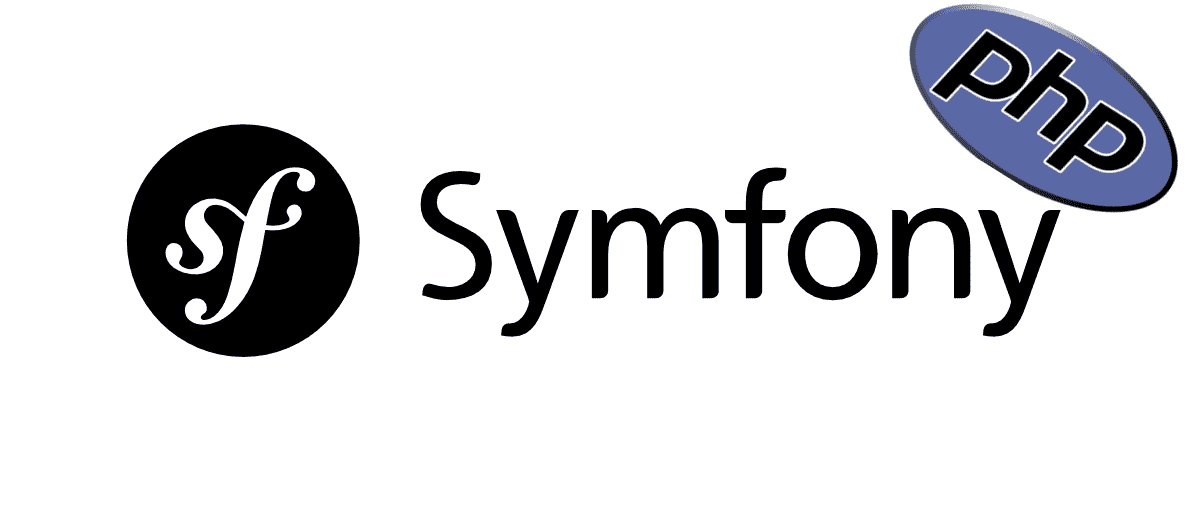
Doctrine is an object mapper better known as ORM for PHP that can be implemented in various frameworks such as CodeIgniter and Symfony; where we map a class with a relational table in the database; for example, if we have a table called Persons:
$person = new Person();
$person>name = 'Juan';
$person>surname = 'Cruz';
So in our database we have a table that contains at least two fields concerning the person's first and last name; with the previous lines of code we can create a new record in the database without making any insert or anything like that (although once the object is created and its values established, it is necessary to do a 'persist' to save it).
Ideal doctrine for complex databases
Doctrine brings us many advantages, especially when we work with moderately large systems or large systems since we do not have to use SQL statements to make all our relationships, but rather a variation of SQL called DQL (Doctrine Query Language) which brings us an object with other nested objects depending on the relationship thereof (for example, if the Persons table database contains a relationship with the Pets table where a person has one or more pets, then when making the request for ONE person record through Doctrine it will return the objects from the Pets table together with the Persons object).
Getting started with Doctrine and Symfony
In order to work with Doctrine we first have to generate the Doctrine entities which include the definition of the relational tables of our database configured at the time of project creation and also created our Bundle.
In the Doctrine entity we will find data such as foreign keys, primary keys, data types, relationships between tables, etc.; all so that Doctrine can link our database tables to the PHP classes with their getters and setters that we will define later and which we can customize to a greater extent; To generate the Doctrine entities we use:
php bin/console doctrine:mapping:import BackendBundle yml
As you can see, we must create the entities within a Bundle that we created in a previous entry:
The Bundle to modularize our projects in Symfony
Once the previous step has been completed, as many files will have been generated as there are tables in our database that have the following structure:
BackendBundle\Entity\Comments:
type: entity
table: comments
indexes:
fk_comment_video:
columns:
- video_id
fk_comment_user:
columns:
- user_id
id:
id:
type: integer
NULLable: false
options:
unsigned: false
id: true
generator:
strategy: IDENTITY
fields:
body:
type: text
NULLable: true
length: 65535
options:
fixed: false
createdAt:
type: datetime
NULLable: true
column: created_at
manyToOne:
user:
targetEntity: Users
cascade: { }
fetch: LAZY
mappedBy: NULL
inversedBy: NULL
joinColumns:
user_id:
referencedColumnName: id
orphanRemoval: false
video:
targetEntity: Videos
cascade: { }
fetch: LAZY
mappedBy: NULL
inversedBy: NULL
joinColumns:
video_id:
referencedColumnName: id
orphanRemoval: false
lifecycleCallbacks: { }

These files will be located in src/BackendBundle/Entity.
If we generate tables or relationships or simply change the database during development, just run the previous command again.
Now we generate the entities that are actually the ones that we are going to define each of the methods and variables that are mapped with the columns of the tables that they define; entities in Symfony are like models in CodeIgniter; to generate the entities we use the following command:
php bin/console doctrine:generate:entities BackendBundle
php app/console generate:doctrine:entity

At this point we are ready to start working together with the controllers, views and entities in Symfony as we will see in later posts.
I agree to receive announcements of interest about this Blog.
It explains how to generate entities through the bin/console in symfony, mapping the relationships from the database.
- Andrés Cruz