The information lists are a fundamental element in any application, be it a blog, product type, to carry out a CRUD, etc; all this is part of the same logic and structure that is to load the list of elements and maybe under a particular criteria (that they are approved, paginated, etc.) and we are going to see how we can create a view or list template using Django .
We are going from the previous entry in which we already created the migration to our MySQL database; so remember that in order to create the migration we had to have created a model that is part of our MTV.
Get all records from the database with the Django ORM (all())
So; The first thing we need is to be able to obtain all the elements; For this we can use the ORM provided by Django which together with the model we can perform any CRUD operation, in this case we are interested in the read-only operation.
Let's go to our views file called views of our application firstApp and create a new function called index:
def index(request):
And the first thing we're going to do is get the logs; that is, the comments for that we have to load the model that we previously defined for that in this same view file (remember that the models file is in the same app):
from .models import Comment
And now from the function that we created earlier we simply have to refer to the model that we loaded; i.e. the class:
comments = Comment.objects.all()
Here, as we mentioned, we refer to the model class called Comment, then it objects followed by the CRUD type method that we want to use. In this case, since we want to obtain all the records, we have to use the method called all:
And with this we get all the records; now we simply have to pass them to the view; For that we can use the function called tender as we did before with the usual parameters that would be:
- The request
- The HTML page or template
- A dictionary with the data that we are going to pass to the view.
return render(request,'index.html',{'comments' : comments })
Finally, the code is as follows:
from .models import Comment
def index(request):
comments = Comment.objects.all()
return render(request,'index.html',{'comments' : comments })
The template (HTML View), iterate all records
Then, we are going to create a template inside fistApp that remembers that it is the HTML view and a folder called templates that you have to create that we are going to call index.html or whatever name you want it to have:
firstProject\firstApp\templates\index.html
In this template, we're going to place a basic HTML page and just a directive called for, which works like any for loop in any programming language.
As you can see, it receives an iterable object, which in this case would be the comments collection, and then we want to print them on the screen; To print a value on the screen we simply have to use the double braces:
<!DOCTYPE html>
<html lang="es">
<head>
<meta charset="UTF-8">
</head>
<body>
{% for c in comments %}
<div>
<p>
{{ c.text }} - {{ c.date_posted }}
</p>
</div>
{% endfor %}
</body>
</html>
For the for directive, you have to use the logical labels, which are the ones that are made up of a key and a percentage.
Create a route for the listing page
Now we have almost everything ready, we are going to create the route in the file called (within the application that you also have to create) urls.py inside the firstApp application:
firstProject\firstApp\urls.py
app_name='comment'
urlpatterns = [
path('', views.index, name='index'),
]
And remember to load the previous file (the one with the routes) inside your project; for that:
firstProject\firstProject\urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('fisrtApp/', include('firstApp.urls'))
]
As you can see, in the application urls file, we simply record a path, without path ''. that is resolved by the index function in the index.py file and we optionally give it a name. Then, we register ALL the routes (in this case only one) of the application at the project level, for that we include them through the Django include function.
And with this, if we enter the previous route:
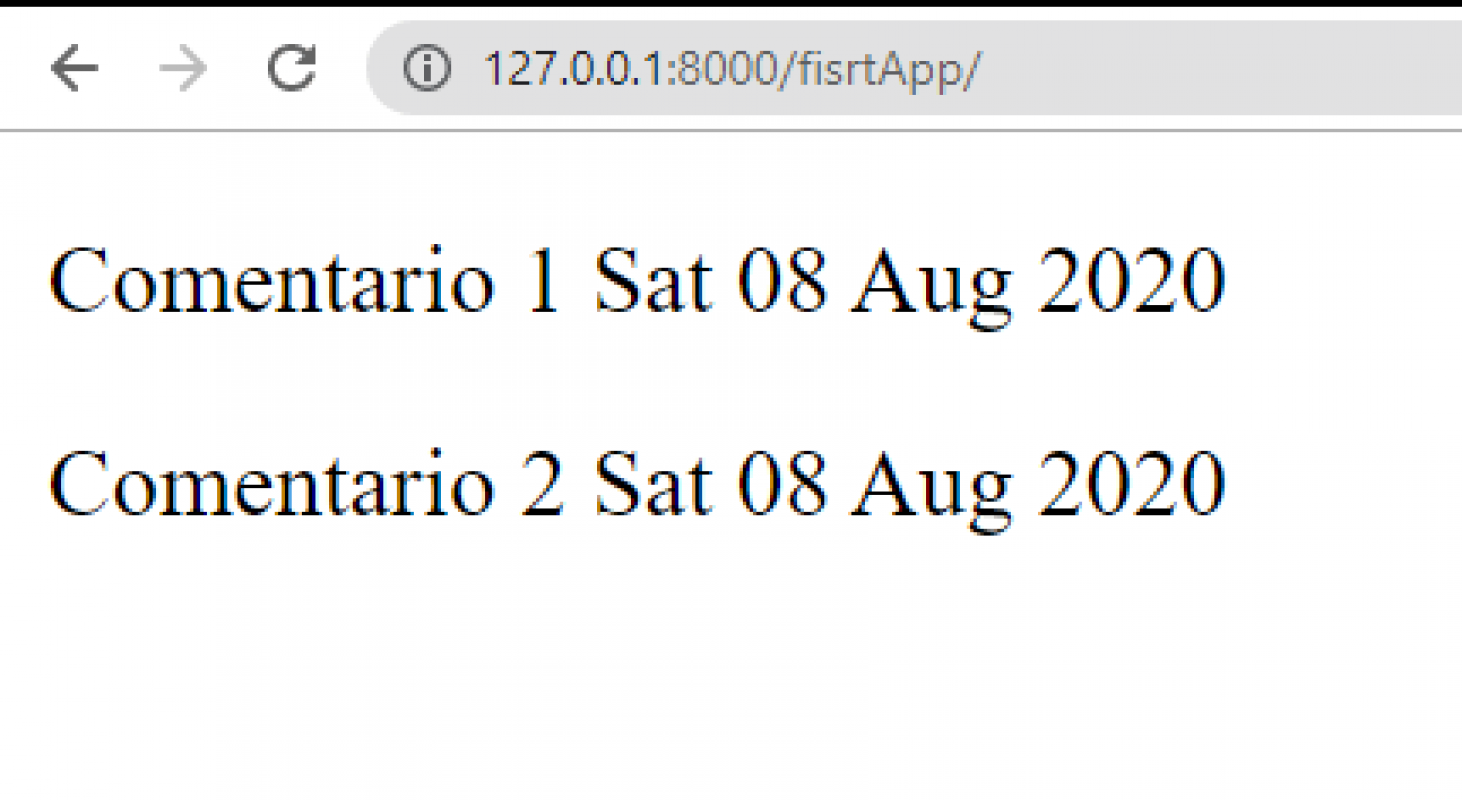
We already have our list.
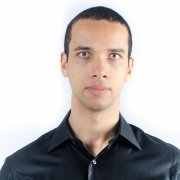
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter