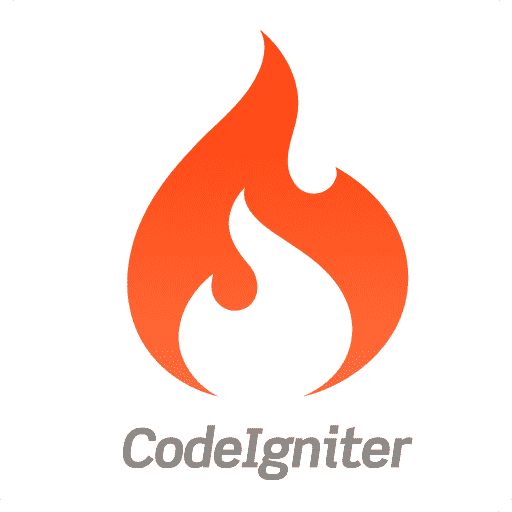
Performing validations on the data received by the user through a form is essential to guarantee the integrity and quality of the requested data; this is why form validations are used, indicating which fields are mandatory and what structure it must have; validations are particularly important in situations that depend on this data, since errors can be costly or even dangerous, since unvalidated forms can be used to perform SQL injection processes, among other types of attacks.
Validating forms in CodeIgniter is very easy; we just have to know what we want to validate; what are the rules that we are going to incorporate; for example, if we have the following form:
<div class="form-group">
<label>Nombre</label>
<input type="text" value="<?php echo $name ?>" class="form-control" placeholder="Nombre" name="name" required>
<?php echo form_error('name', '<div class="text-danger">', '</div>') ?>
</div>
<div class="form-group has-feedback">
<label>Apellido</label>
<input type="text" value="<?php echo $surname ?>" class="form-control" placeholder="Apellido" name="surname" required>
<?php echo form_error('surname', '<div class="text-danger">', '</div>') ?>
</div>
<div class="form-group has-feedback">
<label>Teléfono</label>
<input type="text" value="<?php echo $phone1 ?>" class="form-control" placeholder="Teléfono" name="phone1" required>
<?php echo form_error('phone1', '<div class="text-danger">', '</div>') ?>
</div>
<div class="form-group has-feedback">
<label>Teléfono 2</label>
<input type="text" value="<?php echo $phone2 ?>" class="form-control" placeholder="Teléfono 2" name="phone2" required>
<?php echo form_error('phone2', '<div class="text-danger">', '</div>') ?>
</div>
We are interested in all the fields being present except for phone 2 which is completely optional, we are interested in placing various maximum and minimum lengths in our form and we are also interested in some fields such as age only allowing integer data types; We can do all this and much more in CodeIgniter through form validation; among the most important we have:
Regla | Parámetros | Descripción | Ejemplo |
---|---|---|---|
required | No | Returns true or false if the element is empty. | |
min_length | Yes | Returns FALSE if the form element has a shorter length than the one defined in the parameter. | min_length[3] |
max_length | Yes | Returns FALSE if the form element has a length longer than the one defined in the parameter. | max_length[12] |
exact_length | Yes | Returns FALSE if the form element has a length other than the length defined in the parameter. | exact_length[8] |
valid_email | No | Returns FALSE if the form element does not have a valid email. | |
numeric | No | Returns FALSE if the form element contains something other than a number. | |
integer | No | Returns FALSE if the form element is not an integer |
As you can see, we have validation rules for many cases, data types, emails, decimals, required, and maximum and minimum amounts for String lengths; these are the ones I consider most important, but you can have all the validation rules from the official documentation.
So, to place validation rules in the previous form we must do the following:
$this->form_validation->set_rules('name', 'Nombre', 'max_length[100]|required');
$this->form_validation->set_rules('surname', 'Apellido', 'max_length[100]|required');
$this->form_validation->set_rules('phone1', 'Número de celular', 'min_length[9]|max_length[9]|required');
$this->form_validation->set_rules('phone2', 'Número de celular', 'min_length[9]|max_length[9]);
As you can see, we added multiple validation rules separated by pipes (|); in addition to this, note that phone 2 does not have the "required" validation type applied to it because it is completely optional; then when sending the POST (it can also be GET, just change POST to GET):
if ($this->form_validation->run()) {
// datos a guardar
$save = array(
'name' => $this->input->post("name"),
'surname' => $this->input->post("surname")
);
}
Here simply when we detect that the form is a Post and it is valid, we simply compose an array to later save it in the database; but you can do what you want with the validated data.
Custom rules for the form in CodeIgniter
You may want to create your own custom validation rules for CodeIgniter; for that you simply have to create a function in your controller class and evaluate its condition; the important thing is that it returns either true or false:
function validate_passwd($password) {
// At least one digit required
// $regex = '(?=.*\d)';
// At least one lower case letter required
$regex = '(?=.*[a-z])';
// At least one upper case letter required
// $regex .= '(?=.*[A-Z])';
// No space, tab, or other whitespace chars allowed
$regex .= '(?!.*\s)';
// No backslash, apostrophe or quote chars are allowed
$regex .= '(?!.*[\\\\\'"])';
if (preg_match('/^' . $regex . '.*$/', $password)) {
return TRUE;
}
return FALSE;
}
And then you add it to your validation rules by placing the function created above with the callback_ prefix:
$crud->set_rules('passwd', 'Contraseña', 'min_length[8]|required|max_length[12]|callback_validate_passwd_admin');
Then go to your language file located at application\language\english\form_validation_lang.php and set the error message for your new rule:
$lang['validate_same_passwd'] = "La contraseña debe ser igual a la almacenada en el sistema.";
Here we are taking as an example that you are using English as the default language in your project, but if you change the language you simply change the folder.
Custom messages for validation rules:
In the previous file we can define the messages in case we want to customize them; but we can also do it from the controller:
$this->form_validation->set_message('min_length', '{field} must have at least {param} characters.');
Show form errors
Finally, to show the errors of the form we can use:
<?php echo form_error(name, '<div class="text-danger">', '</div>') ?>
This element is usually placed in the form view, next to the form element to which we apply the validation rule, which for this example is one called name.
Show all errors in a form
Many times it is useful to show the errors together, all the errors of the form from a single place from a single function, for that there is the following function:
validation_errors()
You can also define the element that will contain said error, which by default is simply a line of text, you can place it in a Bootstrap alert for example:
validation_errors('<div class="alert alert-danger" role="alert">', '</div>')
Complete example of a form element in Bootstrap 5:
<div class="form-group">
<?php
echo form_label('Título', 'name');
?>
<?php
$text_input = array(
'name' => 'name',
'minlength' => 10,
'maxlength' => 100,
'required' => 'required',
'id' => 'name',
'value' => $name,
'class' => 'form-control input-lg',
);
echo form_input($text_input);
?>
<?php echo form_error('name', '<div class="text-danger">', '</div>') ?>
</div>
I agree to receive announcements of interest about this Blog.
We are going to learn how to validate forms in CodeIgniter, create custom rules, messages in translation files, in the controller and display form errors.
- Andrés Cruz