If you have taken my course on Vue Native, or have developed on this or React platform, you know that we can only bind styles through JavaScript and NOT with CSS, in other words we cannot establish CSS or a style sheet.
For us developers this is somewhat annoying since we LOVE having our style sheet to have a clear structure of our site; What's more, we CANNOT use technologies such as Bootstrap or Tailwind CSS in React or Vue Native.
In this post, I will address the problem of implementing global styling in a React Native app.
Here are three ways to apply global styling to a React Native or Vue app:
Method 1: Custom Components
Since both Vue and React are component-based, the most intuitive way is to create custom components (e.g. custom text fields, custom buttons, etc.), define the styles within each component, and reuse these components throughout the application.
For example, to use a specific font color throughout the app:
import React from 'react';
import { Text, StyleSheet } from 'react-native';
const BlueText = props => {
return(
<Text style={{ ...styles.blueText, ...props.style }}>{props.children}</Text>
);
};
const styles = StyleSheet.create({
blueText: {
color: 'blue'
}
});
export default BlueText;
Method 2: global style sheet
We can create a GLOBAL help file for the app where we define the style:
// https://github.com/libredesarrollo/vue-native-estilo-03/blob/main/helpers/style.js:
import { StyleSheet } from "react-native";
module.exports.style = StyleSheet.create({
container: {
width: "80%",
padding: 10,
borderWidth: 1,
borderColor: "gray",
borderRadius: 15,
marginLeft: "auto",
marginRight: "auto",
marginTop: 15,
},
h1: {
fontSize: 30,
marginBottom: 10,
},
h1: {
fontSize: 30,
marginBottom: 10
},
h2: {
fontSize: 25,
},
h3: {
fontSize: 18,
},
});
Method 3: Global style sheet
Of course, you can combine multiple styles for ONE component:
<text :style="[style.h1, , { backgroundColor: bg }, {borderColor:'yellow'}, {borderWidth: 10 }]">Index</text>
With this, we can have all kinds of styles and subtly customize them from one component to another.
Extra: Styling per component in Vue Native
We can also define a local style at the component level:
<style>
.image {
width: 100%;
height: 200px;
border-radius: 5px;
margin-bottom: 10px;
}
</style>
This is classic, if you use Vue, you can still use the 3 elements of template, script and style, the only difference is that the style is always LOCAL to the component.
Artículo recomendado
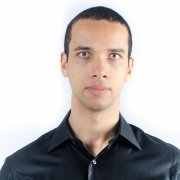
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter