In this post we will see how to use the animation library for JavaScript called anime.js; In general, it is a very easy to use library that will remind us a lot of how animations and transitions work in CSS.
The advantage we have is the possibility of creating dynamic animations that can depend on many scenarios that we can easily handle with JavaScript and without the need to add/remove classes from time to time to vary the behavior of any animable element; besides being more extensible which brings us more possibilities of use.
Downloading the animation library for JavaScript
You can download the animation library at the following link: anime.js on Github; to use the library, we simply add the following link:
<script src="anime.min.js"></script>
Or for the compressed version:
<script src="anime.js"></script>
For the development version; If you want to see more examples or the official documentation, you can consult the following link: anime.js.
Making an example animation
There are many examples which you can find in the following link: anime.js experiments but to start and see the versatility of this library we will work with a modified version of the following experiment anime.js stress test which is quite simple but quite attractive the final effects that are achieved in sight as we will see below.
As we indicated before, the experiment is quite simple to carry out, we only need a bit of CSS and JavaScript as we can see in the following three component parts:
Animated Experiment CSS
Define the CSS for the container that will contain (worth the redundancy) each one of the tables that we will define previously; For that, a box with the following dimensions will be created:
section {
width: 400px;
height: 400px;
}
And each of the colored boxes that will be contained within the section defined above:
div {
display: inline-block;
width: 20px;
height: 20px;
}
Generating the HTML by JavaScript
Now we must create the maximum amount of divs within the section; performing a simple mathematical operation, we realize that:
(400*400)/(20*20) = 400
We need about 400 squares/div to completely fill our section, which we obviously won't do by hand unless you're really bored; to do this we use the following JavaScript:
var maxElements = 400;
var colors = ['#FF324A', '#31FFA6', '#206EFF', '#FFFF99'];
var createElements = (function() {
var sectionEl = document.createElement('section');
for (var i = 0; i < maxElements; i++) {
var el = document.createElement('div');
el.style.background = colors[anime.random(0, 3)];
sectionEl.appendChild(el);
}
document.body.appendChild(sectionEl);
})();
Defining the animation with anime.js
Now came the time for magic; we are going to use the anime.js library to create an animation that is composed of the following code:
anime({
targets: 'div',
translateX: function() { return anime.random(-6, 6) + 'rem'; },
translateY: function() { return anime.random(-6, 6) + 'rem'; },
scale: function() { return anime.random(10, 20) / 10; },
rotate: function() { return anime.random(-360, 360); },
delay: function() { return 400 + anime.random(0, 500); },
duration: function() { return anime.random(1000, 2000); },
direction: 'alternate',
loop: true
});
As we can see, we define a series of parameters made up of random or random numbers in a range that we can customize as we want; We define the translation in each of the 2D axes with translateX and translateY as well as the scaling with scale, the delay of the animation delay, rotate rotate, duration duration and we indicate that the animation runs infinitely loop and a very important parameter that is the direction with the alternate value that allows you to rebuild the scene after the animation is finished (sort of like performing the animation but in reverse when it comes to an end).
With this we get:
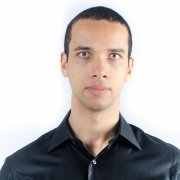
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter