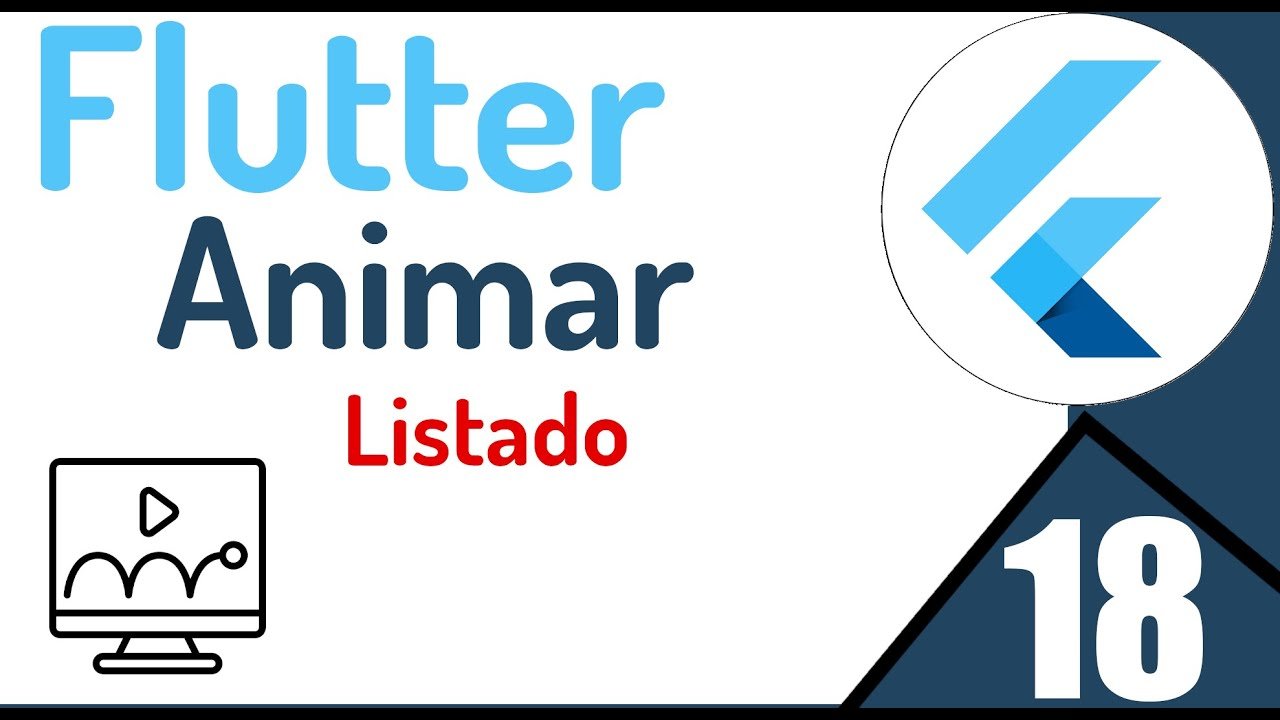
In Flutter, ListView is a widget that is used to display a set of items in a list. ListView is an extremely flexible widget and allows you to display a variety of formats as well as lists in landscape or portrait format, with custom or standard elements. Additionally, ListView is highly performing and ideal for large data sets, as it only loads the items that are currently displayed on the screen.
Create a listview
To create a ListView in Flutter, you first need a dataset on which to build the list. You can then use the ListView.builder widget to build the list dynamically as needed, giving it the necessary elements to display in the list as the user scrolls through it:
ListView.builder(
itemCount: items.length,
itemBuilder: (BuildContext context, int index) {
return ListTile(
title: Text(items[index]),
);
},
);
In this example, items is a list of items to display in the list, and for each item a ListTile will be created, containing a title that displays the item's text.
This is just a basic example, there are many other options and customizations available for the ListView in Flutter.
Animated
Animations are essential in any application we build today, equipping the application to show smooth changes or transitions between one state to another is normal; In the lists through the ListView in Flutter things change a bit, since, due to their behavior, the lists start the animation when an item appears on the screen, it becomes more complicated to carry out this task.
There are many ways to animate a listing; but, to keep it simple, we are going to use the following package:
https://pub.dev/packages/delayed_display
We install the package with:
pubspec.yaml
dependencies:
flutter:
sdk: flutter
syncfusion_flutter_datepicker:
delayed_display:
Which allows adding delays or delay to the widget that is embedded by the DelayedDisplay() widget; In addition to this, we can specify a simple "fade In" type animation which we can customize the direction of the animation by indicating the axis where the effect starts; for example, if we want it from left to right:
slidingBeginOffset: const Offset(-1, 0)
Or vice versa (right to left):
slidingBeginOffset: const Offset(1, 0)
From top to bottom:
slidingBeginOffset: const Offset(0, -1)
Or vice versa (from bottom to top):
slidingBeginOffset: const Offset(0, 1)
You can customize other aspects such as the animation curve among other details that you can review in the official documentation.
Finally, we will use the mentioned widget:
ListView.builder(
itemCount: 50,
itemBuilder: ((context, index) => DelayedDisplay(
delay: const Duration(milliseconds: 5),
slidingBeginOffset: const Offset(-1, 0),
fadeIn: true,
child: Card(***)
- slidingBeginOffset, we specify the animation curve through the Offset, which receives the X and Y position, which are the factors taken into account to perform the displacement effect.
- fadeIn, enables or disables the fade in effect.
- delay, indicates the delay in the animation.
Remember that this post is part of my complete course on Flutter.
I agree to receive announcements of interest about this Blog.
We're going to animate a To Do list in the app to improve the user experience by smoothly changing the list as the user scrolls through it.
- Andrés Cruz