Essential commands and configurations when creating any application in Django
- Andrés Cruz
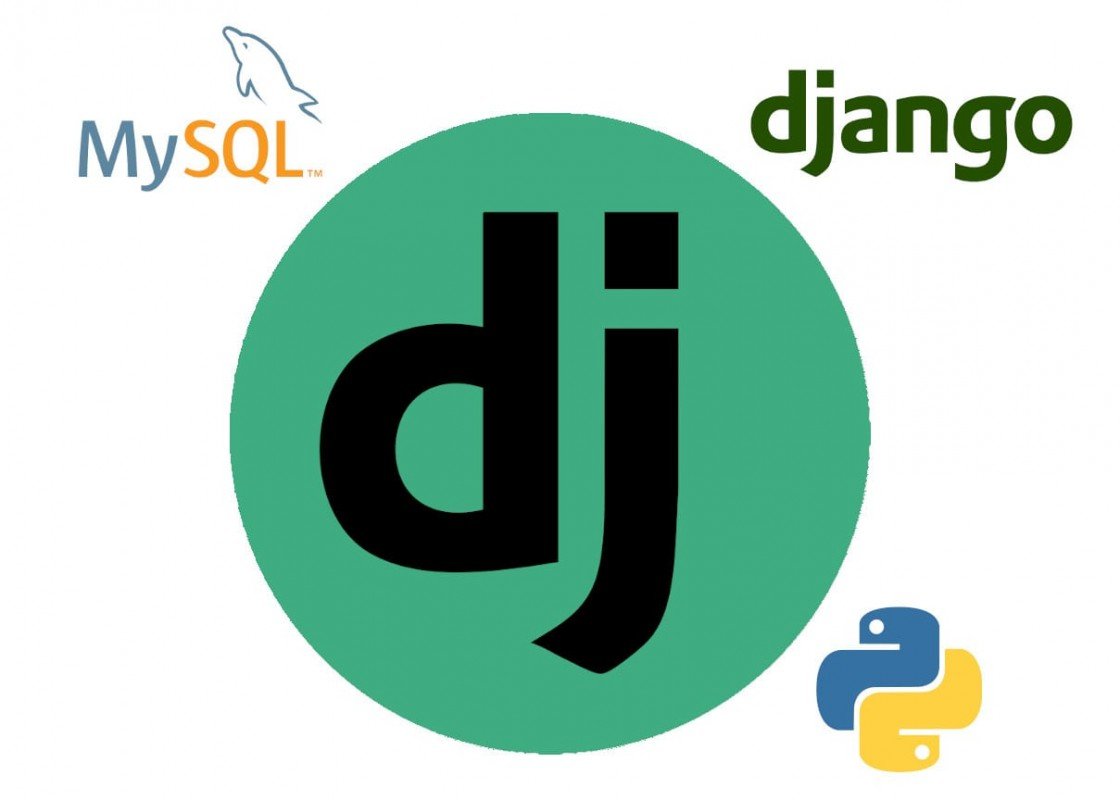
In this post I want to summarize several commands and processes that we should follow when creating an application in Django; they are very common commands and processes that we need to perform in most cases.
Create a virtual environment
To develop any application in Python in general, and Django is no exception, we must create a virtual space or virtual environment in which we can work with specific versions of our packages if necessary; for example, specific versions of Django, Djongo, and DRF; for this:
virtualenv vchannels
Where remember that vchannels is the name of the virtualenv, remember that for this you have to have the package called virtualenv installed globally on your computer, generally this should be one of the few or the only package that you install globally:
pip install virtualenv
Activate virtual space
Once we achieve the previous step, the next thing we have to do is activate our environment; for that, within the virtual environment created previously:
if you are on windows
cd Scripts
activate.bat
If you are on Mac or Linux:
source bin/activate
Instalar Django
Obviously, if we are going to develop in Django, we are going to need to install the corresponding package using pip:
pip install Django
If it were a specific version of the framework or of any package in general.
pip install Django==4.1
Create a project in Django
We already talked before about the difference between project and app in Django, and remember that the global element would be the project, which we are going to create next:
django-admin startproject mychannels
We already talked before about the difference between project and app in Django, and remember that the global element would be the project, which we are going to create next:
..\Scripts\activate
Create an app in Django
If we already have our project, the next thing we need is our application; for example, an app called chat, if you are using VSC and you made the previous installation which was to drag only the project and not the virtual space, you only have to execute the following command without having to move to another folder:
python manage.py startapp chat
Set up a database in MySQL
Let's be honest, MySQL is our database par excellence in the majority of web applications today, whether it is the Oracle version or the open source version called MariaDB, it is the database engine that we use in any web environment such as PHP, Python, Node, etc. and therefore Django cannot be the exception; the first thing we are going to do is create the configuration in settings:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'testdjangochannels',
'USER': 'root',
'PASSWORD': '',
'HOST': 'localhost',
'PORT': '3306',
}
}
You can use the connector that Django provides you by default as of version 3, but if you have problems with it, you can use a substitute called PyMySQL; we install it in our project:
pip install pymysql
And we configure it in the file called __init__.py of the module that we have inside our project with the same project name:
import pymysql
pymysql.version_info = (1, 4, 0, "final", 0)
pymysql.install_as_MySQLdb()
Very aware of placing the correct version of the connector to avoid these errors:
raise ImproperlyConfigured('mysqlclient 1.4.0 or newer is required; you have %s.' % Database.__version__)
Create a super user
As you should know at this point, Django already offers us a simple and effective administration module that is quite extensible; in order to use it, we need to create a super user:
python .\manage.py createsuperuser
It is very important to have executed the migrations of the applications that we have defined by default.
Create our first model
At this point we already have our fully functional core in our Django project, a core that you can use to develop any type of application but to extend ourselves a bit more, we are going to create a model that surely also interests you a lot; For that we have to locate any of the applications that you could have created, which in my case would only be one, the so-called chat, which this model will use to fulfill its creation purpose:
from django.contrib.auth.models import User
class Room(models.Model):
name = models.CharField(max_length=60, unique=True)
users = models.ManyToManyField(User,related_name='rooms_joined', blank=True)
def __str__(self):
return self.name
Create migrations and run migrations
With our model, we now have to tell django to create the or the migration:
python manage.py migrate
Let's remember that this only generates a file that is the one that Django is going to use to a SQL to later execute in the previously configured database; now we need to do that last step noted above; for that:
python manage.py makemigrations
Register our model in the admin to make it manageable
Already with our model and table created with or that we did previously, now we can use it, for example, in the admin application that we have by default; for that we have to locate the file called admin.py of our app and indicate that we want to use our model in the admin:
@admin.register(Room)
class RoomAdmin(admin.ModelAdmin):
pass
Create URLs
Regardless of what we want to do, surely you are going to need to consume some logic that we carry out, for that we need a routes file, practically to be able to consume anything from our MTV through the user's browser; for example, a supposed index function that should be in our venes.py of our application, we can register it in a file that by convention we will call as urls.py and that will enter our app:
path('chat/', include('chat.urls')),
from django.urls import path
from . import views
app_name = 'chat'
urlpatterns = [
path('room/<int:room_id>/', views.room, name='room'),
And now we register it globally in our project:
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('chat/', include('chat.urls')), ]
Extra: Create a CRUD
From this point we already have our base project, the skeleton of our app; therefore you can now create your magnificent application in Django that can do whatever you want, where the only limit is your imagination; for example, the already well-known CRUD in which we have several publications:
That use a master template like the following:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
{% load static %}
<link rel="stylesheet" href="{% static 'bootstrap/dist/css/bootstrap.min.css' %}">
<link rel="stylesheet" href="{% static 'css/custom.css' %}">
<link rel="stylesheet" href="{% static 'fontawesome/css/all.min.css' %}">
</head>
<body>
<div class="container my-3">
{% block content %}
{% endblock %}
</div>
<script src="{% static 'js/jquery-3.4.1.min.js' %}"></script>
<script src="{% static 'bootstrap/dist/js/bootstrap.min.js' %}"></script>
<script src="{% static 'js/store.js' %}"></script>
</body>
</html>
I agree to receive announcements of interest about this Blog.
In this post we are going to see some essential commands and configurations when creating any application in Django, from preparing the environment, configuring MySQL, creating our first model and our CRUD.
- Andrés Cruz