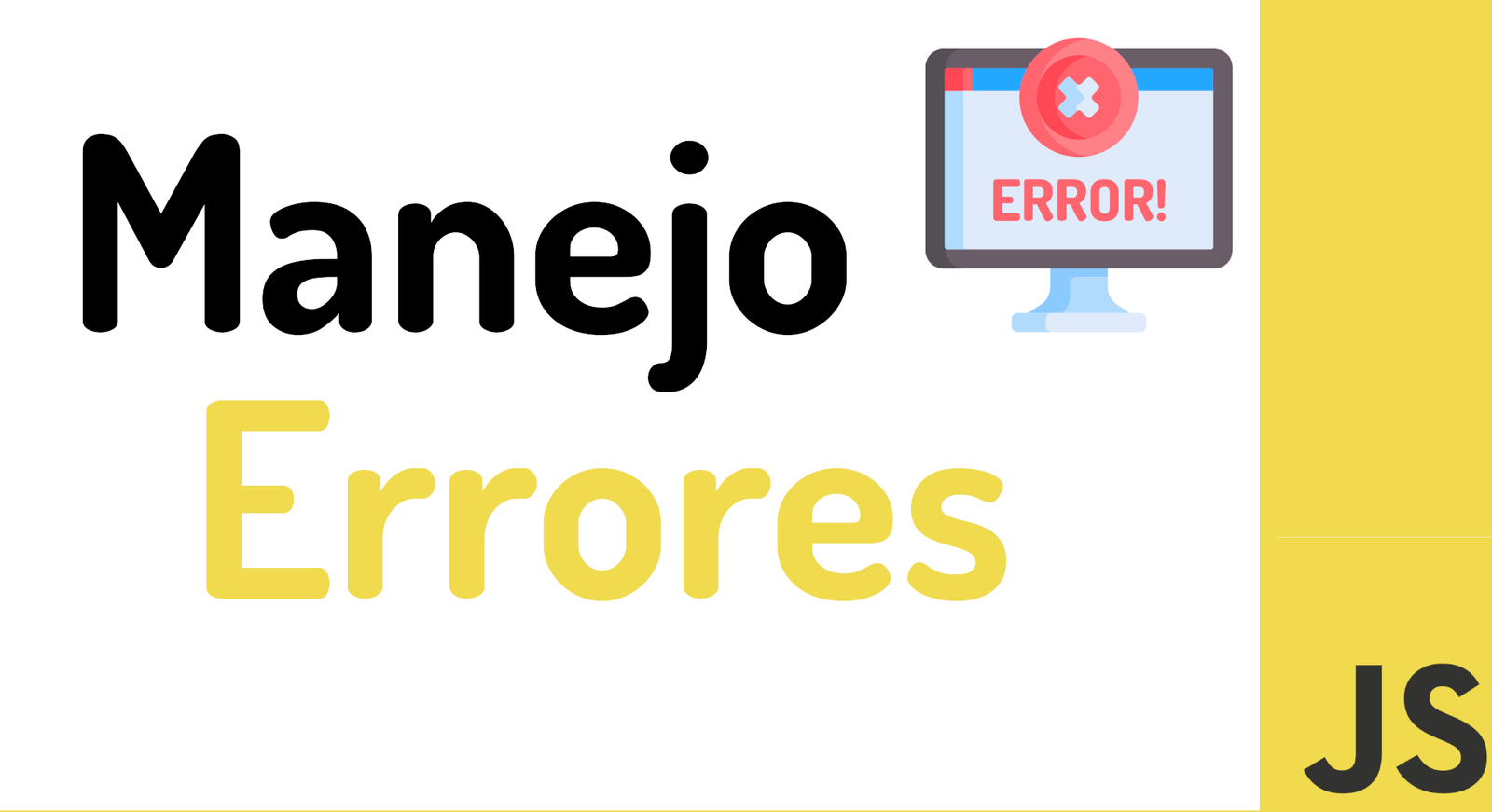
When we make an application, we will always find bugs. Is it because of our mistakes in coding or because of user input errors? A good application will create an error message as an option when the code is executed because it will make it easier for us to handle any errors that occur.
How to handle error messages to avoid failures. Alright, let's get straight to the tutorial.
Try and Catch
To handle JavaScript errors, use try and catch. Writing the try-catch code to handle the error is like this:
try {
// code
} catch (error) {
// error handling
}
In the above code, we have created the code, the first test code is to provide an opportunity for the test code to be captured and handled by the capture code block. Meanwhile, if there is no error in the code, the catch block will be ignored.
try {
console.log("first blok try");
console.log("last blok try");
} catch (error) {
console.log("No error occurs, then this code is ignored");
}
/* output
first blok try
last blok try
*/
The code in the try block above will not return an error, so the code in the catch block will be ignored and not executed. The following is a code example that generates an error:
try {
console.log("First blok try"); // (1)
errorCode; // (2)
console.log("Last blok try"); // (3)
} catch (error) {
console.log("An error occurred!"); // (4)
}
/* output
First blok try
An error occurred!
*/
Line of code (2) will produce an error. The execution of the code inside the test block will be stopped, so the line of code (3) will not be executed. The code will then continue to line (4) or the catch block.
Now consider the catch block. Hay catch has a parameter called error (variable name can be changed). An error variable is an object that stores detailed information about the error that occurred.
The error object has several main properties, namely:
- name: The name of the error that occurred.
- message: Message about the details of the error.
stack: Information about the sequence of events that caused the error. It is generally used for debugging because it contains information about which line caused the error.
Now let's try to modify the code and display the above error property.
try {
console.log("First blok try"); // (1)
errorCode; // (2)
console.log("Last blok try"); // (3)
} catch (error) {
console.log(error.name);
console.log(error.message);
console.log(error.stack);
}
/* output
Awal blok try
ReferenceError
errorCode is not defined
ReferenceError: errorCode is not defined
at file:///home/dicoding/Playground/javascript/CoffeeMachine/error.js:3:5
at ModuleJob.run (internal/modules/esm/module_job.js:152:23)
at async Loader.import (internal/modules/esm/loader.js:166:24)
at async Object.loadESM (internal/process/esm_loader.js:68:5)
*/
From the above information, we can know that the error that appears is ReferenceError because errorCode is considered to be an undefined variable or value.
try-catch-finally
In addition to try and catch, there is one more block to the error handling mechanism in JavaScript, namely finally. The block will eventually continue to execute regardless of the result of the try-catch block.
try {
console.log("First blok try");
console.log("Last blok try");
} catch (error) {
console.log("This line is ignored");
} finally {
console.log("Will still be executed");
}
/* output
First blok try
Last blok try
Will still be executed
*/
Throwing Errors
Now let's deal with a more common case. Please note the following code:
let json = '{ "name": "Johon", "age": 20 }';
try {
let user = JSON.parse(json);
console.log(user.name);
console.log(user.age);
} catch (error) {
console.log(error.name);
console.log(error.message);
}
In the above code, the JSON.parse function will parse or convert the JSON (String) variable to an object. We will run into many scenarios like the one above when making API requests.
Run the above code in your text editor. The app should run smoothly without causing any errors.
So what happens if the JSON string doesn't match the format of the JavaScript object?
try {
let user = JSON.parse(json);
console.log(user.name);
console.log(user.age);
} catch (error) {
console.log(error.name);
console.log(error.message);
}
/* output
SyntaxError
Unexpected token b in JSON at position 2
*/
If the JSON does not match the format, JSON.parse will throw an error. The catch block will catch the error and the code it contains will be executed.
https://javascript.plainenglish.io/javascript-error-handling-3ec6729ad83e
I agree to receive announcements of interest about this Blog.
We will see how to handle errors in JavaScript through the try catch and some possible variants; Handling errors in projects is a fundamental task for the good flow of the application.
- Andrés Cruz