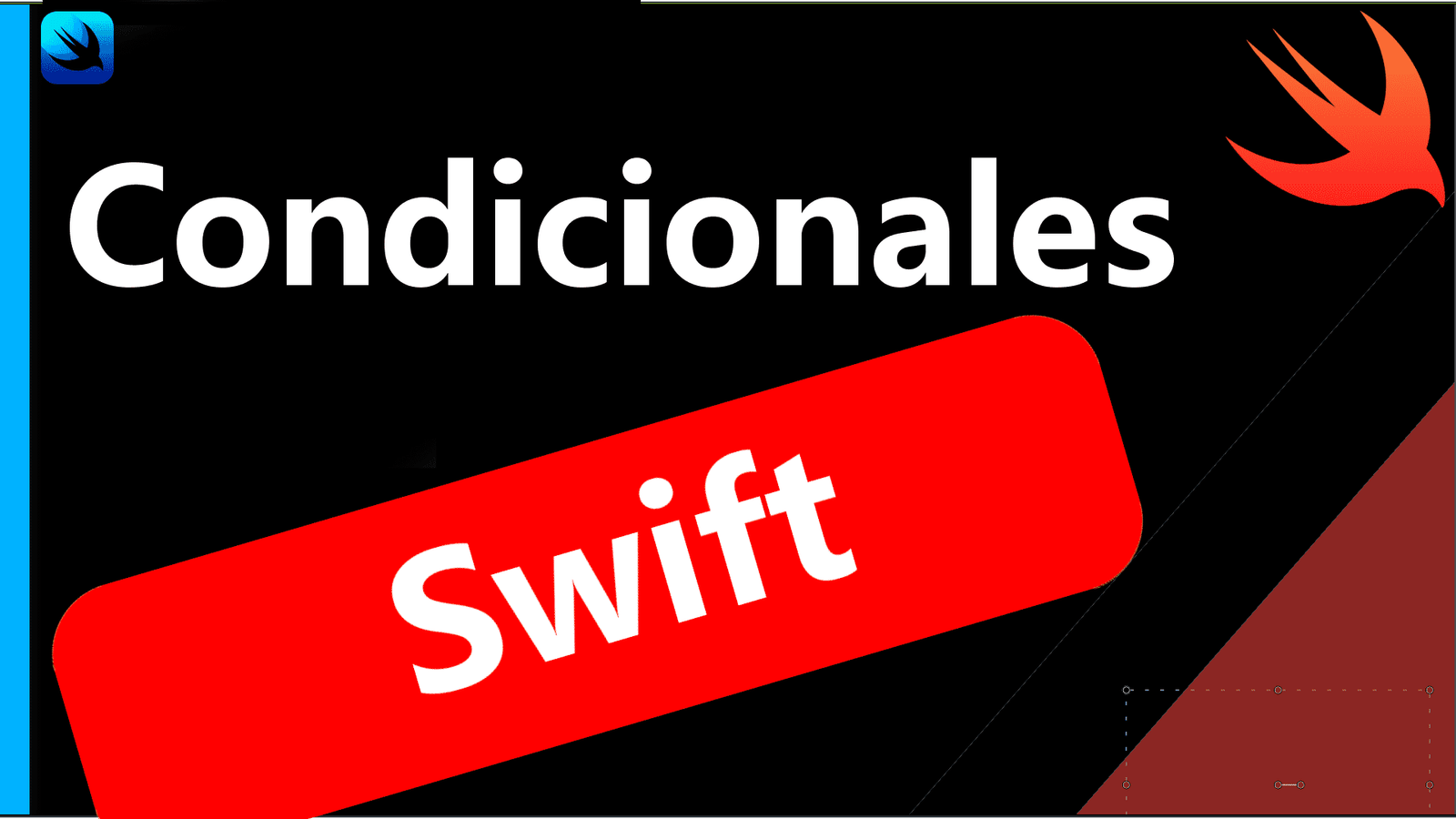
A good practice is to place the braces to encompass the code that we want to execute when the condition is met: for example, look at the following conditional:
var myVar = true
if myVar {
print("Hola Mundo")
}
And this is Swift is mandatory in any type of structure that we are going to use.
Since the condition can be read as, "if var_cond is true then..." and this is where you execute the code that defines the conditional, in the previous example you see the message on the screen since the condition is true: for To evaluate the conditions you can use any of the comparison methods that we saw previously.
You can also use if..else to execute code when the condition is false: specifically, the else is used:
var myVar = true
if myVar {
print("myVar es verdad")
} else {
print("myVar es falso")
}
Let's see some examples of how conditionals work; For example, we want to execute the following printing when the variable number is greater than 22:
var numero = 24
if numero > 22 {
print("number is greater than 22")
}
This time, when executing the code, it will show the text that says:
number is greater than 22
But if we change the value of the variable number from 24 to 14 and execute:
var numero = 14
if numero > 22 {
print("number is less than or equal to 22")
}
Nothing will appear: and we must notify our user that the value is greater than 25; for that we can use the else:
And this time the code is executed and prints that "number is less than or equal to 22".
var numero = 14
if numero > 22 {
print("número es mayor a 22")
} else {
print("número es menor o igual a 22")
}
More complete conditions: nested if and switch
Now, what happens if we want to evaluate more than one condition, or rather, we want to evaluate multiple conditions, suppose we want to evaluate if the number is equal to 10, 20, 30 and so on, this continues; We could use the following conditional:
var numero = 10
if numero == 10 {
print("número es igual a 10")
} else if numero == 20 {
print("número es igual a 20")
} else if numero == 30 {
print("número es igual a 30")
}
Although, as you can see, this can get somewhat tangled, we can use another control structure that works better for us in these cases, known as switches:
var numero = 10
switch numero {
case 10:
print("número es mayor a 10")
case 20:
print("número es mayor a 10")
case 30:
print("número es mayor a 10")
case 40:
print("número es mayor a 10")
case 50:
print("número es mayor a 10")
default:
print("número es mayor a 10")
}
And we have to give it a mandatory default case, in such a way that the switch structure must always be executed.
This way we can execute simple and more complex conditions in our code as we will see in future tutorials.
I agree to receive announcements of interest about this Blog.
We are going to learn the use of conditionals in Swift, the else, nested and the switch structure.
- Andrés Cruz