Django Admin is an application that is installed by default when we create a project in Django. In order to use it, we need other applications that already come with our framework; as:
'account', 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles',
All these applications can be seen and managed in the global configuration file of the project. For example; to achieve authentication in our Django Admin application we use the app called django.contrib.auth, and this is a part of using frameworks like Django, and that is that we can easily reuse components, modules, applications... and even the same framework does the same.
Access to the administration panel
By default, django uses the /admin url for the admin panel. To access the admin panel, we first need to run our project.
Note: If you don't know how to run the server or how to create a project in Django, I recommend reading my getting started with Django article.
First, let's open our urls.py file. This is the file that contains URL routing at the project level. When we create new web pages or APIs, we need to add these URLs to urls.py for routing.
Take a look at the urls.py file at the project level.
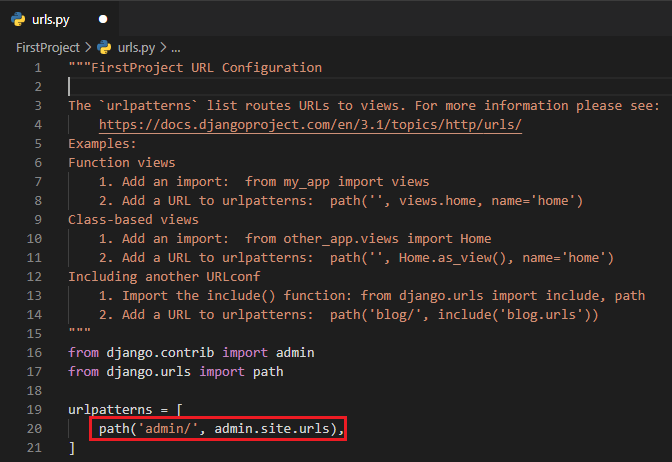
As you can see in the image above, I have marked line 20 with a red rectangle. Here, the first parameter says what our URL is and the second parameter says that this URL will be the admin panel of our project.
After running our server successfully, let's open our browser and go to this website http://127.0.0.1:8000/admin
Note: If you run your project at a different URL, don't forget to change this area.
What exactly allows us Django Admin?
Django Admin offers us a configurable interface through classes to be able to create simple CRUD processes simply through a class schema; In other words, just by defining a class, some attributes such as indicating, model, which fields we want to be manageable in the forms, fields for the list, filters, etc; with this, Django Admin automatically creates a complete CRUD for these fields:
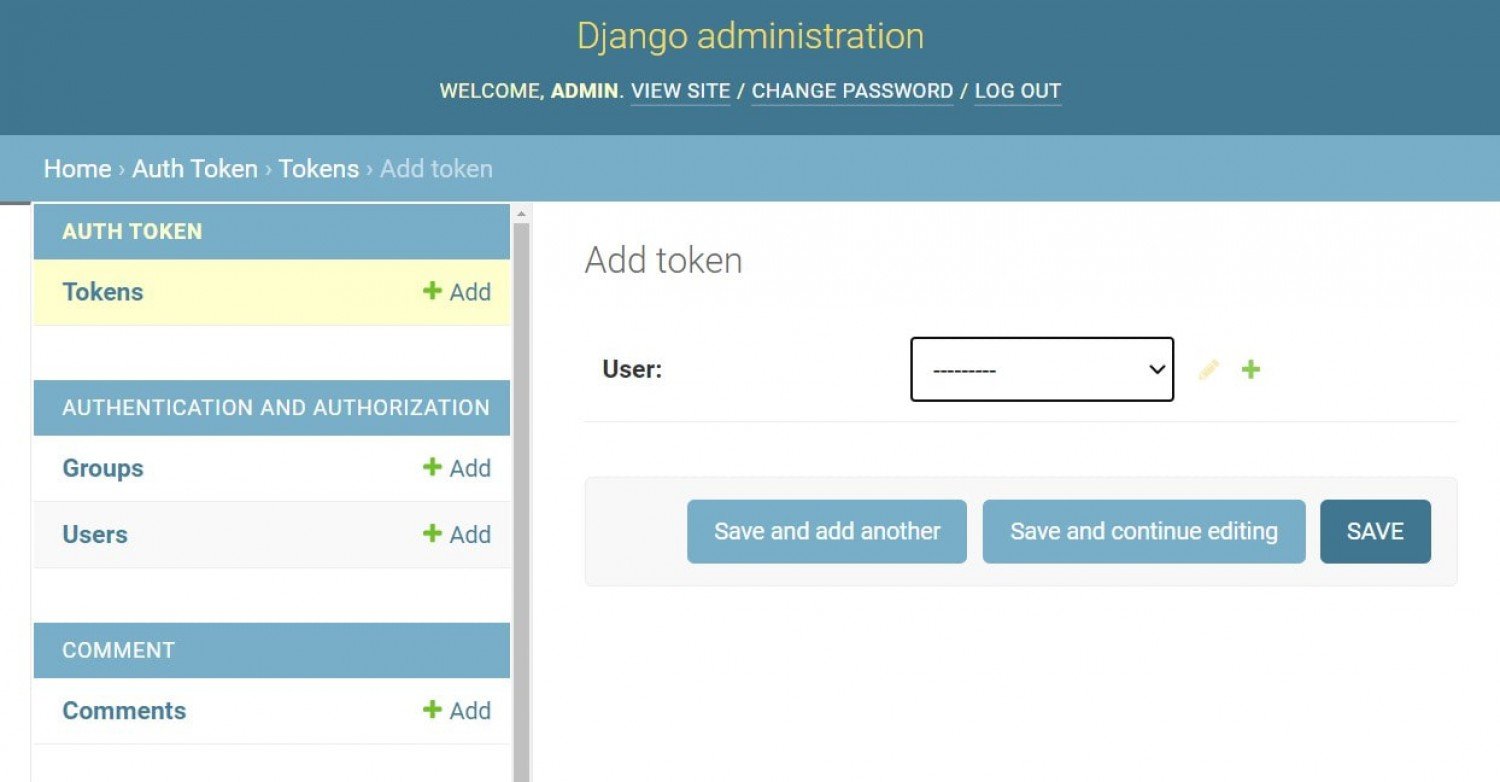
Customize classes to create CRUDs with Django Admin
from django.contrib import admin from .models import Comment # Register your models here. admin.site.register(Comment)
And that's it, in this post we saw a simple example of how we can use and customize Django Admin for our project, but remember that you can get more information on how you can customize your CRUD processes through this powerful application; You can continue to see the official documentation or follow my Django course in which we see a bit of everything with this famous framework.
Customize Django Admin
Django Admin has a great level of customization in how we want to display the data, apply filters, searches, what data we want to manage, etc; for these we have to define the series of features implemented from a class like the following:
# Register your models here.
class CategoryAdmin(admin.ModelAdmin):
pass
admin.site.register(Comment, CategoryAdmin)
Like almost everything in Django, it is nothing more than a Python class that inherits from an internal class to the framework in which we apply the features; then, we register it through site.register() in which we indicate the model and the admin/features class.
Let’s see some common features that we can apply.
Define columns for lists
If you want to place specific columns from the list, just define a tuple called list_display with the columns you want to display:
# Register your models here.
class CategoryAdmin(admin.ModelAdmin):
list_display = ('id','text')
admin.site.register(Comment, CategoryAdmin)
Search fields
We can specify by which fields of our table the list will be searched:
class CommentAdmin(admin.ModelAdmin):
list_display = ('id','text')
search_fields = ('text', 'id')
Navigation by dates
Just below the search bar, there are navigation links to navigate through a hierarchy of dates:
class CommentAdmin(admin.ModelAdmin):
list_display = ('id','text')
search_fields = ('text', 'id')
date_hierarchy = 'date_posted'
Ordering
We can specify the sort field in the list:
class CommentAdmin(admin.ModelAdmin):
list_display = ('id','text')
search_fields = ('text', 'id')
date_hierarchy = 'date_posted'
ordering = ('date_posted',)
Filtered
We can also add filters in the list:
class CommentAdmin(admin.ModelAdmin):
list_display = ('id','text')
search_fields = ('text', 'id')
date_hierarchy = 'date_posted'
ordering = ('date_posted',)
list_filter = ('id', 'date_posted')
Editable fields
From the list, you may be able to modify fields:
class CommentAdmin(admin.ModelAdmin):
list_display = ('id','text')
search_fields = ('text', 'id')
date_hierarchy = 'date_posted'
ordering = ('date_posted',)
list_filter = ('id', 'date_posted')
list_editable = ('text',)
Indicate manageable fields
Depending on the model you are working with, you may want to define only a few manageable fields (to create and edit):
class CommentAdmin(admin.ModelAdmin):
// ***
fields = ('text',)
Exclude manageable fields
We can also exclude manageable fields:
class CommentAdmin(admin.ModelAdmin):
// ***
#fields = ('text',)
exclude = ('text',)
Register classes using a decorator
Another way in which we can register the classes is through a decorator:
@admin.register(Comment)
class CommentAdmin(admin.ModelAdmin):
list_display = ('id','text')
//***
Register the model for labels, categories and elements
Now that we've seen how easy it is to use Django Admin for simple models, let's use it to record types and category relationships:
elements/admin.py
from django.contrib import admin
from .models import Type, Category
@admin.register(Type, Category)
class CategoryAdmin(admin.ModelAdmin):
list_display = ('id','title')
And the element:
@admin.register(Element)
class ElementAdmin(admin.ModelAdmin):
list_display = ('id','title','category','type')
fields = ('title','slug','description','price','category','type')
Group management fields
Using tuples, it is possible to group form fields to place on a single line, for example, if we want to place the title and the slug on a single line, as well as the type and category, we have:
@admin.register(Element)
class ElementAdmin(admin.ModelAdmin):
list_display = ('id','title','category','type')
fields = (('title','slug'),'description','price',('category','type'))
And it looks like:
- Andrés Cruz
En español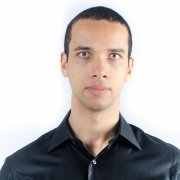
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter