So far we have touched on several important points that you should know before venturing into the use of functions, since functions are structurally composed of all the elements that we saw previously:
Functions are pieces of code that do something, a particular task; Unlike Kotlin, where we define a function with the fun keyword, in Swift we create a function with func; that is, we put an extra 'c' to our reserved word that refers to the definition of a function in Swift:
func hola_mundo() {
}
hola_mundo()
Parameter passing in functions in Swift
func hola_mundo(name:String) {
}
hola_mundo("Mi primera función con Swift");

func hola_mundo(name:String) {
print(name)
}
(name:"Mi primera función con Swift");
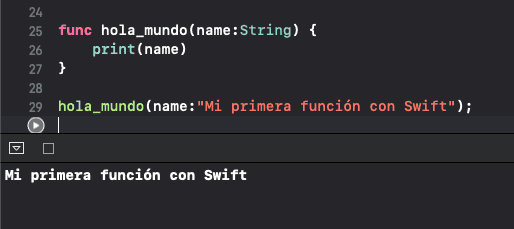
Default values for function parameters
func hola_mundo(name:String = "Andres") {
print()
}
hola_mundo()
func hola_mundo(name:String = "Andres") {
print("Hola \(name) como estas?")
}
hola_mundo()
func suma(a:Int, b:Int = 5) -> Int {
return +
}
let = 4
let =
suma(a:a,b:b)
func suma(a:Int, b:Int = 5) -> {
return a + b
}
a 4
b
print(" Suma de \(a) + \(b) = ");
I agree to receive announcements of interest about this Blog.
We explain the use of functions in Swift, parameter passing, default values, data return; fundamental to create applications for iOS.
- Andrés Cruz