Forms are the components used par excellence to obtain data from the user. In Laravel Inertia, we can use them perfectly while maintaining the classic Laravel scheme on the server, but using Vue with Inertia from the client.
Laravel Inertia is nothing more than a scaffolding or skeleton for Laravel, which adds functionality to a Laravel project to be able to integrate Vue as client technology, with Laravel, as server technology.
In Laravel, in a controller that works with categories, we are going to create the methods to render the component in Vue, using the inertia() function instead of the classic view() function that allows rendering a component in Vue.
And the store function, to store the category data in the database; i.e. create the category:
app/Http/Controllers/Dashboard/CategoryController.php
<?php
namespace App\Http\Controllers\Dashboard;
use App\Http\Controllers\Controller;
use App\Models\Category;
use Illuminate\Http\Request;
class CategoryController extends Controller
{
public function create()
{
return inertia("Dashboard/Category/Create");
}
public function store(Request $request)
{
Category::create(
[
'title' => request('title'),
'slug' => request('slug'),
]
);
dd($request->all());
}
}
The form is created with its respective v-models and I process the submit to send the form to the previous method:
resources/js/Pages/Dashboard/Category/Create.vue
<template>
<form @submit.prevent="submit">
<label for="">Title</label>
<input type="text" v-model="form.title" />
<label for="">Slug</label>
<input type="text" v-model="form.slug" />
<button type="submit">Send</button>
</form>
</template>
For the script section, we use the useForm() function with which it allows us to manage the form in a simple way, it is a helper that facilitates the handling of errors and state of the form in general:
array:10 [
"title" => "Test"
"slug" => "test-slug"
"isDirty" => true
"errors" => []
"hasErrors" => false
"processing" => false
"progress" => null
"wasSuccessful" => false
"recentlySuccessful" => false
"__rememberable" => true
]
Finally, we have the use of the get, post, put, path or delete functions, through the Inertia object in JavaScript that we use to submit the form:
<script>
import { Inertia } from "@inertiajs/inertia";
import { useForm } from "@inertiajs/inertia-vue3";
export default {
setup() {
const form = useForm({
title: null,
slug: null,
});
function submit() {
Inertia.post(route("category.store"), form);
}
return { form, submit };
},
};
</script>
This material is part of my complete course on Laravel Inertia.
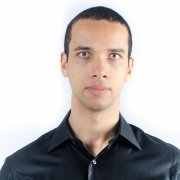
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter