Validations in CodeIgniter 4 are a fundamental point in any application and CodeIgniter 4 is no exception; to be able to validate our data in order to be sure that it is valid and secure; they are processes that we have to carry out in our applications; but surely there are validations that are a little more specific for your business and therefore you cannot use the generic ones that the framework offers us; in those cases, we have to create one ourselves; in CodeIgniter 4 we can do it easily and with them we avoid dirtying the controller too much, which is where we usually place them; we have to go to the file of:
config/Validation.php
En ella, veremos definidas una serie de clases:
public $ruleSets = [
\CodeIgniter\Validation\Rules::class,
\CodeIgniter\Validation\FormatRules::class,
\CodeIgniter\Validation\FileRules::class,
\CodeIgniter\Validation\CreditCardRules::class,
];
Which you can review and you will see that they are classes with functions, the names of the functions correspond to the validations that we can use and they receive an argument, which as you can suppose is the validation parameter; The framework already takes care of all this mapping for us; in my example (which is part of my complete course on CodeIgniter 4 that you can take on this platform) we are going to create a file inside:
app/validator/UserRules
We are going to create an extra folder that we call /app/Validation (it can have any name and inside it a file called UserRules (or whatever name you prefer):
<?php
namespace App\Validation;
use App\Models\UserModel;
class UserRules
{
}
And within this file and classes, we create our validation functions to which we apply our validations; logically, they have to return a boolean, true if validation passes and false otherwise:
public function provider(string $userId): bool
{
return $this->checkType($userId, "provider");
}
public function customer(string $userId): bool
{
return $this->checkType($userId, "customer");
}
To use them, just:
$res = $this->validate([
'user_id' => 'required|customer'
]);
Extra, create validations with parameter passing
We can also create more general validations in which we can pass one or more parameters by defining the validation:
$res = $this->validate([
'user_id' => 'required|checkType[customer]',
]);
And in our class:
public function checkType($userId, $type)
{
$userModel = new UserModel();
$user = $userModel->asObject()->where("type", $type)->find($userId);
return $user != null;
}
Conclusions
So now you know how you can use custom validations and a bit more than that, pass parameters to custom validations.
- Andrés Cruz
En español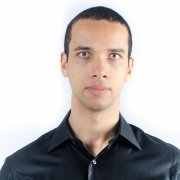
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter