To understand animations in Vue Native we have to be clear about some rules:
- We can only animate one component, the one called animated:view, we cannot animate buttons, texts... and other Vue Native or React Native components, only the one mentioned above.
Animations in Vue Native follow the same guidelines as in React Native, and this is logical, since by using Vue Native internally React Native, in the end we have a very similar use. - We animate properties, with their values, not components directly, to animate for example a size of a component, we have to use a property to vary numerical values, colors... among other accepted ones, and it is these that we establish for the animatable components .
- The animations are carried out using a simple function in which we specify the parameters to be animated, such as the duration, property to be animated among other characteristics:
timing(this.popiedadAAnimar, {
toValue: 400,
duration: 5000,
easing: Easing.linear
}
toValue: Allows us to indicate what value the property assigned to this animation will reach, the maximum or minimum limit.
duration: we indicate the duration of the animation in milliseconds, in this case 400 thousandths of a second.
easing: We specify the animation curve.
And we assign this to a component in our template:
<animated:view
class="estatica"
:style="{
height: popiedadAAnimar,
width: popiedadAAnimar,
borderRadius: popiedadAAnimar,
}"
/>
propertyAAnimate is the property that we are going to animate:
data() {
return {
popiedadAAnimar: 0,
};
},
Which we initialize:
this.popiedadAAnimar = new Animated.Value(10)
It is the equivalent of:
this.popiedadAAnimar =10
But with the new Animated.Value it is the one we have to use for the animations.
Going back to the beginning, we have the key function in all of this, which is what allows us to animate our component that looks like the following:
crecerAnimacion: function () {
Animated.timing(
this.popiedadAAnimar,
{
toValue: 400,
duration: 5000,
easing: Easing.linear,
},
{
toValue: 400,
friction: 1,
}
).start(() => {
this.popiedadAAnimar = new Animated.Value(10);
this.crecerAnimacion();
});
},
The start function is executed when the animation ends, it is a callback, which we can take advantage of to create an infinite loop for the animations.
Complete code
The following code is part of my complete course on Vue Native that you can review from the courses section:
<template>
<view class="center">
<animated:view
class="estatica"
:style="{
height: popiedadAAnimar,
width: popiedadAAnimar,
borderRadius: popiedadAAnimar,
}"
/>
</view>
</template>
<script>
import {Animated, Easing} from 'react-native'
export default {
data() {
return {
popiedadAAnimar: 0,
};
},
methods: {
crecerAnimacion: function () {
Animated.timing(
this.popiedadAAnimar,
{
toValue: 400,
duration: 5000,
easing: Easing.linear,
},
{
toValue: 400,
friction: 1,
}
).start(() => {
this.popiedadAAnimar = new Animated.Value(10);
this.crecerAnimacion();
});
},
},
created() {
this.popiedadAAnimar = new Animated.Value(0)
},
mounted() {
this.crecerAnimacion()
},
};
</script>
<style>
.estatica {
background-color: red;
align-self: center;
}
.container {
justify-content: center;
flex: 1;
}
</style>
In the end, expect something like the following:
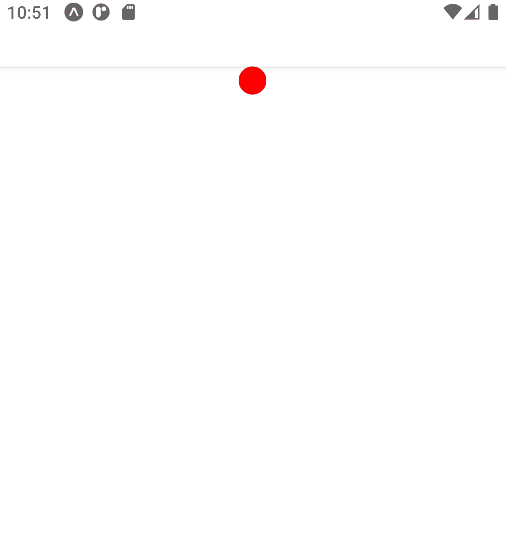
Of course, there is a lot more to see about animations in Vue Native:
- Interpolations
- Curves
- Animate colors
This with much more is part of my complete Vue Native course; You can also find more information in the official documentation.
You can see the introduction to the section of my course in which we cover animations in more detail:
- Andrés Cruz
En español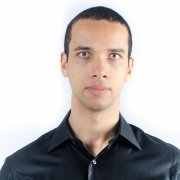
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter