Web workers allow code sections of JavaScript files to be executed in parallel in a separate thread; Generally, when creating a web application, web page, etc., all the JavaScript code is executed in a single thread created by the browser; however, when performing blocking or high-processing tasks, it is advisable to execute them outside of this thread so as not to affect this main thread.
Web workers offer a simple mechanism to run JavaScript code in the background.
In this post we will see how to work with web workers.
Getting started with web workers
As we indicated at the beginning, web workers are executed in an isolated process (in parallel); In addition, the code that we want to execute in parallel must be found in a separate file which will be downloaded asynchronously; To create our web workers we must create an object from the Worker class as follows:
var worker = new Worker('task.js');
Simply creating an object of the Worker class indicating the file to load is enough to create a web worker.
Passing messages between the main thread and the web worker
In addition to executing the JavaScript code in a separate thread, web workers offer a simple mechanism for passing messages between this separate thread or process with the main thread.
This mechanism is very simple and useful to use, where the passing of a message from the main thread triggers an event in the web worker.
To pass a message from the main thread to a Worker, use the postMessage() method:
valor = 50;
var myWorker = new Worker("tarea.js");
myWorker.postMessage(valor);
And as we saw in the previous example, this message pass actually allows us to pass data from the main thread to the Worker.
In order to see the messages that the Worker returns to the main thread, the onmessage property is used:
valor = 50;
var myWorker = new Worker("tarea.js");
myWorker.postMessage(valor);
myWorker.onmessage = function (oEvent) {
console.log("Números primos : " + oEvent.data);
};
Practical example: Worker to generate prime numbers
The following JavaScript code allows you to determine all the primes that exist from zero to a given value:
function checkPrimo(number) {
var divisor = 1;
var primo = 0;
for (var i = 0; i <= number; i++) {
if (number % i == 0) {
primo++;
}
if (primo > 2)
break;
}
if (primo == 2) {
return true;
}
else {
return false;
}
}
// funcion para determinar si un conjunto de numeros es primo
function checkPrimoCota(number) {
primos = "";
for (i = 0; i <= number; i++) {
if (checkPrimo(i))
primos += i + " ";
}
return primos;
}
And when passing a message to the web worker from our main page:
postMessage("¡Empezando a trabajar!");
onmessage = function (oEvent) {
postMessage("Recibido el siguiente valor: " + oEvent.data);
postMessage("Los siguientes son primos:" + checkPrimoCota(oEvent.data))
};
See the practical example in the download links at the beginning and end of this post.
Errors handled by Workers
As with passing messages, to handle errors you must use a property called onerror:
valor = 50;
var myWorker = new Worker("tarea.js");
myWorker.postMessage(valor);
myWorker.onmessage = function (oEvent) {
console.log("Números primos : " + oEvent.data);
};
myWorker.onerror = function (oEvent) {
console.log("A ocurrido un error en el archivo: " + oEvent.filename+" en la línea "+oEvent.lineno+" "+oEvent.message);
};
Where:
filename: Name of the script that caused the error.
message: Error caused.
lineno: Line where the error occurred.
Considerations about web workers
Web workers can NOT access:
SUN.
window object.
document object
parent object
It is necessary that the JavaScript code to be executed by the web workers are in separate files.
To close the web workers we use the close method.
To verify web worker browser support:
if(typeof(Worker) !== "undefined") {
// Soporta los Web worker
// Crear nuestros web workers
} else {
// No soporta los Web worker
}
Conclusions
Web workers can become a great tool to avoid blocking processes in our JavaScript, by executing code in parallel we can achieve better performance of our web applications.
You can see the complete example in the following links:
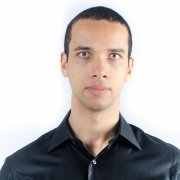
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter