Configuration files in Flask allow us to add global parameters to our project; enable testing mode, debug, place the secret key of the app used, for example, for data encryption, among many other configurations that you can add in Flask.
Flask provides us with some mechanisms with which we can manage configuration parameters of our project, for example environment, database, connections to third-party services, etc.
These settings are made in configuration files in Flask allow us to add global parameters to our project; enable testing mode, debug, place the secret key of the app used, for example, for data encryption, among many other configurations that you can add in Flask.
The configuration in Flask is either a class/subclass or just a dictionary and can be changed programmatically and at runtime from our Flask project; For example, take a look at the following lines of code where we are programmatically changing the debug mode to true, and we are doing it through a config bootstrap or just a dictionary and in this way we can use the config object in Flask programmatically:
app = Flask(__name__)
app.config['DEBUG'] = True
app.debug = True
Configuration based on environment variables
As we discussed in the previous article, we also have settings that we can use as environment variables:
DEBUG = True
TESTING = True
For example, to modify the environment from an environment variable:
export FLASK_ENV=development flask run
Configuration parameters based on classes or objects
This has been the most complete and organized approach and therefore it is the recommended one that we use in our course; since, as we are going to see, it offers us the possibility of using different configurations depending on the environment we find ourselves in, we can make different configuration parameters based on classes, which is a very interesting mechanism that we have to have configurations of all kinds, and for different development environments.
We just create a file with any name and extension from .py and add a class; for example a file called configuration.py; For example, in our Flask course, we use the following configuration:
class BaseConfig(object):
USER_APP_NAME = 'FlaskMalcen'
USER_ENABLE_EMAIL = True
'Base configuracion'
SECRET_KEY = 'Key'
DEBUG = True
TESTING = False
SQLALCHEMY_DATABASE_URI="mysql+pymysql://root:root@localhost:3306/pyalmacen2"
RECAPTCHA_PUBLIC_KEY='6LffHtEUAAAAAI_KcKj6Au3R-bwhqRhPGe-WhHq_'
RECAPTCHA_PRIVATE_KEY='6LffHtEUAAAAAJdHhV9e8yLOSB12rm8WW8CMxN7X'
BABEL_TRANSLATION_DIRECTORIES='/Users/andrescruz/Desktop/flask/4_flask_app/flask_app/translations'
#WTF_CSRF_TIME_LIMIT = 10
class ProductionConfig(BaseConfig):
'Produccion configuracion'
DEBUG = False
class DevelopmentConfig(BaseConfig):
'Desarrollo configuracion'
DEBUG = True
TESTING = True
SECRET_KEY = 'Desarrollo key'
#MAIL_SUPPRESS_SEND = False
MAIL_SERVER = "smtp.mailtrap.io"
MAIL_PORT = 2525
MAIL_USERNAME="xxx"
MAIL_PASSWORD="xxx"
#MAIL_DEFAULT_SENDER=('andres de DL',"andres@gmail.com")
MAIL_USE_TLS=True
MAIL_USE_SSL=False
USER_EMAIL_SENDER_EMAIL="andres@gmail.com"
CACHE_TYPE = "simple"
Defining configuration parameters using inheritance between classes
Now, suppose we are going to define a base class to make the initial or base configurations that we want the project to have, and with this we can inherit it to other classes that will be the different environments or modes that we want our application to have.
To load it in our app, and therefore that our project can use these configurations; for example, development mode:
app.config.from_object('configuration.DevelopmentConfig')
Or the production one:
app.config.from_object('configuration.ProductionConfig')
The interesting thing about this is that we can easily use common configurations that are the ones we have in our class called BaseConfig and we can inherit them to other classes that are the ones we use to indicate the different modes of our application and this is the one we use in the course on Flask.
Extra: Load configurations dynamically
Many times, we need to get dynamic data to create the configuration; for example, for the file upload folder: we can perfectly define it in the init of our app:
app.config['UPLOAD_FOLDER'] = os.path.realpath('.') + '/my_app/static/uploads
As you can see, this is the most complete version that you can choose when developing your Flask configurations, since we can easily vary from one environment to another, activating or deactivating certain configurations automatically with just changing a line of code.
I leave you the official documentation for more detail.
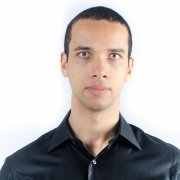
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter