Widgets:
Widget is a way to declare the user interface in Flutter.
Flutter provides a huge number of widgets that help us build applications in a modular way, as if it were lego pieces. The main idea is that you create your user interface from widgets. The widgets describe how the view or screen of your application should be according to its organization and properties.
Scroll bar:
One of the fundamental elements when developing any type of applications and especially mobile ones due to the space available, is the scroll or the scroll bar.
To add a scroll bar to a ScrollView, wrap the scroll view widget into a scroll bar widget. Using this widget we can scroll through a widget.
The scroll bar allows the user to skip or scroll to a part of the interface when it cannot be fully displayed on the screen at one time.
Constructor:
We are going to create a scroll bar that by default will allow the scroll to be applied to its child element.
Scrollbar( {Key? key, required Widget child, ScrollController? controller, bool? isAlwaysShown,bool? showTrackOnHover, double? hoverThickness,double? thickness, Radius? radius, ScrollNotificationPredicate? notificationPredicate} ),
Code Implementation:
In this code snippet, we use a widget of type Listview and wrap it in the scrollbar. In this, isAlwaysShown is a bool indicating that the scrollbar should be visible, even when scrolling is not in progress, and showTrackOnHover controls whether the bar will be shown on scroll or always hidden.
But remember that you can use any type of widget that you know will not fit on the screen.
Expanded(
child: Scrollbar(
isAlwaysShown: _isAlwaysShown,
showTrackOnHover: _showTrackOnHover,
child: ListView.builder(
itemCount: 20,
itemBuilder: (context, index) => MyItem(index),
),
),
),
We'll add the listview.builder() method that will allow us to demonstratively make or create some items so that they don't fit on the screen and the scroll bar appears.
Finally, the entire code:
import 'package:flutter/material.dart';
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
bool _isAlwaysShown = true;
bool _showTrackOnHover = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Scrollbar Demo'),
),
body: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Expanded(
child: Scrollbar(
isAlwaysShown: _isAlwaysShown,
showTrackOnHover: _showTrackOnHover,
hoverThickness: 30.0,
child: ListView.builder(
itemCount: 20,
itemBuilder: (context, index) => MyItem(index),
),
),
),
Divider(height: 1),
],
),
);
}
}
class MyItem extends StatelessWidget {
final int index;
const MyItem(this.index);
@override
Widget build(BuildContext context) {
final color = Colors.primaries[index % Colors.primaries.length];
final hexRgb = color.shade500.toString().substring(10, 16).toUpperCase();
return ListTile(
contentPadding: EdgeInsets.symmetric(horizontal: 20, vertical: 20),
leading: AspectRatio(
aspectRatio: 1,
child: Container(
color: color,
)),
title: Text('Material Color #${index + 1}'),
subtitle: Text('#$hexRgb'),
);
}
}
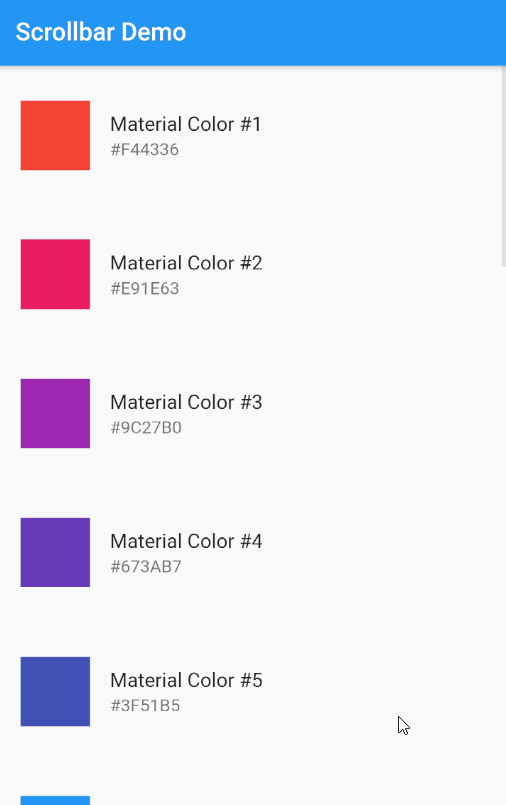
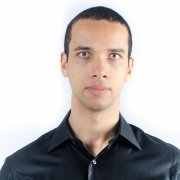
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter