Before we begin, let's quickly introduce what Flutter is.
Flutter is Google's framework for creating applications for Android and iOS, leaving out apps for Windows on mobile devices; Flutter's policy is similar to that of other frameworks such as Xamarin in which we can create two applications for different platforms such as Android and iOS with a single project.
A strong point that Flutter has is that it is fast when developing applications for mobile devices, in addition to this, it allows you to create native applications for these platforms and not webapps, as is the case with Ionic; and for this reason we have applications with a better finish.
Another important point is the programming language used by Flutter; Flutter uses Dark, so we have to learn this object-oriented programming language, so we can create classes, in different files, and relate them to each other.
Another important point is that Flutter uses Material Design; therefore you will see that how many components created from our application, this automatically take the design of the Material Design.
Install Flutter on our computer
To install Flutter, the first thing to do is go to the official site and download the Install Flutter installer for your operating system.
The next page details the steps to install Flutter correctly on your computer; in the case of Windows:
Flutter Windows install.
Flutter Extension for Android Studio and Visual Studio Code
You must decide which IDE you are going to use if Android Studio or Visual Studio Code; in both IDEs you can install Flutter and start creating your applications; If you don't want to complicate yourself too much, you could use Android Studio, since when you install it you already have access to the Android Simulators automatically from the IDE itself.
Likewise, for both IDEs, once Flutter is installed on your computer, you must install the extension to be able to create projects with this Framework for Material Design in mobile applications on Android and iOS.
Creating our first application: Tutorial
Once you have your environment ready to work with Flutter; as we talked a bit in the previous section; We are going to start working with Flutter; we are going to use Android Studio and with this we are ready to continue with our little Flutter course or guide; Now it's your turn to start our application.
The first thing we will do is open the IDE that you have selected; In my case it will be Android Studio which, as we have already seen, is useful for much more than Kotlin and Android.
At this point we are ready to start creating our application; for that we go to File - New - New Flutter Project:
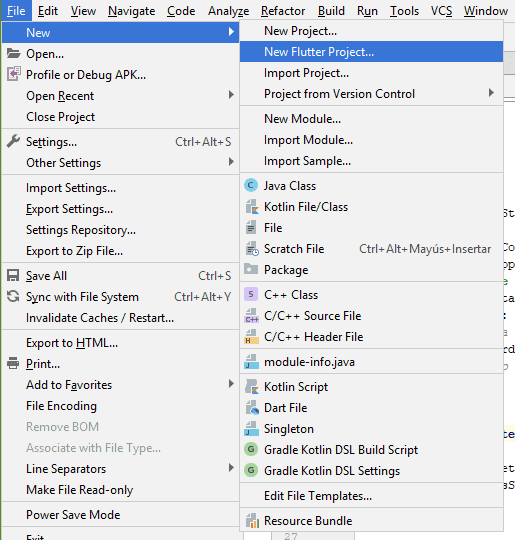
And we select the option that tells us Flutter Application
:
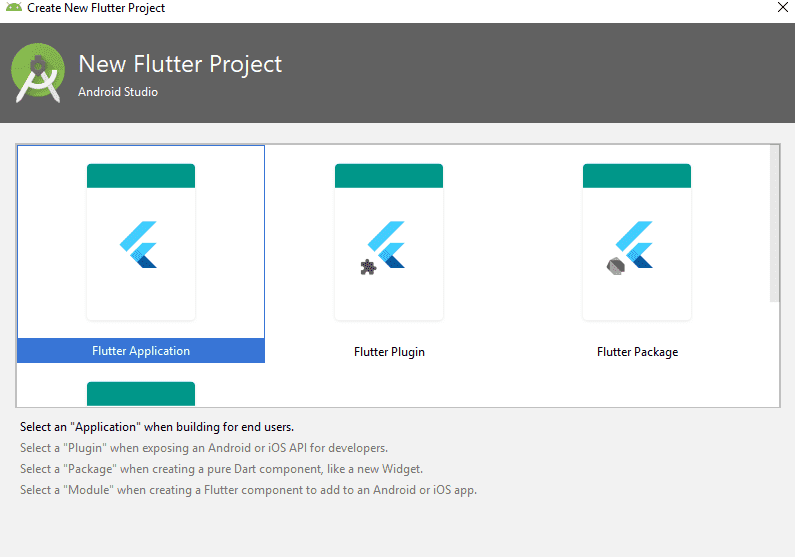
Then we will have the typical window to define the parameters of our application; the name, location, of the SDK, of the project that we are going to create and little else:
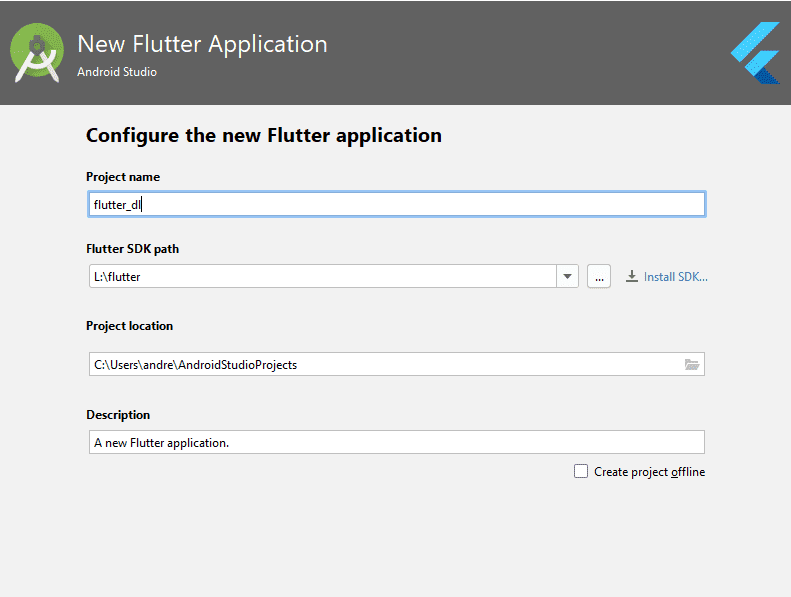
Once this is done, you hit next until Android Studio finishes configuring your project.
Once it is ready, try to run the application in a simulator, you will see the following message from Android Studio:
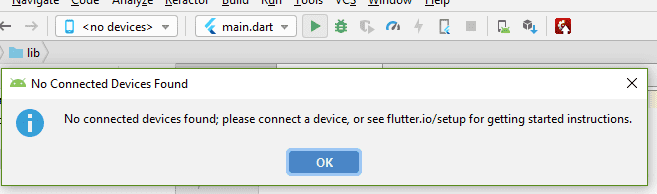
As you can see, it does not give us the option to run the Android Virtual Device or AVD for its acronym or anything, it only works if we connect an Android device to our PC which is already correctly configured as a development device; however, there is a "trick" to call it in some way to be able to use our Android Studio virtual devices.
Running our Android emulator to debug in Flutter
As we indicated, you will go to AVD Manager and then launch your Android simulator that you have selected:

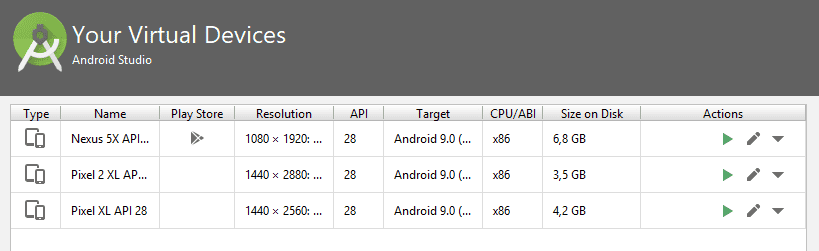
Now with this, you wait for the simulator to start and load and you can run your Android application that will be like the following:
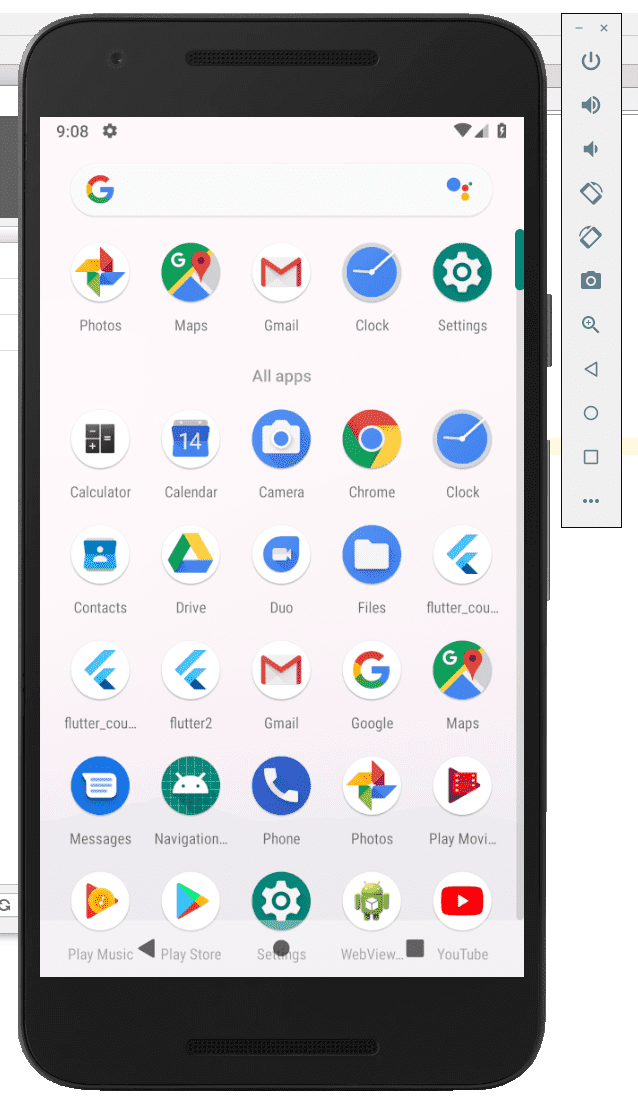
Although also remember that you can use a physical device if you wish instead of a virtual one; this is useful when you do not have a team with many features.
Widgets in Flutter for everything
Then you run the application in the simulator that you already launched in the previous steps and you will see an application like the following:
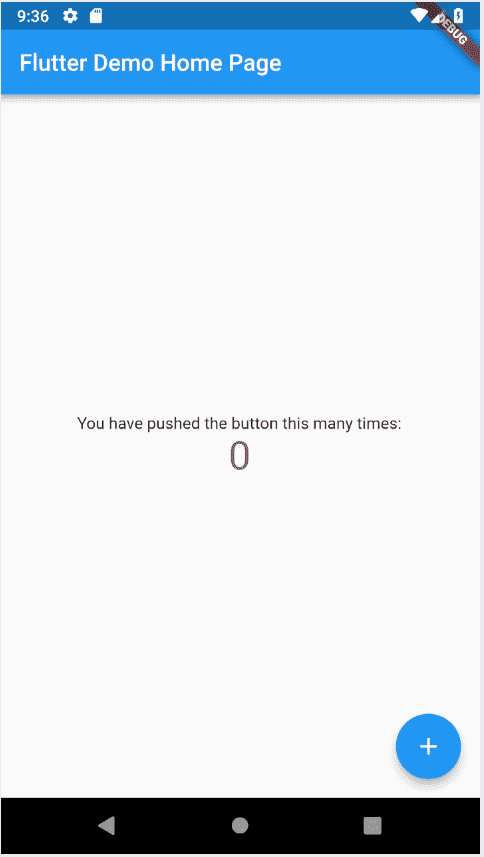
How you see is a kind of counter; the interface consists of the toolbar (1), the central body (2) and button (3) and a text (4); This is where we come in with the widget explanation; widgets are the cornerstone of Flutter application development.
All these numbers that we mentioned before are blocks that correspond to a Widget, which you can see as small applications that make up your application; and not only that, each of the 4 previous components are internally made up of more widgets; for example, in the case of the floating button, which by its nature is a widget, internally has an image component, to define the + that you see in it, which, as you can guess, is a widget; in summary; everything is a widget until the base element that can be a text or an image.
The widgets are a mechanism that allow us to develop the different components; everything in Flutter is a widget; and if EVERYTHING; if we analyze the previous image that our emulator sent us; each of the elements we highlight is a widget; there is a component for the most fundamental things we can do; even all that interface that we point out is contained in a large widget that is our application:
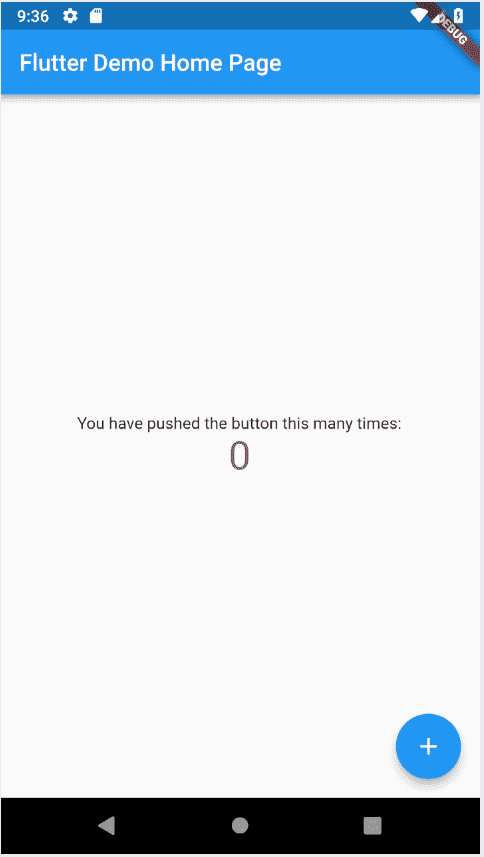
The MaterialApp to define as the base or home of our application
The first thing we are going to do is delete all the content of our main.dart which, as you can suppose, is the first file to be executed, it is the one that the Flutter SDK looks for when executing our application; it must return a special class called MaterialApp that expects as a parameter, what you can assume, a Widget, which we are going to implement next.
We are going to implement our first application in a separate class; which is the best way we have to develop new components; in other words, develop our own Widgets, that is, our application:
The first thing we are going to do is create a class in our main file, for that we use the reserved word class followed by the name of the class, which in our case will be MyFirstApp:
class MyApp
At the moment we have done very little, just define a class, but as you must already know at this point, this class needs a body, and not just any body; the classes that use visual interface components, in other words our widgets need something special, a type of the class that offers us elements that we can reuse to develop our components, and a starting point for our code (the build method); for this we must extend our class with a class that allows us to draw our widget tree!; for that we can use the StatelessWidget class, which is one of the simplest there is:
class MyApp extends StatelessWidget {
}
When we do this, the compiler will give us an error indicating that it expects something else, we must define our startup method, something similar when we are programming our applications for this platform in Android Studio we must indicate (once our appcompatactivity is defined) the methods of our life cycle of an Android app; here we must define a build method; that simple:
class MyApp extends StatelessWidget {
build(context) {
}
}
The same as once receives a parameter but we will see this later...
This is a special method where we can build our widget; It has also been our main method of our class, since at no time are we going to call it if the flutter SDK will not do it internally at the time of execution of our application.
Of course this method called build of the Flutter SDK has to do something, it has to define something interesting for our application; the first thing we are going to do is define an elementary method, which is actually a Widget, like everything here in Flutter; Said method or Widget is called MaterialApp to which we can define a home attribute so that it looks as follows:
class MyApp extends StatelessWidget {
build(context) {
home: XXX
}
}
This attribute, as you must assume, receives a Widget, which in this case will be one called Scaffold that simply paints a blank screen like the following:
class MyApp extends StatelessWidget {
build(context) {
home: Scaffold
}
}
This Scaffold Widget allows us to define the body of our application, we can define the entire screen through this container; in other words, we can define the header or the appBar and the body or body; the first thing we are going to define is the AppBar:
class MyApp extends StatelessWidget {
build(context) {
home: Scaffold(
appBar: AppBar(
title: Text("MiLista"),
),
);
}
}
As you can see, we simply define a new attribute called title which, as you can guess, receives a Widget that can be anything but the traditional text like the one we define here.
Of course, the build expects to return something, and that something must be our MaterialApp with everything we defined above:
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('MiLista'),
),
),
);
}
}
Also remember to place the main which is essential to run our application; since everything starts from here.
Now at this point you can run your application and you will see the following output:
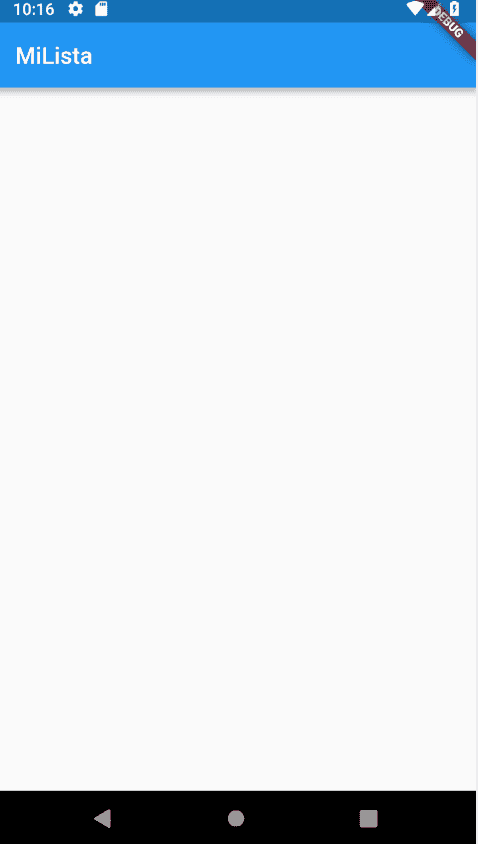
Creating a letter or card in Flutter
The next thing we are going to define will be the body of our application, which is contained in our Widget called Scaffold; We are going to create one of these cards that are so cool on Android, one of the best things brought to us by the Material Design that Google adopted with Android 5 and later called Card Views; For that we are going to use a Widget as you should already know that it is called Card:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('MiLista'),
),
),
);
}
}
How can you start realizing, everything is having more order, everything makes more sense and a Stack-type structure, where we add new components; as you probably already ventured, our Widget Card has some attributes that we can fill in, which would be the title and the image; so that you know how to work with images in Flutter from your assets folder, you can go to the last point in this article:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('MiLista'),
),
body: Card(
child: Column(
children: <Widget>[
Image.asset('assets/codeigniter.png'),
Text("Curso en CodeIgniter")
],
),
),
),
);
}
}
Here we use a new component or widget, in this case it is the one for the images; is a simple widget that specifies the image that we import into the assets folder.
In addition to this, we create a widget to be able to place a stack of widgets, for that is the Column helpers that as you can see implements a collection of widgets through an array of widgets.
Finally, when you run your app; you'll get:
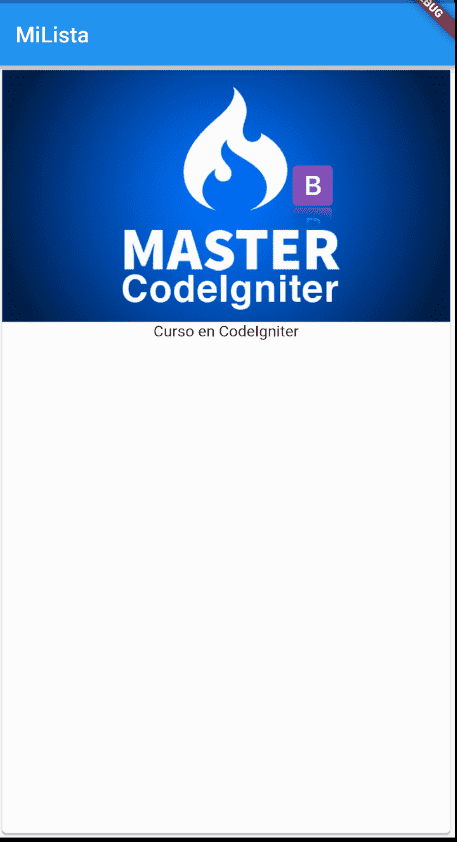
Enabling our assets folder
In order to use resources in our Flutter project, we need to go to pubspec.yaml and uncomment the following lines:
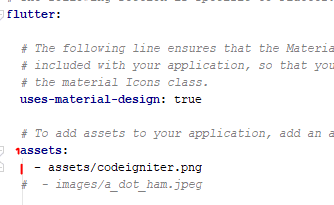
You have to be careful with the tabs since they are used since with them the name of the folder that you are going to create to throw resources in your project is defined, which in our case is called assets:
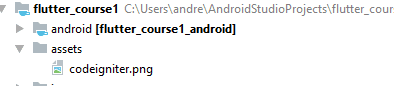
With the previous step covered, you can create a folder called assets or whatever name you defined in the root of your project and drop your image into it.
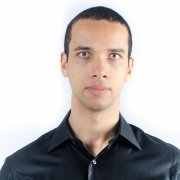
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter