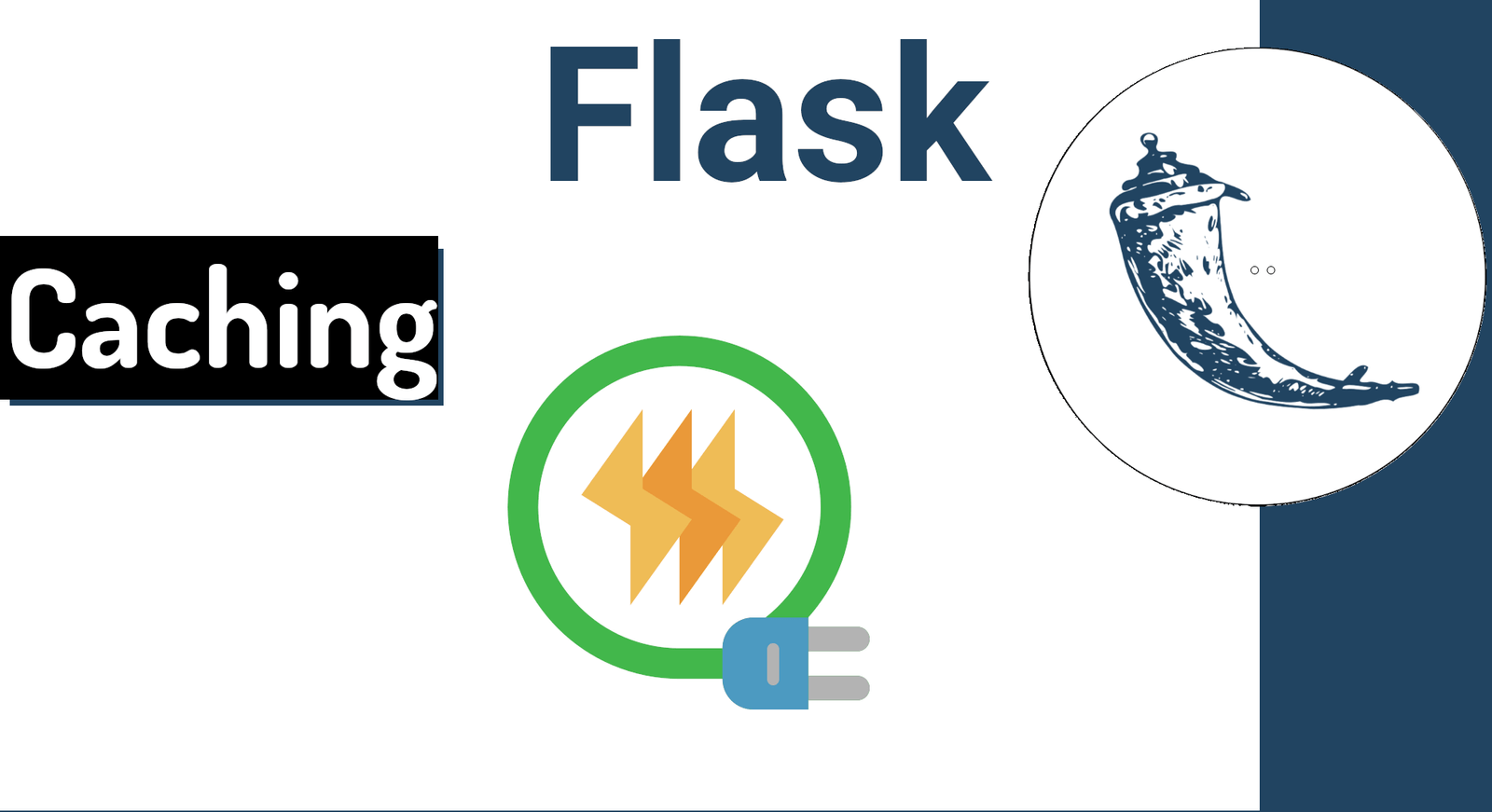
One of the great challenges we have once the application is created is to optimize it, so that it consumes fewer resources and also so that it returns responses more quickly; these aspects are required under many circumstances, for example, for SEO.
Page loading speed is one of the most important factors that will determine the success or failure of a web application.
Flask Caching allows you to store data in cache, so that it can be returned more quickly; this data is usually data from the database or documents processed in HTML, in this way, when receiving a request from the user, it is checked if it is cached:
- If the results are cached, the cached results are returned.
- If the results are not cached, they are generated as implemented in the controller/view and then cached.
We are going to install Flask Caching with:
$ pip install Flask-Caching
The next step is to register it at the application level:
app\my_app\__init__.py
from flask_caching import Cache
***
#cache
cache = Cache()
cache.init_app(app)
# blueprints
***
The next step is to configure the cache storage, which can be the ones you can consult in the following link:
https://flask-caching.readthedocs.io/en/latest/#built-in-cache-backends
We will use:
my_app\config.py
class DevConfig(Config):
***
CACHE_TYPE = 'SimpleCache'
The SimpleCache option tells Flask Cache to store results in memory in a Python dictionary, which for most Flask applications will be enough.
Caching templates and functions
To cache the result of a controller/view, simply indicate the decorator cache.cached:
my_app\tasks\controllers.py
from my_app import cache
***
@taskRoute.route('/')
@cache.cached(timeout=60)
def index():
return render_template('dashboard/task/index.html', tasks=operations.pagination(request.args.get('page', 1, type=int), request.args.get('size', 10, type=int)))
The timeout parameter specifies how many seconds the result cache should last.
When using the cache in a view, you must place the route decorator before the cache decorator.
Parameterless caching functions
Driver results aren't the only thing that can be cached. To cache any Python function, simply add a similar decorator to the function definition, as follows:
@cache.cached(timeout=60, key_prefix='unique_key')
def myFunction():
***
The key_prefix argument is required so that Flask Caching can store the results of non-view functions. The value must be unique for each function we want to cache.
Caching functions with parameters
The previous decorator cannot be used in functions that have arguments, in these cases we must use the memoize decorator so that they are included in the cache:
class Person(db.Model):
@cache.memoize(50)
def has_membership(self, role_id):
return Group.query.filter_by(user=self, role_id=role_id).count() >= 1
Memoize is also designed for methods, as it will take into account the identity of the 'self' or 'cls' argument as part of the cache key.
While developing the app, we don't want the cache functions to be running as the screens change as we update the app. To avoid this, we set the CACHE_TYPE variable to null for the developing configuration:
my_app\config.py
my_app\config.py
class Config(object):
***
CACHE_TYPE = 'SimpleCache'
class DevConfig(Config):
***
CACHE_TYPE = 'null'
I agree to receive announcements of interest about this Blog.
We will see how to use the caching system known as Flask Caching, with which we can cache controllers/views or functions with or without arguments.
- Andrés Cruz