation();
event.preventDefault();
}
var contenedor = document.getElementById("contenedor");
contenedor.addEventListener("dragenter", dragenter, false);
contenedor.addEventListener("dragover", dragover, false);
contenedor.addEventListener("drop", drop, false);
Conclusions
And this is all for now, we will continue talking about the file API later, exemplifying its use when we can not only list file information, but also open them for reading them.
Links of interest
I agree to receive announcements of interest about this Blog.
With SQLite in HTML5 you can store persistent information of different types (user, control, etc.) with JavaScript using the openDatabase, executeSql and executeSql methods.
- Andrés Cruz