Continuing to work with the audio APIs (First steps with the Soundcloud API with JavaScript) now it is the turn of the giant Google and its YouTube platform which is used for much more than playing music and watching videos of cats... YouTube has an API that is available for JavaScript from which we can have great control over the YouTube videos integrated into our website.
Include the JavaScript API in our website
The first thing we must do is include said API, which is nothing more than a JavaScript file:
<script src="http://www.youtube.com/player_api"></script>
Loading a YouTube video
Once our library is included, the next thing we have to do is load a video programmatically with JavaScript using the following JavaScript code:
function onYouTubePlayerAPIReady() {
player = new YT.Player('player', {
height: '390',
width: '640',
videoId: $($('.lista_canciones li')[index]).attr("data-href"),
events: {
'onReady': onPlayerReady,
'onStateChange': onPlayerStateChange
}
});
}
Once our library is included, the next thing we have to do is load a video programmatically with JavaScript using the following JavaScript code:
function onPlayerReady(event) {
event.target.playVideo();
}
function onPlayerStateChange(event) {
if (event.data === 0) {
//video finalizado
}
}
Playing a new video after finishing playing a video on YouTube
We will also need to include the event that will indicate each time a change is made to the video. In our case, we are interested in knowing when a video has finished playing in order to load another using the loadVideoById method that receives the identifier of a YouTube video:
function onPlayerStateChange(event) {
if (event.data === 0) {
event.target.loadVideoById({
videoId: $($('.lista_canciones li')[index]).attr("data-href")
});
}
}
With this we have the basic elements to create our own automated and personalized playlist from our website; You can check the official documentation at the following link: YouTube Player API Reference for iframe Embeds.
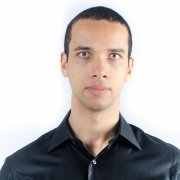
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter