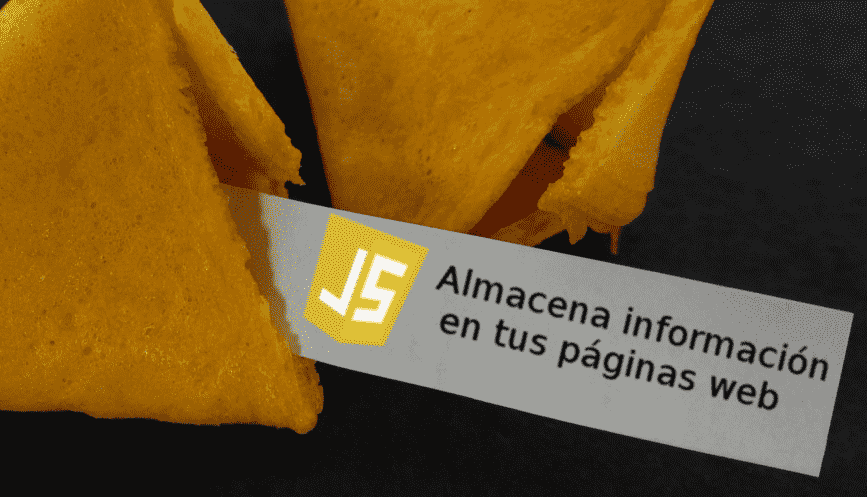
Many times we want to save some other information from our user who is visiting a web page but for various reasons we do not want to use server-side technologies.
By using other technologies such as Android, desktop applications or any application on the server side, the answer when wanting to save information persistently in files and/or databases is easier; with JavaScript we have some similar solutions like Cookies.
Cookies are small files that are stored on web pages.
JavaScript has several persistent technologies (that the information we want to store is maintained even when the user closes or refreshes the browser window) such as SQLite and Cookies that we will discuss in this post.
Cookies in JavaScript
Surely you have heard multiple times about cookies when entering a website a message like the following appears: "This site, like most, uses cookies. If you continue browsing we understand that you accept the cookie policy."
But maybe you haven't paid much attention to it yet, but don't worry; this post provides an introduction to the use of Cookies with JavaScript.
Getting started with Cookies with native JavaScript
Creating Cookies
To create Cookies we must use the key=value or key=value scheme:
document.cookie="key=value;"
Accessing Cookies
To access all Cookies from the current position we can use the following JavaScript:
document.cookie;
For our example (which we will see a little later) it gives something like the following result:
"nombre=andres; usuario=acy;"
Cookie Examples
We will create a couple of Cookies to experiment with; the first Cookie indicates the name of the person and another Cookie to indicate the user which will be requested from the user through a JavaScript prompt:
// ejemplo tomado en gran parte de http://www.w3schools.com/js/js_cookies.asp
function setCookie(cname, cvalue) {
document.cookie = cname + "=" + cvalue + "; ";
}
function getCookie(cname) {
var name = cname + "=";
var ca = document.cookie.split(';');
for (var i = 0; i < ca.length; i++) {
var c = ca[i];
while (c.charAt(0) == ' ')
c = c.substring(1);
if (c.indexOf(name) == 0)
return c.substring(name.length, c.length);
}
return "";
}
function checkCookie() {
var nombre = getCookie("nombre");
var usuario = getCookie("usuario");
if (nombre != "" && usuario != "") {
alert("Hola " + nombre + " tu usuario es: " + usuario);
} else {
nombre = prompt("Da tu nombre:", "");
if (nombre != "" && nombre != NULL) {
setCookie("nombre", nombre);
}
usuario = prompt("Da tu usuario:", "");
if (usuario != "" && usuario != NULL) {
setCookie("usuario", usuario);
alert("refresca la página");
}
}
}
checkCookie();
What you should try in the example above is to supply your name and a username and refresh the page.
Once the previous steps have been carried out, you will see that the browser "remembers" the name previously placed and the user; With this small example you can demonstrate the basic use of cookies and from here explore a wide number of new possibilities to place our web applications on another level.
Cookie expiration date
Cookies can be defined a useful life so that once the date passes they are simply deleted automatically:
document.cookie="nombre=andres; expires=Thu, 17 Dec 2016 12:00:00 UTC";
For this we can use a scheme like the one presented in the W3C:
function setCookie(cname, cvalue, exdays) {
var d = new Date();
d.setTime(d.getTime() + (exdays*24*60*60*1000));
var expires = "expires="+d.toUTCString();
document.cookie = cname + "=" + cvalue + "; " + expires;
}
We specify the number of days we want our Cookie to last (with the exdays variable) and calculate the time from the current time d.getTime(), then it remains to specify the appropriate format:
var expires = "expires="+d.toUTCString();
Ending the Cookie CRUD
So far we have seen how to create, access and specify the lifetime of the Cookies, it remains to indicate how we can delete and modify the existing ones.
Deleting Cookies.
In the previous section we indicated how to define a lifetime for Cookies; we can use a date before the current one to delete Cookies that are no longer useful to us:
document.cookie="nombre=andres expires=Thu, 16 Dec 2015 12:00:00 UTC";
Modifying Cookies.
Cookies are modified as they are created; that is to say:
document.cookie="nombre=andres";
Final Example of Cookies
To finish, let's see an example where we use everything explained so far.
>!--Demas JavaScript del ejemplo anterior-->
function deleteCookie() {
document.cookie = "nombre=; expires=Thu, 01 Jan 1970 00:00:00 UTC";
document.cookie = "usuario=; expires=Thu, 01 Jan 1970 00:00:00 UTC";
}
function updateNombre() {
nombre = prompt("Da tu nombre nuevamente:", "");
if (nombre != "" && nombre != NULL) {
setCookie("nombre", nombre);
}
}
function updateUsuario() {
usuario = prompt("Da tu usuario nuevamente:", "");
if (usuario != "" && usuario != NULL) {
setCookie("usuario", usuario);
}
}
Links of interest
I agree to receive announcements of interest about this Blog.
JavaScript has several persistent technologies (that the information we want to store is maintained even when the user closes or refreshes the browser window) such as SQLite and Cookies that we will discuss in this post.
- Andrés Cruz