Create a list in Vue 3 with the o-table component Oruga UI, Rest Api in Laravel
We are going to build the necessary template to create a list of elements, in this case post:
<template>
<div>
<h1>Listado de Post</h1>
<o-table
:loading="isLoading"
:data="posts.current_page && posts.data.length == 0 ? [] : posts.data"
>
<o-table-column field="id" label="ID" numeric v-slot="p">
{{ p.row.id }}
</o-table-column>
<o-table-column field="title" label="Título" v-slot="p">
{{ p.row.title }}
</o-table-column>
<o-table-column field="posted" label="Posteado" v-slot="p">
{{ p.row.posted }}
</o-table-column>
<o-table-column field="created_at" label="Fecha" v-slot="p">
{{ p.row.created_at }}
</o-table-column>
<o-table-column field="category" label="Categoría" v-slot="p">
{{ p.row.category.title }}
</o-table-column>
</o-table>
</div>
</template>
As you can see in the code above, we just put in posts as one more parameter, there's no need to use a v-for to iterate through each element, otherwise we use an o-table-column component to create each one of the items of our element.
We don't reference the item directly, otherwise we first map to the v-slot and then access the item along with additional row data.
Get the data
To get the data for the previous list, it is typical, a property called posts, to manage the posts, and then an axios request when launching the application:
<script>
export default {
data() {
return {
posts: [],
isLoading: true,
};
},
async mounted() {
this.$axios.get("/api/post?page="+this.currentPage).then((res) => {
this.posts = res.data;
console.log(this.posts);
this.isLoading = false;
});
},
};
</script>
We have an additional property to know if the table data is being loaded or not.
- Andrés Cruz
En español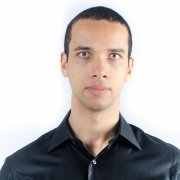
Develop with Laravel, Django, Flask, CodeIgniter, HTML5, CSS3, MySQL, JavaScript, Vue, Android, iOS, Flutter