Buttons, like any user interface, consist of an element that allows a user to interact with the system; they are generally event-based and highly customizable; in this article we will see how to define and use a button in Android.
In Android, the buttons can consist of text and/or image, classifying it into three types of buttons:
Text: To create a button with only text, just use the following XML:
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Boton" />
When interpreting the above XML:

Image: To create a button with an image, just use the following XML:
<ImageButton
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/ic_action_new" />
When interpreting the above XML:

Text and Image: To create a button made up of text and images, just use the following XML to define the button:
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:drawableLeft="@drawable/ic_action_new"
android:text="Boton" />
When interpreting the above XML:

If we want the image to be aligned to the right:
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:drawableRight="@drawable/ic_action_new"
android:text="Boton" />
When interpreting the above XML:

Button attributes
Some of the main button attributes are:
- android:id Identifier of the element; in this case of the button; as with HTML, it is the way to uniquely identify and access an element.
- android:text Text of the element; in this case of the button.
- drawable(Left/Right) With this attribute we can place an image to the button aligned to the left (drawableLeft) or to the right (drawableRight).
- layout_width and layout_height We indicate the width and height respectively of the element; in this case our button; can receive any of the following values:
- wrap_content Indicates that the dimensions of the element will be adjusted to the content that it presents:
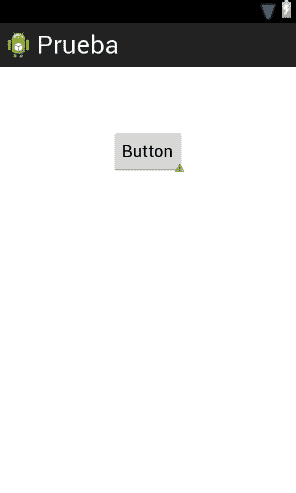
android:layout_width="match_content" y android:layout_height="wrap_content".
- match_parent Indicates that the element's dimensions will be matched to the container's means; It's like the width or height property with the value 100%:
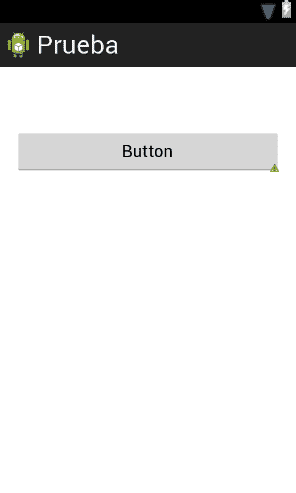
android:layout_width="wrap_content" y android:layout_height="wrap_content".
A numerical value, generally in densities per pixels (dp) or dpi, but others such as and in pixels (px) can also be used, but the latter is not highly recommended; example 50 dp.
Of course, other style attributes can also be applied to them, such as:
- android:visibility Allows you to define if the element will be visible or not; same as the visibility property in CSS.
- android:padding Allows you to define the space or margin between the element and its content; like the padding property in CSS.
- android:background Allows you to indicate the background color or image of an element; same as the background property in CSS.
- android:layout_gravity Align an element; they behave similarly to the float property in CSS.
- android:layout_margin Allows you to define the space or margin between the element and its container; like the margin property in CSS.
- Among many others.
Button events
We can use the attribute:
android:onClick="funcion"
For example, if we wanted to apply this functionality to the first type of button seen (although it is possible to use it in all three types of buttons):
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Alarm"/>
android:onClick="funcion"
Where "function" is the method or function that will be called in the Activity when the button is clicked.
public void funcion(View view) {
// hacemos algo ...
}
OnClickListener event handler
We can also program the event handler outside of the XML that forms the interface and place it inside the Activity:
Button buttonButton = (Button) findViewById(R.id.buttonButton); buttonButton.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { // do something ... } });
The R class contains the references to the components of our application; basically graphics, elements and global texts of the application; with R.id.buttonButton we can access the element with id buttonButton:
android:id="@+id/botonButton"
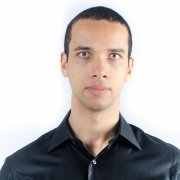
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter