If conditionals
The if() conditional is very similar to that used in any other programming language such as Java, PHP, JavaScript, etc.
But it has an interesting addition that allows us to indicate if the last expression of a conditional is a value, then we can assign that value to some variable; that is to say:
var x = 5
val h = if (x > 0) {
// otras operaciones
true
} else {
// otras operaciones
false
}
Return values/variables in the conditional
In this small example, we compare the variable x to the value returned by the conditional, which can be true or false depending on the value that the variable x has at a given moment (for the example h would have the value true); to use this structure we must leave the value that we want to return (in this case a boolean) at the end of the conditionals (the if and the else, of course, the conditional can implement any other logic or series of operations, but the return values -the ones we want to set to the assigned variable- must be set at the end of the conditional).
This is an interesting functionality that Kotlin has that allows us to reduce the number of lines of code when we want to express a hierarchy of conditionals, the redundancy in the assignment to final variables and allows us to have more concise and readable code that translates into a better user experience. time to develop our applications, and of course, we can practically use this scheme with any other structure.
Check if a variable is null: autocast
A very important point is that apart from the implementations that we saw previously to use the properties of variables of type NULLable or that can be null in Kotlin through a conditional:
val str: String? ="Kotlin"
if (str != NULL) {
println(str.length)
}
Let's remember that if a variable is declared as NULLable, it means that it can be null at some point and therefore we must use the operators that we used in a previous entry to avoid null references, but when performing the previous verification, we can skip this step of use the operators, since the Kotlin compiler autocasts the NULLable variable that we are checking and in this way we can use any property without the need for these operators.
Another expression that we can use for when we have many conditionals is the when expression, which is the equivalent of the switch expression of other programming languages but also enhanced:
We can express values by range:
when (x) {
in 1..9 -> print("x es un número entero positivo de una cifra")
else -> print("x no es un número entero positivo de una cifra")
}
We can use functions to indicate the data type of the variable:
when (x) {
is String -> print("x es un texto")
else -> print("ninguno de los anteriores")
}
Use own functions or some API:
fun mas_cinco(x: Int): Int {
return x+5
}
when (x) {
in 1..9 -> print("x es un número entero de una cifra")
mas_cinco(x) -> print("x es válido")
!in 10..20 -> print("x está fuera de rango")
else -> print("ninguno de los anteriores")
}
Indicate that a number is even:
when (x){
x.isEven() -> print("x es par")
else -> print("x es impar")
}
Or indicate that it is odd, negating the previous function:
when (x){
!x.isEven() -> print("x es par")
else -> print("x es impar")
}
Or using the function to indicate that the number is odd:
when (x){
x.isOdd() -> print("x es impar")
else -> print("x es par")
}
These last examples we are using extension functions to define isOdd() and isEven() which will be the subject of another entry.
Also as you can see in the above code, we can use the else keyword which works the same as the default in other programming languages.
We can decide not to express the value in the when clause and instead express it in each of the cases.
when {
x.isOdd() -> print("x is odd")
x.isEven() -> print("x is even")
else -> print("x is funny")
}
Perform mathematical operations:
when(x) {
10 + 20 -> print("30")
else -> print("Otro valor")
}
That of course these values can be variables.
We can also use the defined when block to return a value and assign it to a variable, just like we did with the if above:
val r = when(x) {
10 + 20 -> "30"
else -> "Otro valor"
}
We can combine all these options that we saw previously as we want, the idea is to present some of the possible scenarios to have a clearer idea of the operation of this powerful conditional that we can use in Kotlin.
In this post we saw how to use conditionals in Kotlin, in the next post we will talk about cycles or loops in Kotlin; you can refer to the official documentation at the following link: Kotlin: Control Flow: if, when, for, while.
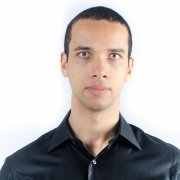
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter