Many times it is necessary to display HTML content in a Flutter application, a good example of this I can mention to you myself, I have been creating an application in Flutter for this blog, which includes the Academia app, but returning to the blog, to the be HTML content; What you are reading is HTML content generated by a WYSIWYG JavaScript plugin and that through a Rest Api can be consumed from the app in Flutter, so I need some way to present this HTML content through a webview or something similar.
In this post we will see a plugin that allows you to display HTML content in Flutter using a plugin as there is no solution that is part of the core of Flutter.
If you are building an application in Flutter and need to render HTML content, you can use the flutter_html package. This package allows you to display HTML content inside your Flutter widgets. Below I explain how to do it:
Install:
Add flutter_html to the dependencies of your pubspec.yaml file by running the following command:
flutter pub add flutter_html
Then get the dependencies with:
flutter pub get
Use:
Import the package into your code file:
import 'package:flutter_html/flutter_html.dart'; import 'package:flutter_html/style.dart'; // Para usar estilos CSS
Use the Html widget to render HTML content:
Dart
Html( data: /* Your HTML source */, style: { /* CSS styles (not real CSS) */ 'h1': Style(color: Colors.red), 'p': Style(color: Colors.black87, fontSize: FontSize.medium), 'ul': Style(margin: EdgeInsets.symmetric(vertical: 20)), }, )
In the example above, you can provide your own HTML instead of /* Your HTML source */. Styles are applied using a map where the keys are the names of the HTML tags and the values are Style objects.
Complete Example:
Here is a complete example of how to use flutter_html:
import 'package:flutter/material.dart'; import 'package:flutter_html/flutter_html.dart'; import 'package:flutter_html/style.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return const MaterialApp( debugShowCheckedModeBanner: false, title: 'Mi App con HTML', home: HomePage(), ); } } class HomePage extends StatelessWidget { final _htmlContent = """ <div> <h1>This is a title</h1> <p>This is a <strong>paragraph</strong>.</p> <p>I like <i>dogs</i></p> <ul> <li>Elemento de lista 1</li> <li>Elemento de lista 2</li> <li>Elemento de lista 3</li> </ul> <img src='https://www.kindacode.com/wp-content/uploads/2020/11/my-dog.jpg' /> </div> """; const HomePage({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: const Text('Mi App con HTML')), body: SafeArea( child: SingleChildScrollView( child: Html( data: _htmlContent, style: { 'h1': Style(color: Colors.red), 'p': Style(color: Colors.black87, fontSize: FontSize.medium), 'ul': Style(margin: EdgeInsets.symmetric(vertical: 20)), }, ), ), ), ); } }
In this example, the HTML content is shown with custom styles. The part that allows us to customize the CSS of the rendered HTML is very interesting, with which we can show a style customized by us and not the default style in HTML.
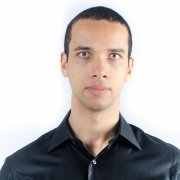
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter