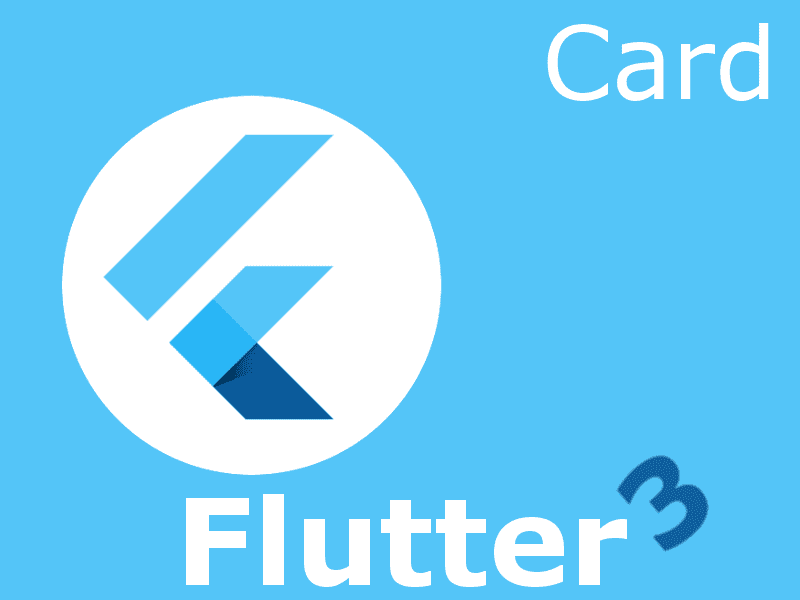
The Card widget in Flutter is a visual component used to display information on a card or chart; It is a generally white grid with rounded edges and shading. This card can be customized with different elements to highlight the content such as images, text, buttons and forms among others. The Card widget is commonly used in mobile and web applications to present information in a clear and organized manner.
The Cards in Flutter are another fundamental component in any application that works with Material Design and this is no exception; Card Views are a fundamental component in Android since its inception, which adopted Material Design as a design rule and of course we can use Flutter as yet another widget.
Cards are used to display information on a letter or card, it's that simple; they are simply container components with rounded corners and shading; It's that simple, in it we can add texts, images or any other container widget as columns to add multiple elements or simply add only one widget; We can also use the Center type widget as well as any widget in general, but these could be its most common configurations.
To create a Card in Flutter, nothing is easier, we simply have to add the following lines:
Card()
But with this, as you can suppose, we have practically nothing, we need more elements, more widgets with which we can take advantage of the Flutter Cards.
Define images in our Card widget
Although with this we have practically nothing, since an empty container is of no use to us; we can add all the elements we want, or any other widget through the child property, but we can ONLY add ONE WIDGET, and nothing else:
Card(
child: Center(
child: MaterialButton(
minWidth: 200.0,
height: 40.0,
onPressed: () {},
color: Colors.lightBlue,
child: Text('Material Button',
style: TextStyle(color: Colors.white)),
),
),
),
As you can see, here we have several important properties, to indicate the size through the width and height, in addition to the fact that we are adding a button with a text as we saw in the previous entry on Button widgets in Flutter: Raised, Flat, Material, Icon and Floating Action
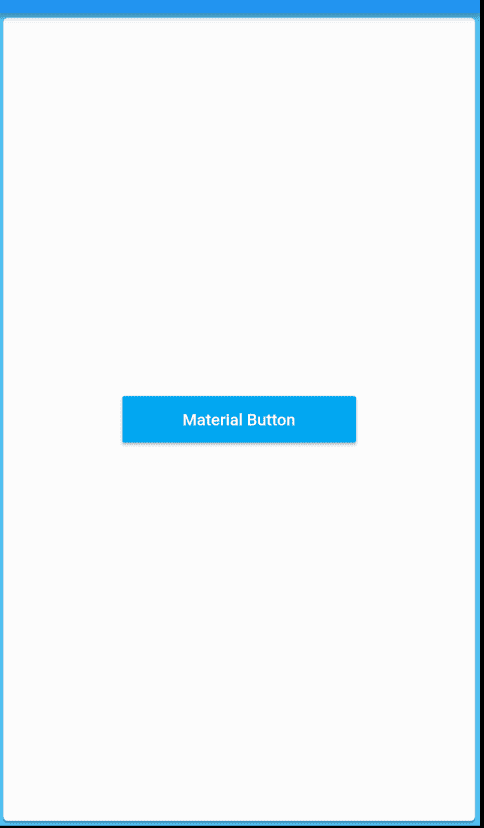
Although we can add any type of widget, and there are some that are really great for us when working with Cards in Flutter.
As you can see, something quite boring and poor, but of course we can use any other widget and there are widgets that will help us in this task, for example one of the column type with which we can add many other widgets:
body: Card(
child: Center(
child: Column(
children: <Widget>[
Image.asset('assets/codeigniter.png'),
Text("Curso en CodeIgniter"),
IconButton(
icon: Icon(Icons.access_alarms),
tooltip: "Mensaje",
),
],
),
),
),
Remember that if you don't know how to use images in Flutter, you can review the previous entry where we explained how to do it.
As you can see in this example, the ideal is to combine widgets; take the characteristics of a widget and thus add them together; As we indicated before, Cards in Flutter can only contain one widget; therefore it is almost mandatory to use other widgets such as Column that allows us to add the elements we want.
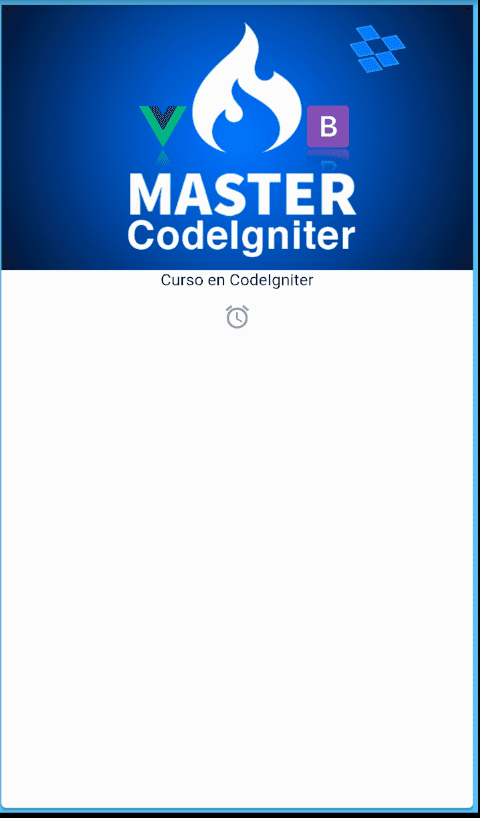
https://kodestat.gitbook.io/flutter/37-flutter-using-cards
Padding and margins in Flutter Cards
Of course, like any container or layout that we use in our applications, it needs a margin; there are also properties for this reason, for that we can use the padding property as shown below:
Container(
padding: new EdgeInsets.all(32.0),
height: 350,
child: Card(
color: Color.fromRGBO(84, 197, 248, 1),
child: Center(
child: Column(
children: <Widget>[
Image.asset('assets/codeigniter.png'),
Text("Curso en CodeIgniter"),
IconButton(
icon: Icon(Icons.access_alarms),
tooltip: "Mensaje",
),
],
),
),
),
)
Here on this occasion we vary some things; First we define another type of layout, which is the container that, as you can guess from its name, allows an element to be contained through its child attribute; among those elements we place our Card; and not only that, in this same container we define our internal spacing through the padding property; we also take advantage and define the height so that it does not occupy the entire screen:
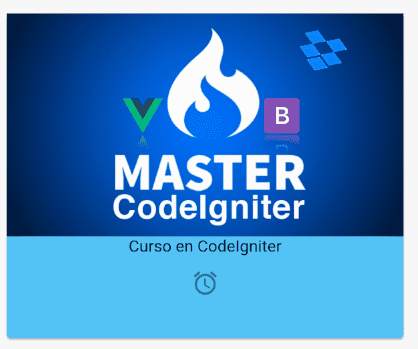
Rounded edges for Cards
If you come from native Android development, you will know that one of the main characteristic elements of CardView is precisely that they have rounded borders by default; here we can also create our rounded edges on our Cards using the shape property:
Container(
padding: new EdgeInsets.all(32.0),
height: 350,
child: Card(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
color: Color.fromRGBO(84, 197, 248, 1),
child: Center(
child: Column(
children: <Widget>[
Image.asset('assets/codeigniter.png'),
Text("Curso en CodeIgniter"),
IconButton(
icon: Icon(Icons.access_alarms),
tooltip: "Mensaje",
),
],
),
),
),
),
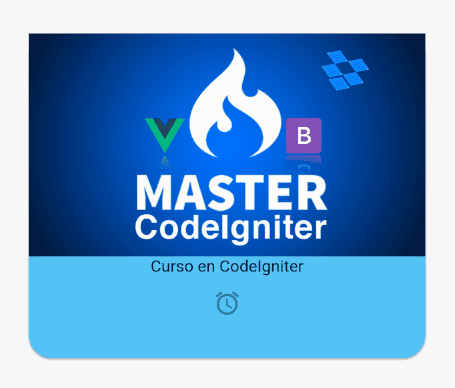
Finally, here is the complete code of our application as well as the link to the official documentation:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
home: Scaffold(
// backgroundColor: Color.fromRGBO(84, 197, 248, 1),
appBar: AppBar(
title: Text('Card'),
),
body: Container(
padding: new EdgeInsets.all(32.0),
height: 350,
child: Card(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0),
),
color: Color.fromRGBO(84, 197, 248, 1),
child: Center(
child: Column(
children: <Widget>[
Image.asset('assets/codeigniter.png'),
Text("Curso en CodeIgniter"),
IconButton(
icon: Icon(Icons.access_alarms),
tooltip: "Mensaje",
),
],
),
),
),
),
),
);
}
}
If you want to have a good idea of how you can use Cards in Flutter, which components you can place and their organization, you can see the widget tree in the official documentation:
I agree to receive announcements of interest about this Blog.
We are going to learn to work with the Cards that have been the container par excellence that we can use in Material Design.
- Andrés Cruz