We can now start working according to the project we created with Electron and Node; we are going to know in detail and in practice the concepts of "main process" and "rendering process (web page)".
We will now see our package.json with the Electron dependency:
package.json
{
"name": "electron-chat2",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "electron ."
},
"author": "",
"license": "ISC",
"devDependencies": {
"electron": "^20.0.3"
}
}
In the root of the project, we are going to create a JS file with the following content:
index.js
const { app, BrowserWindow } = require('electron')
function createWindow() {
let win = new BrowserWindow({
width: 800,
height: 600,
})
win.loadFile("index.html")
}
app.whenReady().then(createWindow)
First, you need to import the necessary objects and classes that we are going to use to work with; Electron when using Node, is quite modular and therefore, we import the necessary elements to work with it, in this case:
- A reference to an object of type BrowserWindow that you are going to instantiate and display to your users.
- With the app object, I control the life cycle of the application.
After that, we need to wait for the application to be ready before we can create a small window 800 x 600 in size (the size specified by new BrowserWindow({ width: 800, height: 600, })).
Finally, the content of the index.html file, which contains the main content of the Electron application, is loaded and displayed:
win.loadFile("index.html")
Although we have not yet defined the index.html file, as you can guess, this corresponds to the rendering process or web page, let's remember that web pages have the HTML extension.
The app.whenReady() function returns a promise when Electron is initialized (and thus ready to make calls to the OS, in this case, to create a window).
This file, as you can guess, is the main process in which we maintain communication with the OS with the wide API of features provided by Electron, in this case, the function that allows us to create a window.
Now, we need to show some of the Graphical Interface to the user, buttons, texts, images, etc. For this, we have to work on the rendering process or web page, which, as its name indicates, is an HTML page that can have any HTML content:
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello Electron</h1>
</body>
</html>
As you can see, in the previous web page, we can place any type of HTML content, in this case, a welcome message.
Now we need a mechanism with which to run the application and be able to display the window with the greeting; we create the command to run our application:
package.json
{
"name": "electron-chat2",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "electron ."
},
"author": "",
"license": "ISC",
"devDependencies": {
"electron": "^20.0.3"
}
}
And when executing in the terminal the command of:
$ npm run start
Remember that the previous material is part of my complete course on Electron.js
The next step is, avanzar en el desarrollo de la aplicación de Electron.js.
- Andrés Cruz
En español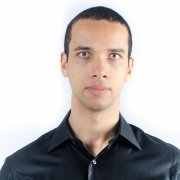
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter