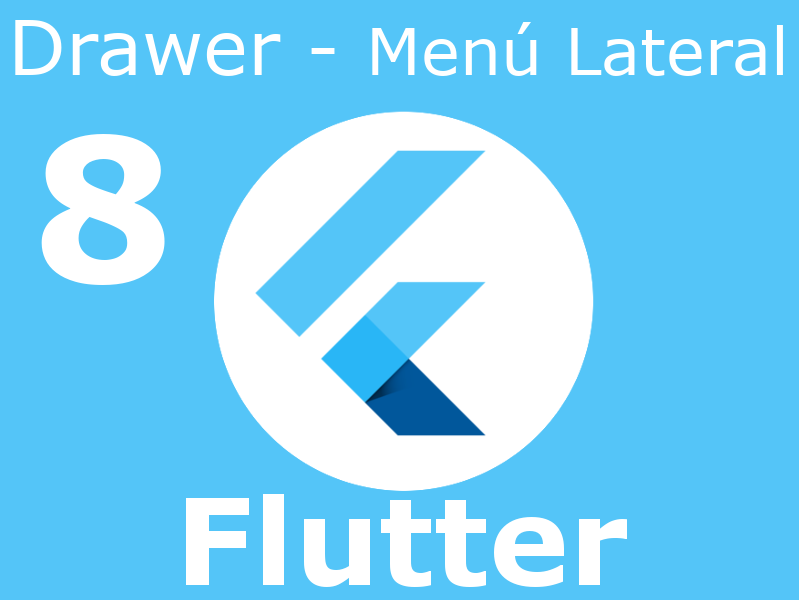
The navigation drawer usually opens from the left side of the screen, but you can also set it to open from the right side and take up 70 percent of the screen, and to close it, you can simply swipe or click out of the drawer.
What is a side menu or Drawer and what is it for?
A side menu is a UI element that is displayed on the side of an app and that displays navigation options or secondary actions. This type of UI element is widely used in the context of apps in Flutter or Material Design and Android. in general. It can be opened by swiping or touching a button or icon, and it scrolls through the main content of the app to reveal additional options. Users can interact with menu options to access different views or actions; It is widely used for navigation between different pages.
A side menu or Navigation Drawer is simply a navigation panel that is displayed from the left or right side, generally by pressing a hamburger-type button from the AppBar or Toolbar and that of course we can implement in our application in Flutter.
The objective of this menu is to present a set of options to our user that consists of functionalities that the application allows.
How to create a side menu?
Using Dart specifically the Flutter framework we can easily create it in our applications using a widget that allows us to create said component and we can easily bind it to a Scaffold through a property called drawer.
Creating the Drawer
Here you have to start with the typical, create a clean project in Flutter with Android Studio or Visual Studio Code, remove the initial code and create a Scaffold:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter NavBar',
),
home: Scaffold(
appBar: AppBar(title: Text("Menu Lateral"),),
drawer: CustomDrawer.getDrawer(),
)
);
}
}
We are going to know how we can create a Drawer or side menu in Flutter just like we did in Android in a previous entry in which we created a side menu in Android; we are going to give the entire implementation of the code and then we will analyze the code in detail:
Remember that a side menu is just a vertical panel in which we can place a collection of elements that in our case would be a collection of items one stacked below another; therefore the Column is perfect for us here to stack a multitude of widgets for our header and options:
class DrawerItem {
String title;
IconData icon;
DrawerItem(this.title, this.icon);
}
class CustomDrawer {
static int selectedDrawerIndex = 1;
static final _drawerItems = [
DrawerItem("Perfil", Icons.person),
DrawerItem("Ver eventos", Icons.access_alarm),
DrawerItem("Crear eventos", Icons.add_alarm),
DrawerItem("Ver consejos", Icons.web),
DrawerItem("Aviso legal", Icons.info)
];
static _onTapDrawer(int itemPos, BuildContext context){
Navigator.pop(context); // cerramos el drawer
selectedDrawerIndex = itemPos;
}
static Widget getDrawer(BuildContext context) {
final prefs = new UserPreferences();
List<Widget> drawerOptions = [];
// armamos los items del menu
for (var i = 0; i < _drawerItems.length; i++) {
var d = _drawerItems[i];
drawerOptions.add(new ListTile(
leading: new Icon(d.icon),
title: new Text(d.title),
selected: i == selectedDrawerIndex,
onTap: () => _onTapDrawer(i, context),
));
}
// menu lateral
return Drawer(
child: Column(
children: <Widget>[
UserAccountsDrawerHeader(
accountName: Text(prefs.name), accountEmail: Text(prefs.email)),
Column(children: drawerOptions)
],
),
);
}
}
In general terms, before going into detail about all the code, the app was created to be consumed using static methods; that is, this is a separate class that you can consume from your Flutter pages through the getDrawer() method, just as we do in our course:
Items para el menú lateral
To facilitate the process of creating the items or the options of the side menu and to have everything better organized, we create an auxiliary class called _DrawerItem with the elements of which our menu item will consist; for our example, it has the usual, a title or name for the item and an associated icon, but you could customize it further and add other elements such as color; as you can see, this class is private, the reason is that we are only interested in using it in the class that is in charge of defining the vertical panel or side menu.
And from the code above you can also see that we have an array with the menu items that use the class that we defined and explained above.
But we cannot process an array of classes as such, remember that everything in Flutter is a widget and a side menu is no exception, so you could bring this list that we have defined as base or hardcode in our build function, for example from a Rest Api.
And we pass this list to a List of widgets of type ListTile that we will use later.
Map the list of options objects to a list of widgets
The next thing we have in the list is to map our list, that is, we have a list of objects, but drawerItems but we need is a list of widgets; there are many ways in which we can do this, but the most interesting is to use the map method that allows us to map from one type of data to another; as you can see in the implementation, we change from a list of objects to a list of widgets; the interesting point here is that the map function implements an anonymous function in which we can do any kind of operation to convert our initial item to another type of object, which in this case would be a widget that in the end will be our list of widgets. ; since the map function can be assigned to another object and then we call the toList() method to go from a map to a list.
Create the Side Menu: Drawer in Flutter
Header or header of the side menu
Now, with the basic data defined and ready to use, we create our side menu, for that we make use of the Scaffold as the root element of our app and its property called drawer in which we can pass a widget that will be our Drawer side menu; This side menu receives a child as usual in this type of component and then we define a Column, because we are going to define a list of elements or widgets; The first thing we will place will be a UserAccountsDrawerHeader, which is a widget to define a header, as easy as the famous header to define elements such as the profile image, username or account, email, among other aspects that you can review.
Tap event and navigation
After the Drawer header, we pass our list of options, it's that simple, we have everything we did in the previous block in a function that we pass from one to our side menu and that's it; As you can see, each menu item can implement a tap event which we have defined by passing the index of the tapped item; and from here comes the ListTile widget that allows us to define texts, icons and the tap event.
Another optional widget in this implementation would be the ConstrainedBox that allows us to define a box with maximum or minimum dimensions, it is a kind of responsive container with extremes; You can look for more information about it in the official documentation.
Another important point that we have not yet seen and that we are going to discuss in another entry is navigation between screens, which is a fundamental issue and without it, the options on our side menu will be of little use.
But with this we have our side menu implemented and ready to do whatever you want to do.
I agree to receive announcements of interest about this Blog.
We are going to know how we can create a side menu or Drawer with options, headers and user information in Flutter.
- Andrés Cruz