Widget onWillPop: Intercept when clicking the back button in Flutter to go to the previous page
- Andrés Cruz
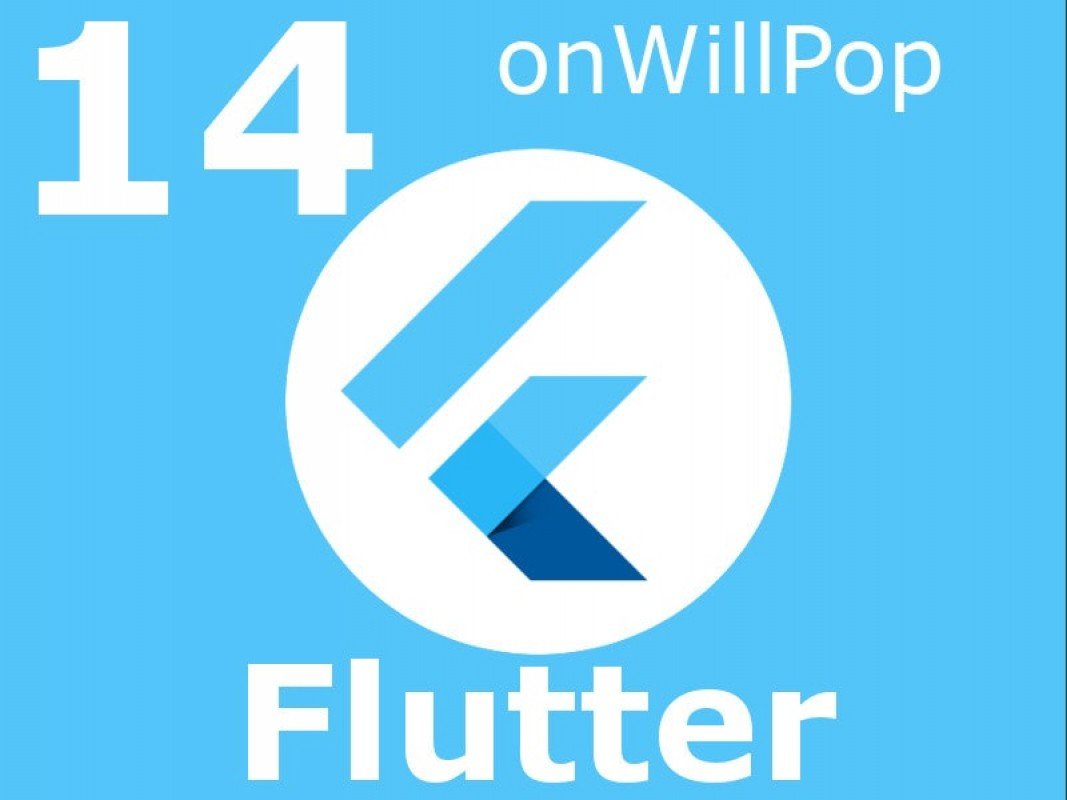
WillPopScope is a widget in Flutter that allows you to control the behavior when a user presses the back button on a mobile device. It's especially useful when you want to perform a custom action instead of just closing the app entirely. For example, you can use WillPopScope to display a confirmation dialog before the user leaves a screen or to perform some other specific action.
When using the WillPopScope widget, you must provide a parameter onWillPop which is a function that returns a Future<bool>. This method is called automatically when the user presses the back button. If the function returns true, the app will be closed, while if it returns false, the navigation will stop and the app will not be closed.
For example, you could use WillPopScope to display a confirmation dialog before the user leaves a screen. Here's an example of how this might look in code:
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Mi pantalla"),
),
body: WillPopScope(
onWillPop: () async {
bool confirm = await showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text("¿Desea salir de esta pantalla?"),
actions: <Widget>[
FlatButton(
onPressed: () => Navigator.of(context).pop(false),
child: Text("No"),
),
FlatButton(
onPressed: () => Navigator.of(context).pop(true),
child: Text("Sí"),
),
],
);
},
);
return confirm;
},
child: Text("Contenido de mi pantalla..."),
),
);
}
In this example, the onWillPop method displays a confirmation dialog with two buttons. If the user selects "No", the dialog closes and the application remains on the same screen. If the user selects "Yes", the dialog closes and the application returns to the previous screen. Whenever a boolean value is returned from the onWillPop method, the WillPopScope will make the decision to navigate or close the application.
When we go back from the route to return to the previous page, in Flutter it is likely that you need to show some previous warning to prevent the user from losing some important information there that the user was working on. This is very easy to do in Flutter using a widget that allows us to intercept this type of behavior; it is known as WillPopScope and works as follows.
WillPopScope widget in practice
Using this widget is very simple, we only have to place it at the top level in our widget tree and we indicate the one we had at the top level directly in the child property of this widget.
Before WillPopScope:
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Guardar"),
),
body: Container(
child: _buildForm(note),
),
);
}
After of WillPopScope:
@override
Widget build(BuildContext context) {
return WillPopScope(
onWillPop: _onWillPopScope,
child: Scaffold(
appBar: AppBar(
title: Text("Guardar"),
),
body: Container(
child: _buildForm(note),
),
),
);
}
As you can see, we simply embed the top or parent widget of our page with the WillPopScope and that's it; this code snippet is part of my free Flutter course on YouTube:
onWillPop property to define the behavior when doing the back
Finally, we have to define another property, which is actually a function which returns a Boolean Future.
This is great since we can use it to do what we're going to do with this widget in 99.9 percent of the cases which would be, build a Dialog:
WillPopScope(onWillPop: _onWillPopScope, child: _buildForm(note)),
The _buildForm function can be anything that returns a widget or widget tree; basically it would be our page in Flutter; In our case it is a form following the development we do in our Flutter mini course on YouTube.
Future<bool> _onWillPopScope() {
return showDialog<bool>(
context: context,
child: AlertDialog(
title: Text("¿Seguro que quieres regresar a la página anterior?"),
content: Text('Tiene data sin guardar'),
actions: [
FlatButton(
onPressed: () => Navigator.of(context).pop(false), child: Text("No"),),
FlatButton(
onPressed: () => Navigator.of(context).pop(true), child: Text("Si"),)
],
));
}
}
There we build an example dialog, which will be displayed when we click either on the bar or on the back button:
The most interesting thing about this is that we create a process that will be executed when we try to drop the back in an app in Flutter; either through the Android back button or through the icon in the toolbar.
I agree to receive announcements of interest about this Blog.
We explain how to work with the onWillPop widget to intercept the user's action when going back through the toolbar, back button or any other method and display any type of widget.
- Andrés Cruz