Send get and post requests (Form) in CodeIgniter 4
We are going to learn how to make the typical submission of data from an HTML form with some HTML fields defined in it in CodeIgniter 4, be processed by a particular controller or function defined within the controller; for this we are going to need a couple of functions, an HTML view and a model that we already explained previously, in addition to having the connection to the database and the generated table; all these are concepts that we have already seen before and therefore you can visit the corresponding articles.
Remembering...
A very important point if we remember how we worked in Codeigniter 3, that is, the previous version of the framework, is that you have more flexibility when defining this type of process; that is, we could perfectly define the entire process of painting the view/form and processing it in a single function, but this in CodeIgniter 4 is not recommended and it is not the approach we should follow here; and this is a very important point, CodeIgniter 4 takes the organization of other frameworks such as Laravel to define resource-type controllers that we will address in another entry, but in a few words it allows us to create all CRUDnroutes.
Components needed to work with forms in CodeIgniter 4
- A view that will only be consumed via get, a get type request and is the one that will be in charge of painting our view, specifically our form
- Another view that will be in charge of processing the request of the form that will go via Post, which has been the type of recommended method to create resources (and CodeIgniter also uses this type of request to update records, but we will see this in another post.) .
- An HTML view, which has been our form.
- A model, which is simply the entity with which we are going to work to create a record based on the information or data received by the form.
Build the app
In this block we are going to go step by step building the application that we indicated previously.
Defining the Model
Let's work with the movies model:
<?php namespace App\Models;
use CodeIgniter\Model;
class PersonModel extends Model {
protected $table = 'people';
protected $primaryKey = 'id';
protected $allowedFields = ['name', 'surname','age','description'];
}
Define controller
Now, we are going to create a controller that we are going to call as Person.php:
class Person extends Controller
{
}
Remember that the controller is the layer that is in charge of communicating the view with the model, and more than this, it is the one that has the logic and organization to carry out each of the processes indicated above, painting a view and processing a form.
Define CRUD resource routes
Como vamos a aprovechar este formulario para crear un completo CRUD en posteriores entradas, vamos a aprovechar a definir una ruta de tipo recurso, como la explicamos anteriormente:
$routes->resource('person');
With this, we now have to define in our controller some functions with corresponding names and parameters that are going to be in charge of carrying out our CRUD, although for now we are interested in simply creating a record; for that.
Function to paint a form get
The first function that we are going to see would be to paint a form, therefore we do not have to do anything else, remember that we are creating a route of resource type and therefore we have to create a function called new, which is what allows us to consumed via a get request and will simply return a view:
public function new()
{
return view('person/new');
}
Function to create a Post record
Now the most interesting function, the one that can only be consumed via a Post request and therefore is the one that receives the data from our form that travels based on a Post request:
public function create()
{
$person = new PersonModel();
$person->insert(
[
'name' => $this->request->getPost('name'),
'surname' => $this->request->getPost('surname'),
'age' => $this->request->getPost('age'),
'description' => $this->request->getPost('description'),
]
);
}
The request to get the data
Let's remember that regardless of what you use, there is always something called request, which is the request of our user, here the data of our user travels, the data of the previous form, therefore, it is the mechanism that we have to use to obtain the data; in C4, to obtain data that goes via Post would be as follows:
this->request->getPost('key')
Where a key matches the name of the field; so to get each of the above form fields; for example, name:
$this->request->getPost('name')
And now we simply want to insert a record: for that we have to create an instance of our model, which in this case would be of some people, and the function called insert, which is the one that is in charge of inserting a record; It's that simple, and with this we have the complete process.
View for the form
Let's create a simple form in the app/Views folder of our application like the following and call it new.php to match the function name:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Crear Persona</title>
</head>
<body>
<h1>Crear Persona</h1>
<form action="<?= base_url() ?>/person" method="post">
<label for="name">Nombre</label>
<input type="text" name="name" id="name" value="Andres">
<br>
<label for="surname">Apellido</label>
<input type="text" name="surname" id="surname" value="Cruz">
<br>
<label for="age">Edad</label>
<input type="text" name="age" id="age" value="29">
<br>
<label for="description">Descripción</label>
<textarea name="description" id="description" cols="30" rows="10">Desarrollador</textarea>
<br>
<input type="submit" value="Enviar">
</form>
</body>
</html>
Return to form view
Now at this point, if you try to create a record from: http://codeigniter4.test/person/new or the domain you have configured, you will see that at the end a blank screen remains, and this is because the create function returns nothing; here you could perfectly do something like the following to paint a view from create:
public function create()
{
*** Resto del código
return view('person/new');
}
But in this way we are basically doing the same job as the new function and therefore we know that replicating code so that it stays the same is bad in any programming language and besides that, if at this point we try to refresh the page we will see an inside alert like the following:
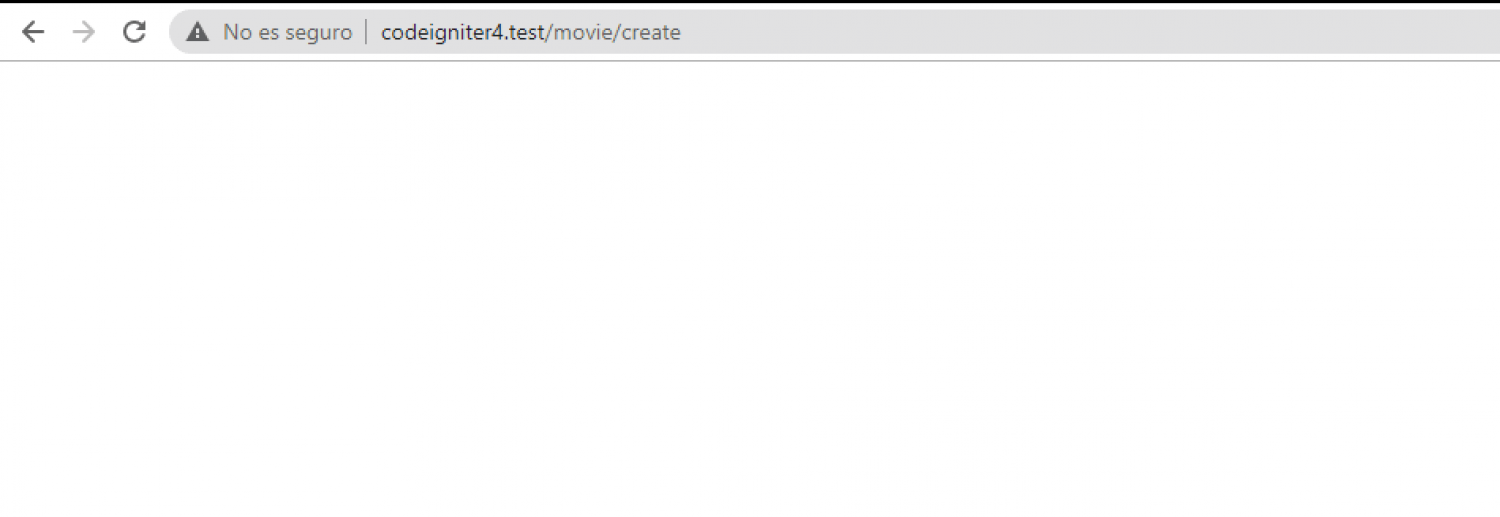
And this is because we are sending or again a Post type request; and it doesn't look good; therefore, what we are going to do is simply return to the previous function, for this we can use the routes and we have a function called back that allows us to return to the previous function:
return redirect()->back();
It is as if you press back or go back from your browser
And with this now it works in a much better way.
Extra: Remember previous values with the old function in CodeIgniter 4
You are probably interested that C4 remembers the previous values in your form; for that, we can use a function called old that receives two parameters:
- Field Name
- Default value (which is the one we are going to establish when there is no previous value, for example, when we enter the new page for the first time.)
Now, so that the data can be taken from the redirection that we are doing, we have to use the function called withInput when doing the redirection:
return redirect()->back()->withInput();
And for the form fields, we have to define the function in question; for example:
<?=old('name',"")?>
I agree to receive announcements of interest about this Blog.
We are going to show the process to send forms in CodeIgniter 4, from the creation of routes, controller, functions and of course, the process of the request or request of our user.
- Andrés Cruz