We are going to explain how we can create the routing scheme for our application in Vue Native; in such a way, this is an essential feature to create practically any type of application since in most cases we need to navigate between several screens:
And with this package, we can achieve it easily.
Here the first thing you have to keep in mind is that in Vue Native we DO NOT have a main.js or index.js or in short...
The file that is responsible for creating the Vue instance and loading components as we did with Vue Router; here our startup file is called App.vue, therefore here we define our navigation scheme.
Following the official documentation, we need to install some packages globally, for me in particular the global installation did NOT work and I also needed some additional react packages for it to work correctly, which I explained in another entry that mentioned the error that occurred to me Unable to resolve module 'react-native-screen'.
So, in my project:
npm install react-native-screens react-native-reanimated react-native-gesture-handler react-native-paper
npm install vue-native-router
With this, we can create our pages or screens to navigate: we are going to create a couple in a folder that we are going to call screens:
screens/IndexScreen.vue:
<template>
<view>
<text>Index</text>
</view>
</template>
screens/DetailScreen.vue:
<template>
<view>
<text>Detail</text>
</view>
</template>
And they look like this:
And our App.vue:
Here I leave you the complete Routing project in Vue Native:
<template>
<app-navigator></app-navigator>
</template>
<script>
import {
createAppContainer,
createStackNavigator,
} from "vue-native-router";
import IndexScreen from "./screens/IndexScreen.vue";
import DetailScreen from "./screens/DetailScreen.vue";
const StackNavigator = createStackNavigator(
{
Index: IndexScreen,
Detail: DetailScreen
},
{
initialRouteName: 'Detail',
}
);
const AppNavigator = createAppContainer(StackNavigator);
export default {
components: { AppNavigator },
}
</script>
React native, used internally by Vue native
Vue native internally uses React native for each of its components, including navigation, which as you can see, is very similar to what we do with Vue Router, in which we have a component to reference the components and their routes (StackNavigator) and another to set at the app level (createAppContainer), both functions loaded from the react-native package
I leave you the source code, which is part of my COMPLETE COURSE:
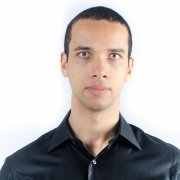
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter