Notifications are a fundamental part of any system or website, through which messages, feedback, errors, warnings or any other information about data or process states are displayed to users.
In HTML5 we can use the Notification object to configure and display informational messages to the user; the syntax to create a notification is as follows:
var notification = new Notification(titulo, {opciones})
Basic notification in JavaScript
The easiest notification to configure is the one in which only its title is present; in other words:
var notification = new Notification("Hola Mundo")
We obtain:
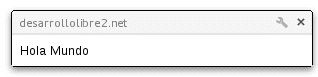
Click on the following button to launch the basic notification:
隆Lanzar notificaci贸n b谩sica!
Check notifications API support in the browser
We can use the following conditional to verify support in the browser:
if (!('Notification' in window)) {
// el navegador no soporta la API de notificaciones
alert('Su navegador no soporta la API de Notificaciones :(');
return;
}
Customizing notifications
It is possible to customize to a greater degree than simply placing a title in the notifications through the second optional parameter that allows you to configure a set of options that we will see below:
JavaScript Notification Parameters
title: Main message or title that will be displayed in the notification.
options: (optional)
- body: Text with some extra content to display.
- dir: Indicates the direction of the alignment of the notification texts, it can be:
- auto.
- ltr: align notification texts to the left:
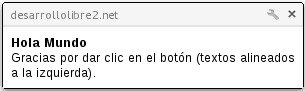
Click on the following button to launch the notification with the text aligned to the left:
- Launch notification (text on the left)!
- rtl: align notification texts to the right:
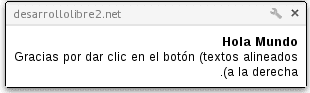
Click on the following button to launch the notification with the text aligned to the right:
- Launch notification (text on the right)!
- lang: Specifies the language used in the notification.
- tag: Notification identifier.
- icon: URL of an image to be used as an icon in the notification:
JavaScript notification permissions
It is possible that if we simply launch the notification as follows without requesting permissions:
var notification = new Notification("Hola Mundo")
It is not enough to show the notification; As with the geolocation API in HTML5, it is necessary to request permission from the user to view notifications:
The Notification.requestPermission() method to request permissions from the user...
This method is used to ask the user if they allow notifications to be displayed:

Once the response is obtained from the user, notifications can be defined and launched in the body of the method:
Notification.requestPermission() Method Syntax
Notification.requestPermission(function(permission){ var notification = new Notification("Hola Mundo"); });
Notification event handlers
Notification.onclick
Event that indicates when the user clicks on the notifications.
Notification.onshow
Event that indicates when notifications are displayed.
Notification.onerror
Event that indicates when an error occurs.
Notification.onclose
Event that indicates when notifications are closed.
The basic syntax for all the events seen above is as follows:
var notification = new Notification( "Hola Mundo");
notification.onshow = show();
notification.onerror = error();
notification.onclose = close();
notification.onclick = click();
function show() {
console.log("Se abri贸 la notificaci贸n");
}
function error() {
console.log("Ocurri贸 un error con la notificaci贸n");
}
function close() {
console.log("Se cerr贸 la notificaci贸n");
}
function click() {
console.log("Clic la notificaci贸n");
}
Notification properties
Notification.permission
indicates the current permissions granted by the user to display notifications; they may be:
- granted: User allows notifications to be shown.
- denied: The user denied the notifications.
- default: The user's decision is not known; It is likely that the user has not yet been asked for permission to show notifications.
There are also other read-only properties such as:
Notification.title
Title of the notification.
Notification.dir
Notification direction.
Notification.lang
Language used in the notification.
Notification.body
Secondary text of the notification.
Notification.tag
Notification ID.
Notification.icon
URL of the image used as the notification icon.
Final example of notifications in JavaScript
With the following JavaScript code:
function notificacionBasica() {
var notification = NULL;
if (!('Notification' in window)) {
// el navegador no soporta la API de notificaciones
alert('Su navegador no soporta la API de Notificaciones :(');
return;
} else if (Notification.permission === "granted") {
// Se puede emplear las notificaciones
notification = new Notification( "Hola Mundo");
} else if (Notification.permission !== 'denied') {
// se pregunta al usuario para emplear las notificaciones
Notification
.requestPermission(function(permission) {
if (permission === "granted") {
notification = new Notification(
"Hola Mundo");
}
});
}
}
And a button in HTML to activate the notification:
<button onclick="notificacionBasica()">隆Lanzar notificaci贸n b谩sica!</button>
We get a notification like the following:
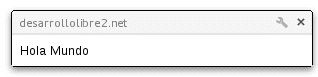
Click on the following button:
Launch basic notification!
You can find all the examples seen so far in the following links:
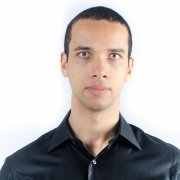
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter