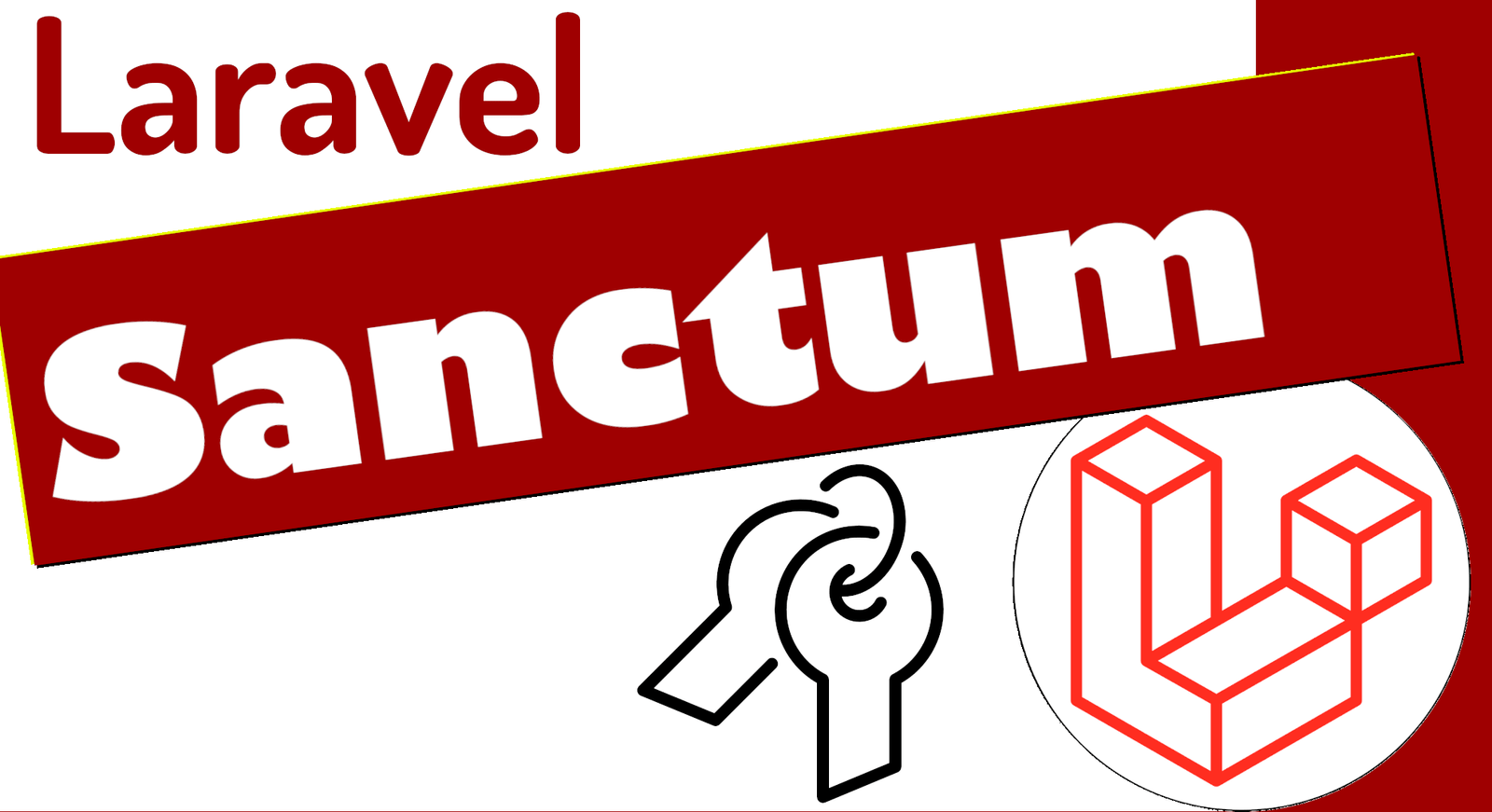
In the previous post we saw how to handle creating the access token in Laravel Sanctum; in this post, we will see how to handle the authentication token in Laravel Sanctum using Vue in a cookie; specifically, the token generated by Laravel Sanctum through login, register it in a cookie and consume it when starting the application in Vue.
Let's start from a project created in Laravel in which we configure Vue in a Laravel project.
Install cookie plugin
We are going to install a plugin to handle Cookies in Vue 3:
https://www.npmjs.com/package/vue3-cookies
Ejecutamos en la terminal:
$ npm install vue3-cookies --save
Configure the plugin in the project
Its use is very simple, we are going to first initialize the cookie plugin in the project, for this, we will use the application configuration file:
import { createApp } from "vue";
//tailwind
import '../../css/vue.css'
// Oruga
import Oruga from '@oruga-ui/oruga-next'
import '@oruga-ui/oruga-next/dist/oruga.css'
import '@oruga-ui/oruga-next/dist/oruga-full.css'
import '@oruga-ui/oruga-next/dist/oruga-full-vars.css'
//Material Design
import "@mdi/font/css/materialdesignicons.min.css"
import axios from 'axios'
import App from "./App.vue"
import router from "./router"
import VueCookies from 'vue3-cookies'
const app = createApp(App).use(Oruga).use(router)
app.use(VueCookies);
app.config.globalProperties.$axios = axios
window.axios = axios
app.mount("#app")
In the script above, we have other plugins configured, in this case, Oruga UI with Laravel and Vue, as this is a project that is part of my complete course on Laravel.
Use of cookies
With this, we can make use of the cookie and with this, be able to save values:
this.$cookies.set(keyName,'value');
Or get them:
$cookies.get(keyName)
As you can see, its use once configured is extremely simple.
Configure vue3-cookies with the authentication data
We are going to create a function to set the user values in the parent component, with which we can use it anywhere in the application through the child components:
resources\js\vue\App.vue
setCookieAuth(data) {
this.$cookies.set("auth", data);
},
The same, we will use it from the login:
resources\js\vue\componets\Auth\Login.vue
submit() {
this.cleanErrorsForm();
return this.$axios
.post("/api/user/login", this.form)
.then((res) => {
this.$root.setCookieAuth({
isLoggedIn: res.data.isLoggedIn,
token: res.data.token,
user: res.data.user,
});
***
}
The above function is the one we use to configure the authentication cookie in the project when logging in from the application; The login function in Laravel is:
public function login(Request $request)
{
$credentials = [
'email' => $request->email,
'password' => $request->password
];
if (Auth::attempt($credentials)) {
$token = Auth::user()->createToken('myapptoken')->plainTextToken;
session()->put('token', $token);
return response()->json([
'isLoggedIn' => true,
'user' => auth()->user(),
'token' => $token,
]);
}
return response()->json("Usuario y/o contraseña inválido", 422);
}
I agree to receive announcements of interest about this Blog.
We are going to implement a login method in Laravel to generate an authentication token in Sanctum that we consume through Vue and record in a Cookie.
- Andrés Cruz