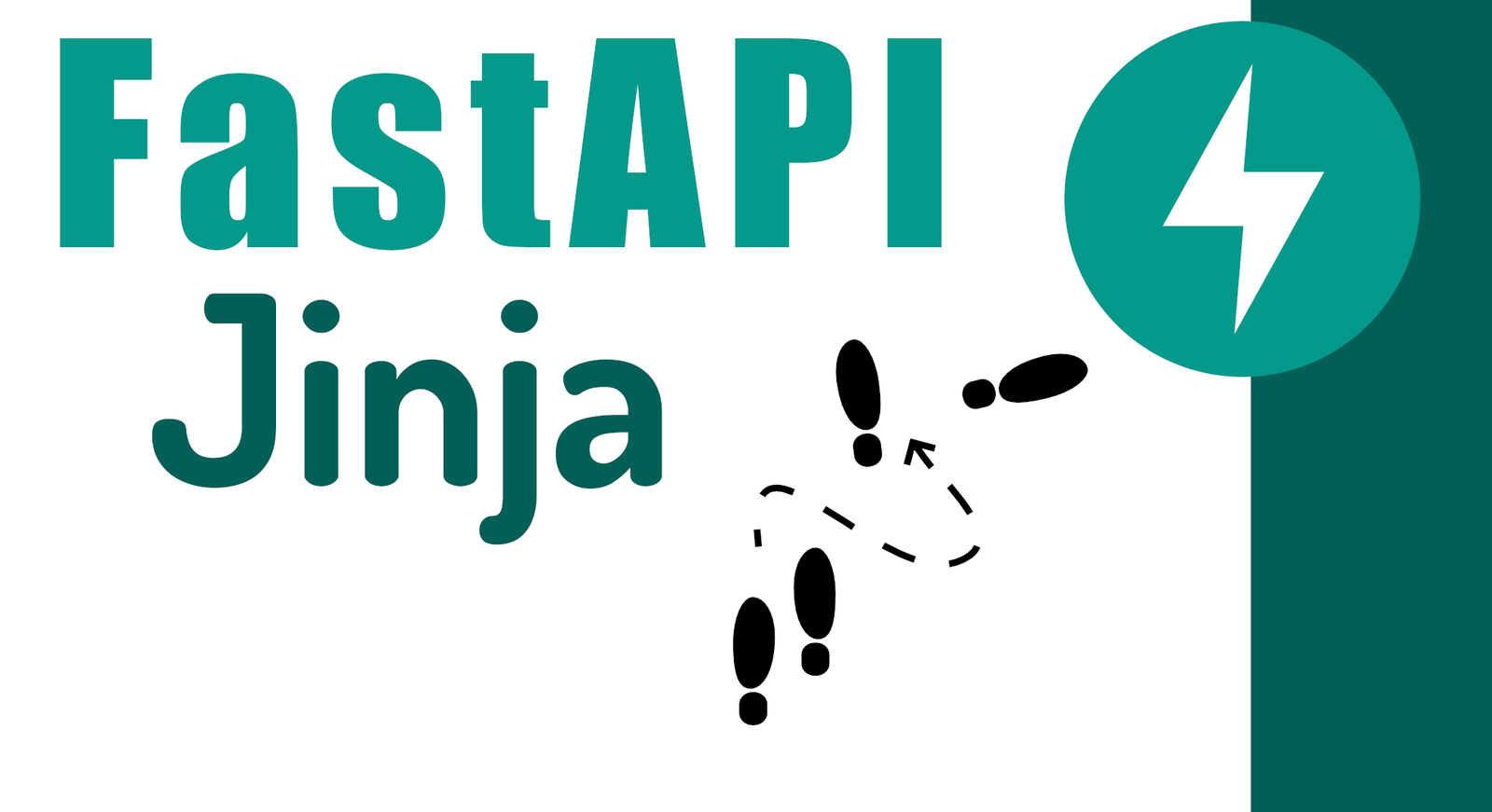
One of the problems we currently have is that we do not have an effective way to present the data to the end user. Since Flask is a web technology, the usual thing is to present this data on a web page; this is where what is known as the template engine becomes important; a template engine is a resource used to present this data from the server and generate a web page in the process, they are the next layer of the MVC that corresponds to the view and allows the presentation logic to be separated from the data; to do this, a set of features are used to manipulate the server data and generate the HTML page that will be consumed by the user. Features such as impressions, filters, conditionals, cycles or imports allow generating this HTML code necessary to represent a page. Web. They are widely used in server-side programming, since they allow the presentation of data without having to modify the source code.
In short, with a template engine it is possible to present the data managed by the server, specifically in our case in Flask and generate the HTML in the process; for example, if we have a list of users, we can print it to a table or similar in HTML using certain functionalities (directives).
About Jinja
- The {{ }} syntax is called a variable block and we can use it to print to the template.
- The {% %} syntax houses control structures such as if/else, loops, and macros.
Set up Jinja in Flask
<!DOCTYPE html>
<html lang="en">
<head>
<title>Page
Hello Flask
Lorem ipsum dolor sit amet consectetur, adipisicing elit. Reiciendis libero possimus nihil ipsam quam deleniti
voluptas eaque qui. Necessitatibus corporis quas odio tenetur quos, recusandae itaque doloremque assumenda
consequatur aliquam.
from flask import render_template, request
@app.route('/')
@app.route('/hello')
hello_world():
render_template('index.html')
from flask import render_template, request
@app.route('/')
@app.route('/hello')
hello_world():
name = request.args.get('name', 'DesarrolloLibre')
render_template(, name=name)
request.args.get('name', 'DesarrolloLibre')
name=name
name=name
<!DOCTYPE html>
<html lang="en">
<head>
<title>Page
Hello {{name}}</h1>
</body>
http://127.0.0.1:5000/
http://127.0.0.1:5000/?name=Andrew
Hello Andrew
app = Flask(__name__, template_folder="<ROUTETEMPLATES>")
Control blocks
Conditionals
<div>
{% if True %}
Is TRUE
{% endif %}
</div>
Bucle for
<ul>
{% for item in items %}
<li>{{ item }}{% endfor %}
- loop.index: Gets the current index of the iteration starting from one.
- loop.index0: Gets the current index of the iteration starting from zero.
<ul>
{% for e in [1,2,,,,,] %}
<li>{{ e }} {{ loop.last }} endfor
Filters
<DATA> | <FILTER>
{{ task | default('This is a default Task') }}
This is a default
{{ 5 | default('This is a default Task') }}
5
<title1>Todo Application/title1
3
31.0
{{ [1, 2, , , ]|max }}
{{ [1, 2, , , ]|min }}
- One for the title in the header.
- One for the title in the content.
I agree to receive announcements of interest about this Blog.
We will give an introduction to Jinja 2 focused on Flask, we will learn about the template engine, filters, template inheritance, blocks among other aspects.
- Andrés Cruz