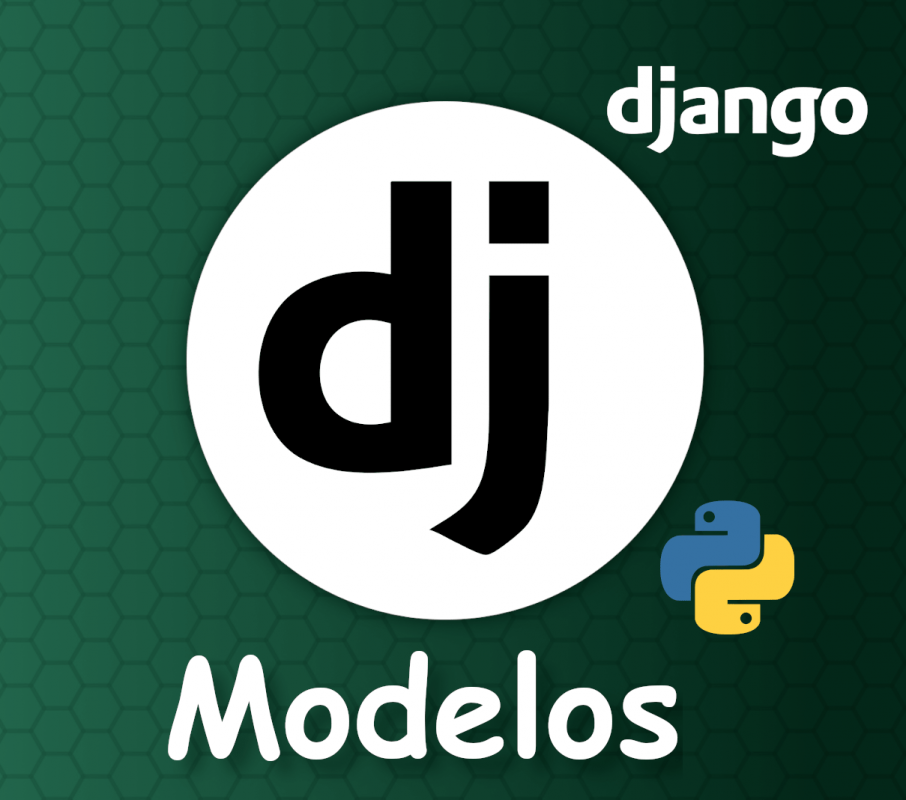
In this post we are going to see how we can create a model in Django; in previous posts we already discussed how we can create the connection to the database of a project in Django with MySQL, now we are going to take advantage of that connection to be able to make more interesting views and templates and not just a hello world or a text print or static visas as we saw previously.
This entry provides a fairly general usage for django-models; in such a way, that you will know what they are for and what else we can use them for, for example, for migrations and tables in the database through Django.
What is a model?
Models and the single application file in Django
A huge advantage that we have when creating classes such as models or forms in Django is that we can define all the structures, that is, the classes, in a single file, therefore we can have great control over each of the layers or sublayers of our project. .
Create a model in Django
Field types
class Comment(models.Model):
text = models.()
date_posted = models.(auto_now_add=)
element = models.(, =', =., =)
def __str__():
return ' #{}'.format(.)
I agree to receive announcements of interest about this Blog.
We are going to see how we can create a model in Django, which remember is one of the MTV layers of our application and the one in charge of managing its data; we will also see how to define different fields.
- Andrés Cruz