As part of our list application, which is part of our CRUD, we are going to create the delete option, which since it is not necessary to define a component for this option, since we do it directly in the list, we can use anything we can apply a click event, for example, to a button:
<template>
<div>
<h1>Listado</h1>
<router-link :to="{ name: 'create' }">Crear</router-link>
<ul>
<li v-for="(a, k) in $root.animes" :key="a">
<router-link
:to="{ name: 'detail', params: { anime: JSON.stringify(a) } }"
>
{{ a.title }}
</router-link>
-
<router-link :to="{ name: 'edit', params: { position: k } }"
>Edit</router-link
>
<button @click="remove(k)">Eliminar</button>
</li>
</ul>
</div>
</template>
As you can see, we have a remove function, to which we pass the index, but it can also be the id if you are working with a database or a rest api; finally we removed it from our list:
<script>
export default {
data() {
return {
animes: [],
};
},
async mounted() {
await this.$root.getAnime();
console.log(this.$root.animes[1].title);
},
methods: {
remove:function(i){
this.$root.animes.splice(i,1)
}
},
};
</script>
And with this, when the element of a reactive component, such as the array, is removed, it is automatically updated from our array.
- Andrés Cruz
En español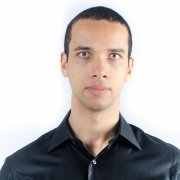
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter