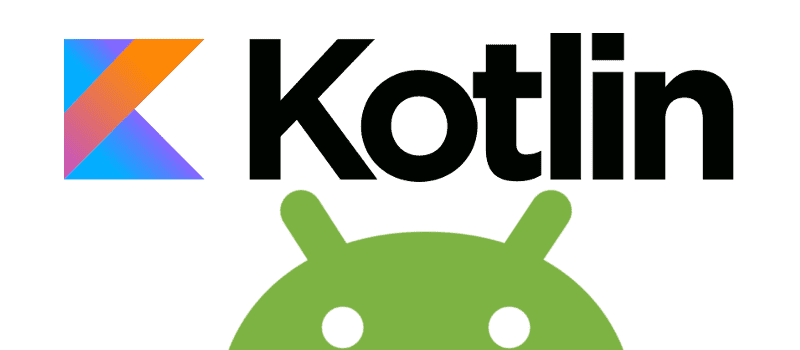
Extension functions are a powerful tool that Kotlin offers us when developing Android applications.
One way to look at it is as if we could extend classes defined either by us, by third parties or provided by Kotlin based on functions in a fast and uncomplicated way.
For example, if we wanted to extend the Int data type with a function that tells us if it is an even number:
fun Int.isEven(): Boolean = (this % 2 == 0)
With this function we are expressing in a function that extends from integer data types that checks if a number is even or not; to use it we can do the following:
println(10.isEven())
The compiler response would be true.
As we indicated at the beginning, we can also use the extension functions in other types of structures such as class; for example, taking the above Person class that we defined in the Classes in Kotlin: empty classes, constructors and properties entry:
class Persona(nombre: String, apellido: String, edad: Int) {
var nombre: String = ""
var apellido: String = ""
var edad: Int = 0
init {
this.nombre = nombre
this.apellido = apellido
this.edad = edad
}
}
We can do the following:
fun Persona.esTerceraEdad(): Boolean = edad >= 60
We can also simplify it as:
fun Persona.esTerceraEdad() = edad >= 60
var persona = Persona("Andrés"," Cruz",27)
println(persona.esTerceraEdad())
For this example, where the age is less than 60 years, return false; if, on the contrary, we create a person like:
var persona = Persona("Andrés"," Cruz",80)
The answer would be true.
Extension functions and null types in Kotlin
Note that based on the examples we are seeing above, nothing prevents us from using extension functions with null variables at any given time, this could be a problem but we can easily solve it by performing a pre-check and indicating the operator in question after Indicate the name of the class in the expression function:
fun Int?.isEven(): Boolean {
if (this != NULL) return this % 2 == 0
return false;
}
println(NULL.isEven())
The above code would return false; Let's see another example overriding the toString() method:
fun Any?.toString(): String {
if (this == NULL) return "nada"
return toString()
}
println(NULL.toString())
And this returns the text nothing.
You can get more information on how to use extension variables in the official documentation at the following link: Kotlin: Extensions.
I agree to receive announcements of interest about this Blog.
Extension functions allow you to extend functionality based on class functions defined either by us, by third parties or provided by Kotlin in a fast and uncomplicated way.
- Andrés Cruz