At this point you probably know that Django has a login module that is mainly designed to work with the admin; all this is free by simply creating a project in Django, since applications such as admin and login (auth):
INSTALLED_APPS = [
'account',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
They come for free when creating a project in Django, now, surely at some point you will be interested in creating a custom process for the login, either to replace the original one, or more interesting, to use it in parallel; for this in our configuration file; settings.py, we have the following section (which we have to add if we want to create a custom login in Django):
AUTHENTICATION_BACKENDS = [
'django.contrib.auth.backends.ModelBackend',
'account.authentication.AuthByEmailBackend',
]
In which we can configure our authentication backends or to perform the login, as you want to see it; as you can see, it is a list, an iterable, therefore we can define more than one and this is excellent since Django will go one by one asking for the combination of username and password that we establish in the application one by one, and if the first in the false list goes to the second and so on until they are all covered; As you can see, it is a behavior that allows us to extend the basic operation of a Django application to handle login.
The first backend you define would be the one Django uses by default; the following would be a file that defines a class that we are going to create in this post.
Creating our authentication class (Custom Login)
In this post we are going to see how to create a custom backend with Django; for this, the first thing we have to do is create a class, it's basically all we need, a class that we can then register in the list we saw above our settings.py; this class will be created of course, it is in a file with any name and it can be in any application of your project; for example, an application called:
python manage.py startapp account
In which we are going to create a file called authentication.py with the following content:
from django.contrib.auth.models import User
"""
Login via email
"""
class AuthByEmailBackend:
def authenticate(self, request, username=None, password=None):
try:
user = User.objects.get(email=username)
if user.check_password(password):
return user
except User.DoesNotExist:
return None
def get_user(self, user_id):
try:
return User.objects.get(pk=user_id)
except User.DoesNotExist:
return None
Explain the above code
As you can see, it is any class, we do not have to inherit from anything, but it does have to have a method with the name authenticate that receives the request, in case you want to pass any additional data, and the username and password are mandatory. .
For the rest, everything remains to do the checks you want to do for the login, in this example, to expand the application, we also search for the email and then we check the hash of the password that Django uses internally for the creation of users; now with this, we go to our settings.py and add the reference to the new class:
AUTHENTICATION_BACKENDS = [
'django.contrib.auth.backends.ModelBackend',
'user.authentication.AuthByEmailBackend'
]
Remember that in your custom backend you can define any type of rules to make your custom login.
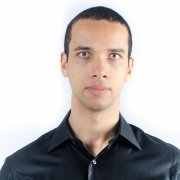
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter